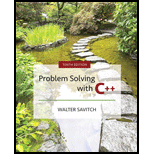
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 14, Problem 4P
Explanation of Solution
Recursive function for “handshake” function:
The recursive function definition for “handshake” function is shown below:
//Function definition for "handshake" function
int handshake(int n)
{
/* If "n" is equal to "1", then */
if(n == 1)
//Returns "0"
return 0;
/* If "n" is equal to "2", then */
else if(n == 2)
//Returns "0"
return 1;
//Otherwise
else
/* Recursively call the function "handshake" with subtracting the value of "n" with "1" */
return n - 1 + handshake(n - 1);
}
Explanation:
The above function is used to compute the number of handshakes in a room using recursive function.
- In this function, If the value of “n” is equal to “1”, then returns “0”.
- If the value of “n” is equal to “2”, then returns “1”.
- Otherwise, that is if the value of “n” is greater than “2”, then returns the value by “n - 1 + handshake(n - 1)”...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a recursive function that takes as a parameter a nonnegative integer and generates the following pattern of stars. If the nonnegative integer is 4, the pattern generated is as follows: **** *** ** * * ** *** **** Also, write a program that prompts the user to enter the number of lines in the pattern and uses the recursive function to generate the pattern. For example, specifying 4 as the number of lines generates the preceding pattern.
Write a recursive function called draw_triangle() that outputs lines of '*' to form a right side up isosceles triangle. Function draw_triangle() has one parameter, an integer representing the base length of the triangle. Assume the base length is always odd and less than 20. Output 9 spaces before the first '*' on the first line for correct formatting.
Hint: The number of '*' increases by 2 for every line drawn.
Ex: If the input of the program is:
3
the function draw_triangle() outputs:
* ***
Ex: If the input of the program is:
19
the function draw_triangle() outputs:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
Note: No space is output before the first '*' on the last line when the base length is 19.
if __name__ == '__main__': base_length = int(input()) draw_triangle(base_length)
8. A country has coins of denomination 3, 5 and 10 respectively. Write a recursive function
canchange() which returns -1 if it is not possible to pay a value of k using these coins.
Otherwise, it returns the minimum number of coins needed to make the payment. For example,
canchange(7) will return -1. On the other hand, canchange(14) will return 4 because 14 can be
paid as 3+3+3+5 and there is no other way to pay with fewer coins
Chapter 14 Solutions
Problem Solving with C++ (10th Edition)
Ch. 14.1 - Prob. 1STECh. 14.1 - Prob. 2STECh. 14.1 - Prob. 3STECh. 14.1 - Prob. 4STECh. 14.1 - Prob. 5STECh. 14.1 - If your program produces an error message that...Ch. 14.1 - Write an iterative version of the function cheers...Ch. 14.1 - Write an iterative version of the function defined...Ch. 14.1 - Prob. 9STECh. 14.1 - Trace the recursive solution you made to Self-Test...
Ch. 14.1 - Trace the recursive solution you made to Self-Test...Ch. 14.2 - What is the output of the following program?...Ch. 14.2 - Prob. 13STECh. 14.2 - Redefine the function power so that it also works...Ch. 14.3 - Prob. 15STECh. 14.3 - Write an iterative version of the one-argument...Ch. 14 - Prob. 1PCh. 14 - Prob. 2PCh. 14 - Write a recursive version of the search function...Ch. 14 - Prob. 4PCh. 14 - Prob. 5PCh. 14 - The formula for computing the number of ways of...Ch. 14 - Write a recursive function that has an argument...Ch. 14 - Prob. 3PPCh. 14 - Prob. 4PPCh. 14 - Prob. 5PPCh. 14 - The game of Jump It consists of a board with n...Ch. 14 - Prob. 7PPCh. 14 - Prob. 8PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 8. A country has coins of denomination 3, 5 and 10 respectively. Write a recursive function canchange() which returns -1 if it is not possible to pay a value of k using these coins. Otherwise, it returns the minimum number of coins needed to make the payment. For example, canchange(7) will return -1. On the other hand, canchange(14) will return 4 because 14 can be paid as 3+3+3+5 and there is no other way to pay with fewer coins Programming Language:- Carrow_forwardNo plagarism! Correct and detailed answer ( code with comments and output screenshot if necessary)arrow_forwardFibonacci numbers are a sequence of integers, starting with 1, where the value of each number is the sum of the two previous numbers, e.g. 1, 1, 2, 3, 5, 8, etc. Write a function called fibonacci that takes a parameter, n, which contains an integer value, and have it return the nth Fibonacci number. (There are two ways to do this: one with recursion, and one without.)arrow_forward
- Question 2: Implementing a Recursive Function .Write recursive function, recursionprob(n), which takes a positive number as its argument and returns the output as shown below. The solution should clearly write the steps as shown in an example in slide number 59 and slide number 60 in lecture slides. After writing the steps, trace the function for “recursiveprob(5)” as shown in an example slide number 61. Function Output: >> recursionprob(1) 1 >> recursionprob(2) 1 4 >> recursionprob(3) 1 4 9 >>recrusionprob(4) 1 4 9 16arrow_forwardWrite a recursive void function that has one parameter that is a positive integer. When called, the function writes its argument to the screen backward. That is,, if the argument is 1234, it outputs the following to the screen: 4321. I've literally tried to create this program and I have no idea how to make it work. Please help me. Is it possible to explain line by line what the code does?arrow_forwardin c++. using recursive function.arrow_forward
- 2√2 π + 2 22 -+ 1 1 1 + 2 22 22 V2 2 1 + 2 22 22 2 V2 This equation can be used in a recursive function to compute to a large number of decimal places. In the above 1 11 + || equation, consider the base case as functions to compute the value of accurate recurse so that you can get the π of accuracy Write a Python code (and show its output) using recursive up to 8 decimal places as 3.14159265. How many times do you need to up to 8 decimal places? Upload a .py file of your source code. Include your answer to the follow-up question in a comment plock.arrow_forwardThe 4th problem mimics the situation where eagles flying in the sky can be spotted and counted.FindEagles: a recursive function that examines and counts the number of objects (eagles) in aphotograph. The data is in a two-dimensional grid of cells, each of which may be empty (value 0) orfilled (value 1 to 9). Maximum grid size is 50 x 50. The filled cells that are connected form an object(eagle). Two cells are connected if they are vertically, horizontally, or diagonally adjacent. Thefollowing figure shows 3 x 4 grids with 3 eagles. 0 0 1 21 0 0 01 0 3 1 FindEagle function takes as parameters the 2-D array and the x-y coordinates of a cell that is a part ofan eagle (non-zero value) and erases (change to 0) the image of an eagle. The function FindEagleshould return an integer value that counts how many cells has been counted as part of an eagle and havebeen erased. The following sample data has two pictures, the first one is 3 x 4, and the second one is 5 x 5 grids. Notethat your program…arrow_forwardPlease can be handwritten. Question 2: Implementing a Recursive Function . Write recursive function, recursionprob(n), which takes a positive number as its argument and returns the output as shown below. The solution should clearly write the steps as shown in an example in slide number 59 and slide number 60 in lecture slides. After writing the steps, trace the function for “recursiveprob(5)” as shown in an example slide number 61. Function Output: >> recursionprob(1) 1 >> recursionprob(2) 1 4 >> recursionprob(3) 1 4 9 >>recrusionprob(4) 1 4 9 16arrow_forward
- Write a recursive function called digit_count() that takes a positive integer as a parameter and returns the number of digits in the integer. Hint: The number of digits increases by 1 whenever the input number is divided by 10. Ex: If the input is: 345 the function digit_count() returns and the program outputs: 3 # TODO: Write recursive digit_count() function here. if __name__ == '__main__': num = int(input()) digit = digit_count(num) print(digit)arrow_forwardThis is in Python This has two parts. This is a problem on recursion. Part a: Write a recursive function that accepts an integer argument, n. The user needs to be asked for the number n. The function should display n lines of asterisks on the screen, with the first (the top) showing 1 asterisk, the second from the top showing two asterisks, up to the nth line which shows n asterisks. Part b. Write a recursive function that accepts an integer argument, n. The user needs to be asked for the number n. The function should display n lines of asterisks on the screen, with the first (the top) showing n asterisks, the second from the top showing n-1 asterisks, up to the nth line which shows 1 asterisk. Submit the two files and two sample outputs for each of the parts.arrow_forwardMULTIPLE FUNCTIONS AND RECURSIVE FUNCTIONS Use #include<stdio.h> Implement the picture shown below, Implement the two output shown below. Thank you..arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
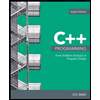
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning