Predict the Output
7. What is the output of the following programs?
A) #include <iostream>
using namespace std;
int function(int);
int main()
{
int x = 10;
cout << function(x) << endl;
return 0;
}
int function(int num)
{
if (num <= 0)
return 0;
else
return function(num - 1) + num;
}
B) #include <iostream>
using namespace std;
void function(int);
int main()
{
int x = 10;
function(x);
return 0;
}
void function(int num)
{
if (num > 0)
{
for (int x = 0; x < num; x++)
cout << '*';
cout<< endl;
function(num - 1);
}
}
C) #include <cstdlib>
#include <string>
#include <iostream>
using namespace std:
void function(string str, int pos);
int main(int argc, char** argv)
{
string names = "Adam and Eve";
function(names, 0);
return 0;
}
void function (string str. int pos)
{
if (pos < str. length())
{
function(str, pos+1);
cout << str[pos]:
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Artificial Intelligence: A Modern Approach
C Programming Language
Concepts of Programming Languages (11th Edition)
Database Concepts (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Why do you need to include function prototypes in a program that contains user-defined functions? (5)arrow_forwardExplanation: Default arguments should always be declared at the rightmost side of the parameter list but the above function has a normal variable at the rightmost side which is a syntax error, therefore the function gives an error. 1. #include int fun(int=0, int = 0); int main() { cout << fun(5); return 0; } int fun(int x, int y) { return (x+y); } а) -5 b) 0 c) 10 d) 5 2. int main() { int a=10; int b,c; b =a++; c = a; cout<arrow_forwardC++arrow_forwardC++ pleasearrow_forwardQues tion 1 The following program converts and print the Fahrenheit equivalents of all Celsius temperatures from 0 to 100 degrees, and the Celsius equivalents of all Fahrenheit temperatures from 32 to 212 degrees. Implement the integer functions as declared in the program: #include int celcius( int fTemp ); // function prototype int fahrenheit( int eTemp ); // function prototype int main() { int i, / loop counter Il display table of Fahrenheit equivalents of Celsius temperature printf "Fahrenheit equivalents of Celcius temperatures:In"); printf( "Celciust\tFahrenhe itln"); II display Fahrenheit equivalents of Celsius 0 to 100 for( i = 0; i<= 100; i+H) { printft "%d\t\W%d\n", i, fahrenheit( i ) ); II display table of Celsius equivalents of Fahrenheit temperature printf( "\nCelcius equivalents of Fahrenheit temperatures:\n"); printf( "FahrenheituCelcius\n"); Il display Celsius equivalents of Fahrenheit 32 to 212 for( i = 32; i<= 212; i+) { printf "%d\t\w%d\n", i, celcius( i ) ); return…arrow_forwardC programing Given the function below, what would the function call question3(10, 101) return? int question3(int a, int b) {if (a == 0) return b;if (b == 0) return a;return question3(10*a+b%10, b/10);}arrow_forwardC++arrow_forwardClasswork Requirements: Developa program in C++ that: Reads as many test scores as the user wants from the keyboard (assuming at most 50scores). Test scores should be whole numbers. You must validate user input; only positive numbers are valid. Prints the scores in original order sorted from high to low the highest score the lowest score the average of the scores Implement the following functions using the given function prototypes: void displayArray(int array[], int size)- Displays the content of the array void selectionSort(int array[], int size)- sorts the array using the selection sort algorithm in descending Hint: refer to example 8-5 in the textbook. int findMax(int array[], int size)- finds and returns the highest element of the array int findMin(int array[], int size)- finds and returns the lowest element of the array double findAvg(int array[], int size)- finds and returns the average of the elements of the arrayarrow_forwardC++arrow_forwarddroparrow_forward3. (a) A PHP function is defined as follows: function planets ($arr) { if ($arr [1]) { print "Mercury"; } else { for ($i=0; $i <= $arr [2]; $i++) {print $arr [3] [$i]; } } print count ($arr); } Recall that array $arr can be defined using a statement of the form: $arr = array (...); (i) Give an array $arr such that planets ($arr) prints Mercury For this case, what is the output of the instruction print count ($arr); Give an array $arr such that planets ($arr) prints Venus (ii)arrow_forwardTrue or False : The concept of function abstraction hinders our code development by confusing us with the details of the function.arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
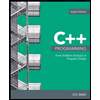