Concept explainers
Consider two parallel arrays of the same size, one containing strings and the second containing integers. Write C++ statements to output the information in the two arrays as a table of names and numbers. The first column of the table will contain the names left-justified in a field of 20, and the second column will contain the integers right-justified in a field of 10. Here is an example of the data when the size of the array is 2.
const int SIZE = 2;
string names[SIZE] = {"Catherine", "Bill"};
int numbers[SIZE] = {12, 2005};

Trending nowThis is a popular solution!

Chapter 13 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Absolute Java (6th Edition)
Starting Out With Visual Basic (8th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- c++ 6. Write a program which will prompt the user to enter an array of 12 characters. The program should then print the array, and then print the array again, but this time as a 4 colums by 3 rows array.arrow_forwardusing C++ In this exercise you would be using a text input file to store a number of students first and last names, and each students scores in test1, test2, midterm, final and homeworks. Now, you get all the stored in relevant arrays. Write a function that will accept all the scores and compute the average score for each student. You can pass only one student’s information at a time while calling the function. Write another function for the grade computation. Display your results with last name, first name, average score and grade for each student. Display should be on screen as well as in an output file.arrow_forwardWrite a C++ program that reads 6 real numbers from the user (negative and positive numbers) into an array, then it does the following:a. Find the sum and average of positive numbers of the array and display them. b. Count negative numbers of the array and display it.c. Find the minimum value of the array and display it.d. Find the index of the minimum value of the array and display it.arrow_forward
- use c++ to write a program that manages a list of up to 10 players and their high scores in the computer's memory. Use two arrays to manage the list. One array should store the player's name and the other array should store the player's high score. Use the index of the arrays to correlate the name with the score. In Chapters 10 and 11, you will learn a different way to organize related data by putting them into a struct or class. Your program should support the following features: a. Add a new player and score. If it is one of the top 10 scores then add it to the list of scores. The same name and score can appear multiple times. For example, if Bill played 3 times and scored 100, 100, and 99, and Bob played once and scored 50, then the top scores would be Bill 100, Bill 100, Bill 99, Bob 50. b. Print the top 10 names and scores to the screen sorted by score with the highest score first. c. Allow the user to enter a player name and output that player's highest score if it is on the top…arrow_forwardplease help me with this. use only c++ code and nothing else. this topic is sorting arraysarrow_forwardWRITE C++ PROGRAM: Typhoon Casualties. Write a program using arrays to enter the following data: names of victims of typhoon Odette, age, and condition (A – alive, D – deceased, I – injured and M – missing). The size of your arrays should match the estimated number of persons in that particular barangay. Enter the name of barangay and the estimated total number of persons residing in that barangay during the typhoon. Display the following after the data entry according to their names – name (alphabetical order), condition and the count of person(s) alive, deceased, injured and missing and then get their percentage from the total population. Earthquake Incident Name of Barangay: _____________ Estimated number of residents:________ Name:____ Age:__ Condition (A,D,I or M):___ More Entry (Y/N)? Y Name:____ Age:____ Condition (A,D,I or M):___ : : Name:____ Age:____ Condition (A,D,I or M):___ More Entry (Y/N)? N List of Victims/Casualties Name Age Condition _____…arrow_forward
- C ++ Qi Write a program to ask the user to record students’ ratings between (0 — 10) for 20 students into a “responses” array, then distribute those ratings into a “frequency” array, after that print them.arrow_forwardUse C++ In part 1 you will be creating a quiz grading program. You will compare the student's answers with the correct answers, and determine passed or not. The program will make use of two parallel arrays. Each array must be able to support up to 30 characters (so you have two arrays of char or unsigned char values, each one with 30 elements). Your program will need to read in the student answer input file name from cin. It will also need to read in the correct answer file name from cin. You should use the >> operator to read from cin and not get or getline. Using get or getline will be more difficult and will require that you filter out white-space characters in your program. The contents of the student input file should be read into one char array. The file will contain up to 30 characters, each character on a separate line of the file. The first line in the input file will contain the students answer to the first question, and so on for up to 30 questions. The answers are A,…arrow_forwardPlease complete this program in C++ Please enusre that there is plenty of comments. PLENTY even if unessessary. Please include screenshots of actual code, screenshots of output, and code in the browser so I can copy and paste. Thank you.arrow_forward
- Write the following program in c++.Combine your first and second name and pick only the first five characters of your name for the project.i. Declare an array named name1 and put all the first five characters into it.ii. Write a for loop with an if condition that will find and output the index value of a searched character in the array. Let the program be such that the user will input the character being searched for.arrow_forwardCreate a simple C++ Program for the following problem and include the pseudocode. TOPIC: Data Structures, Arrays, Strings and Structs Write a program that will read in N integers from keyboard input into an array. The size ofthe array N will be entered on the keyboard. The program will tabulate the output as shownbelow: the first column should show the list of distinct array elements sorted in ascendingorder, while the second column should show the number of occurrences of each element.Sample input: 9 , 15, 7, 9, 3, 7, 3, 3, 7, 6, 3, 20arrow_forwardWrite a C++ program that will read monthly sales into a dynamically allocated array. The program will input the size of the array from the user (must be less than or equal to 12). It will call a function that will find the sum of all sales. It will also call a function to find the average. It will also call a function to sort the array using either a bubble or selection sort in descending order and then display the results. You must have a comment block header at the top of your program. See the Standards document in Course Content You must use pointers for your arrays and dynamically allocate them based on the number of month You must remove and reset the array when completed You must use the correct data types for your variables You must validate your input. The number of months must be between 1 and 12 You must use a loop to read in the sales You must use at least 3 functions to calculate the average, sort the array and display the sales Your output should be…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
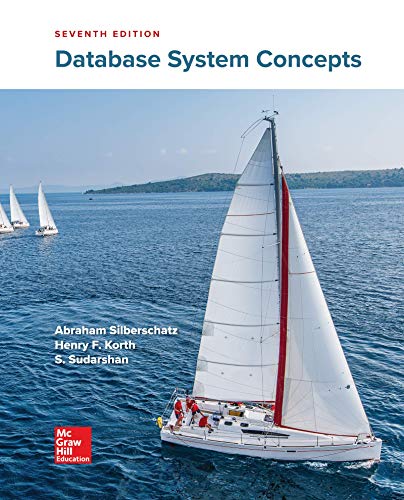
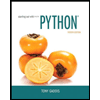
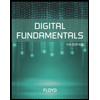
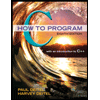
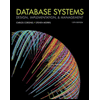
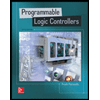