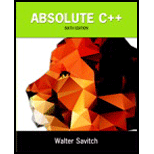
1 . The following variables are used in the program:
- seq variable is of integer type and used to store the user-entered Fibonacci sequence.
- nvariable is of integer type and used as a parameter of the function name findFibonacciNumber.
2. The following functions are used in the program:
- findFibonacciNumber ()function is used to find the Fibonacci number of the user-entered sequence.
- main () function is used to get the user input, call the function, and display the Fibonacci number returned by the function.
Program description:
The main purpose of the program is to create a function named findFibonacciNumber . This function get the sequence of the Fibonacci series as parameter, then calculate the Fibonacci number forsequence, and return the Fibonacci number.

Explanation of Solution
Program:
//including essential header file #include <iostream> //using standard namespace using namespace std; //function to find the Fibonacci number of the given sequence int findFibonacciNumber(int n) { //when the fibonacci sequence is equal to 0 or 1 if (n == 0 || n == 1) { //return 1 return 1; } //call the function recursively to find the fibonacci number return findFibonacciNumber(n-1) + findFibonacciNumber(n-2); } //main function int main() { //variable declaration to store the sequence of Fibonacci number int seq; //prompt the user to enter the fibonacci sequence cout<<"Enter the fibonacci sequence: "; //get the value from the user cin>>seq; //call the function to find and display the fibonacci number of the given sequence cout<<"The fibonacci number of sequence "<<seq<<" is "<<findFibonacciNumber(seq); }
Explanation:
In the above code, a recursive function named findFibonacciNumber is used to find the Fibonacci number of the user-entered sequence. It has a parameter named n of integer type. It gets the sequence of the Fibonacci series from the main () function and stored in the parameter named n. When the value of the Fibonacci sequence is 0 or 1 then this function returns 1. For the other values this function return the Fibonacci number for the given sequence by adding the immediate last two Fibonacci number by calling itself recursivelyand decreasing the value of the parameter by 1 (to get the immediate last value) and 2 (to get the second last value).
In the main function, create a variable named seq of integer type to store the user-entered Fibonacci sequence. Prompt the user to enter the Fibonacci sequence. Store the user-entered Fibonacci sequence in the variable name seq. Call the function named findFibonacciNumber with seq as parameter. Display the function returned value.
Sample output:
Want to see more full solutions like this?
Chapter 13 Solutions
Absolute C++
- In C programming Mathematically, given a function f, we recursively define fk(n) as follows: if k = 1, f1(n) = f(n). Otherwise, for k > 1, fk(n) = f(fk-1(n)). Assume that there is an existing function f, which takes in a single integer and returns an integer. Write a recursive function fcomp, which takes in both n and k (k > 0), and returns fk(n). int f(int n);int fcomp(int n, int k){arrow_forwardWrite an iterative function that determines the number of even elements in an array a of integers of size n. The function should return the number of elements that are even in array a of size n. Propose an appropriate prototype for your function and then write its code. Write a recursive function to solve the above problem. Propose an appropriate prototype for your function and then write its code.arrow_forwardA recursive function is a function defined in terms of itself via self-referential expressions. This means that the function will continue to call itself and repeat its behavior until some condition is met to return a result. a- Write a python recursive function prod that takes x as an argument, and returns the result where, result=1*1/2*1/3*….*1/n b- Include a screenshot that shows a python program that uses the above function and prints the rounded result to three decimal places after prompting the user to enter a number, x. Use x=3. N.B: The code should be included in your answer.arrow_forward
- In Kotlin A. Write a function lengthGreaterThan() with an expression body that takes a String s and an Int n and returns true if the String is at least n characters long. Then revise the function so that it can accept null values. If it receives null as the parameter, it should return false. B. Write a recursive function with an expression body that takes a list of nullable Strings and and Int an returns a list of just the Strings from the original list for which the function you wrote in the first part returns true C. Get the same result as in part B by using filter()arrow_forwardMULTIPLE FUNCTIONS AND RECURSIVE FUNCTIONS .arrow_forwardWrite a program that performs the following functionalities:1. Fibonacci: a recursive function that computes the fibonacci series, which is defined as followsfibonacci (n) = fibonacci(n-2) + fibonacci(n-1)fibonacci(0) = 0fibonacci(1) = 1 So the fibonacci looks like: 0 1 1 2 3 5 8 13 21 34 …. Therefore, fibonacci(4) = 3, fibonacci(5) = 5, fibonacci(7)=13 2. Sum: a recursive function that computes the sum of integers 1, 2, 3, …., n for a given number n. So Sum(6) should return 1 + 2 + 3 + 4 + 5 + 6 , i.e. 21.sum(n) = n + sum(n-1) 3. Largest: a recursive function that computes the largest value for an integer array of positiveand negative values. For example, for the array below, the function largest should return 22,which is the largest value in the array. You can assume there are no more 20 integers in thearray. Think of how to formulate the recurrence relation in this problem yourself. 4. The 4th problem mimics the situation where eagles flying in the sky can be spotted and…arrow_forward
- Question 1. Consider the following difference equation: With ro := 130, set rn := 0.0001 - 0,0194r1 + 1.6909r-1 (n = 1, 2, 3, ...). Question 1.1. Implement a non-recursive function in Python 3 according to the following specification. Include your implementation in your submission. Function specification: Function name: Input parameters: n: a non -negative integer Return The value of r. Question 1.2. Use matplotlib to make a scatter plot of the the first 21 elements in the solution of the difference equation. That is, make a scatter plot of the dataset {(J.r,) : 1 = 0,1, 2, 3,..., 20). Using the "set-ylim" function on your matplotlib axes, set the bounds of the y-axis to be the interval (0,200].arrow_forwardIn programming, a recursive function calls itself. The classical example is factorial(n), which can be defined recursively as n*factorial(n-1). Nonethessless, it is important to take note that a recursive function should have a terminating condition (or base case), in the case of factorial, factorial(0)=1. Hence, the full definition is: factorial(n) = 1, for n = 0 factorial(n) = n * factorial(n-1), for all n > 1 For example, suppose n = 5: // Recursive call factorial(5) = 5 * factorial(4) factorial(4) = 4 * factorial(3) factorial(3) = 3 * factorial(2) factorial(2) = 2 * factorial(1) factorial(1) = 1 * factorial(0) factorial(0) = 1 // Base case // Unwinding factorial(1) = 1 * 1 = 1 factorial(2) = 2 * 1 = 2 factorial(3) = 3 * 2 = 6 factorial(4) = 4 * 6 = 24 factorial(5) = 5 * 24 = 120 (DONE) Exercise (Factorial) (Recursive): Write a recursive method called factorial() to compute the factorial of the given integer. public static int factorial(int n) The recursive algorithm is:…arrow_forward*19. A recursive function f (x), is defined as follows: if (x>100) return (x-10) else return (f (f (x+11) ) ) For which of the following values of x, (a) 100 (b) 91 f(x) (c) 1 = 91? (d) 101arrow_forward
- The 4th problem mimics the situation where eagles flying in the sky can be spotted and counted.FindEagles: a recursive function that examines and counts the number of objects (eagles) in aphotograph. The data is in a two-dimensional grid of cells, each of which may be empty (value 0) orfilled (value 1 to 9). Maximum grid size is 50 x 50. The filled cells that are connected form an object(eagle). Two cells are connected if they are vertically, horizontally, or diagonally adjacent. Thefollowing figure shows 3 x 4 grids with 3 eagles. 0 0 1 21 0 0 01 0 3 1 FindEagle function takes as parameters the 2-D array and the x-y coordinates of a cell that is a part ofan eagle (non-zero value) and erases (change to 0) the image of an eagle. The function FindEagleshould return an integer value that counts how many cells has been counted as part of an eagle and havebeen erased. The following sample data has two pictures, the first one is 3 x 4, and the second one is 5 x 5 grids. Notethat your program…arrow_forwardWrite a recursive function power( base, exponent ) that when invoked returns baseexponentFor example, power( 3, 4 ) = 3 * 3 * 3 * 3. Assume that exponent is an integer greater than orequal to 1. Hint: The recursion step would use the relationship baseexponent = base * baseexponent1and the terminating condition occurs when exponent is equal to1 becausebase1 = base c languagearrow_forwardConsider the following recursive function:int Func(int num){if (num == 0)return 0;elsereturn num+Func(num+1);}1. Is there a constraint on the values that can be passed as a parameter for this function to passthe smaller-caller question?2. Is Func(7) a good call? If so, what is returned from the function?3. Is Func(0) a good call? If so, what is returned from the function?4. Is Func(-5) a good call? If so, what is returned from the function?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
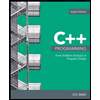