Find the error(s) in the following code: (6)

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
EBK C PROGRAMMING:
- Can I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forward
- Need help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
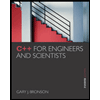
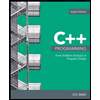
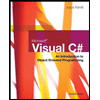
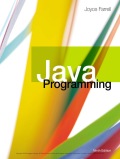
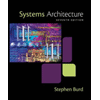