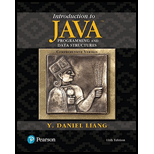
(Triangle class) Design a new Triangle class that extends the abstract GeometricObject class. Draw the UML diagram for the classes Triangle and GeometricObject then implement the Triangle class. Write a test

Program to create Triangle class
Program Plan:
- Include a class name named “Exercise_13_01”.
- Include the scanner package.
- Declare the main() function.
- Create a scanner object.
- Prompt the user to enter three sides of the triangle.
- Prompt the user to enter a color.
- Prompt the user to enter a Boolean value on whether the triangle is filled.
- Create triangle object with the entered user input.
- Set color and set filled in the triangle.
- Print the output.
- Close the main() function.
- Close the class “Exercise_13_01”.
- Include an abstract class “GeometricObject”.
- Declare all the data types of the variables.
- Construct a default geometric object.
- Construct a geometric object with color and filled value.
- Assign color and fill to the object.
- Set a new color.
- Define an isFilled method returns filled and since filled is Boolean; the get method is named isFilled.
- Set a new filled and getDateCreated method.
- Override toString function to return output.
- Define Abstract method getArea.
- Define Abstract method getPerimeter.
- Close class.
- Include a class “Triangle” that extends the class GeometricObject.
- Declare the three sides of the triangle.
- Include a triangle constructor.
- Assign values to all three sides of the triangle.
- Assign triangle values to all attributes.
- Return side1 and Set side1 to a new length.
- Return side2 and Set side2 to a new length.
- Return side3 and Set side3 to a new length.
- Create a Function to return area of the Triangle.
- Create a function to return a string description of the object.
- Close class.
The java code to design a new Triangle class that extends the abstract GeometricObject class and to design a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled or not.
Explanation of Solution
Program:
File name: Exercise_13_01.java
//package to have user input
import java.util.Scanner;
//class Definition
public class Exercise_13_01
{
// Main method
public static void main(String[] args)
{
// Create a Scanner object
Scanner input = new Scanner(System.in);
/* Prompt the user to enter three sides of the triangle */
System.out.print("Enter three side of the triangle: ");
double side1 = input.nextDouble();
double side2 = input.nextDouble();
double side3 = input.nextDouble();
// Prompt the user to enter a color
System.out.print("Enter a color: ");
String color = input.next();
/*Prompt the user to enter a boolean value on whether the triangle is filled*/
System.out.print("Is the triangle filled (true / false)? ");
boolean filled = input.nextBoolean();
/* Create triangle object with the entered user input */
Triangle triangle = new Triangle(side1, side2, side3);
// set color
triangle.setColor(color);
// set filled
triangle.setFilled(filled);
// print the output
System.out.println(triangle.toString());
System.out.println("Area: " + triangle.getArea());
System.out.println("Perimeter: " + triangle.getPerimeter());
System.out.println("Color: " + triangle.getColor());
System.out.println("Triangle is" + (triangle.isFilled() ? "" : " not ")
+ "filled");
}
}
Filename: GeometricObject.java
//abstract class GeometricObject definition
public abstract class GeometricObject
{
//data type declaration
private String color = "while";
private boolean filled;
private java.util.Date dateCreated;
// Construct a default geometric object
protected GeometricObject()
{
dateCreated = new java.util.Date();
}
/* Construct a geometric object with color and filled value */
protected GeometricObject(String color, boolean filled)
{
dateCreated = new java.util.Date();
//assign color and fill
this.color = color;
this.filled = filled;
}
// Return color
public String getColor()
{
return color;
}
// Set a new color
public void setColor(String color)
{
this.color = color;
}
/* isFilled method returns filled and Since filled is boolean,the get method is named isFilled */
public boolean isFilled()
{
return filled;
}
// Set a new filled
public void setFilled(boolean filled)
{
this.filled = filled;
}
// Get dateCreated
public java.util.Date getDateCreated()
{
return dateCreated;
}
//override toString function to return output
@Override
public String toString()
{
return "created on " + dateCreated + "\ncolor: " + color +
" and filled: " + filled;
}
// define Abstract method getArea
public abstract double getArea();
//Define Abstract method getPerimeter
public abstract double getPerimeter();
}
Filename: Triangle.Java
/*Class definition of Triangle that extends the class GeometricObject */
public class Triangle extends GeometricObject
{
//declare the three sides of the triangle
private double side1;
private double side2;
private double side3;
//triangle constructor
public Triangle()
{
}
//assign values to all three sides of the triangle
public Triangle(double side1, double side2, double side3)
{
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
//assign triangle values to all attributes
public Triangle(double side1, double side2, double side3, String color, boolean filled)
{
this(side1, side2, side3);
setColor(color);
setFilled(filled);
}
// Return side1
public double getSide1()
{
return side1;
}
// Set side1 to a new length
public void setSide1(double side1)
{
this.side1 = side1;
}
// Return side2
public double getSide2()
{
return side2;
}
// Set side2 to a new length
public void setSide2(double side2)
{
this.side2 = side2;
}
// Return side3
public double getSide3()
{
return side3;
}
// Set side3 to a new length
public void setSide3(double side3)
{
this.side3 = side3;
}
// Function to Return area of the Triangle
@Override
public double getArea()
{
double s = (side1 + side2 + side3) / 2;
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
/* override function to Return perimeter of the triangle */
@Override
public double getPerimeter()
{
return side1 + side2 + side3;
}
/*override function to Return a string description of the object*/
@Override
public String toString()
{
return super.toString() + "\nArea: " + getArea() + "\nPerimeter: " + getPerimeter();
}
}
UML Diagram:
Explanation:
- Here, GeometricObject is an abstract class that extends the triangle class which is represented using an arrow. The triangle class then contains a rectangle that represents the objects used to input the three sides of the triangle.
- The remaining part is used to represent all the methods and functions used in the triangle class.
- The Triangle() method creates a triangle with default sides. The method Triangle(side1:double, side2; double,side3;double) creates a triangle of the specified sides.
- Then the respective sides of the triangles are returned using getSide() methods.
- Then the method getArea() returns the area of the triangle and the method getPerimeter() returns the perimeter of the triangle and the function toString() returns a string description of the object.
Enter three side of the triangle: 3
4
5
Enter a color: red
Is the triangle filled (true / false)? false
created on Sun Jul 08 13:24:03 IST 2018
color: red and filled: false
Area: 6.0
Perimeter: 12.0
Area: 6.0
Perimeter: 12.0
Color: red
Triangle is not filled
Want to see more full solutions like this?
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- 1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forward
- using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardusing r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
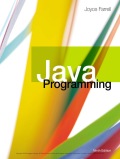
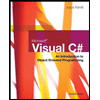
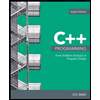
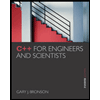
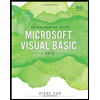