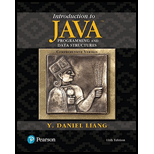
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 13.6, Problem 13.6.5CP
What is wrong in the following code?
public class Test {
public static void main(String[] args) {
Person[] persons = {new Person(3), new Person(4), new Person(1)};
java.util.Arrays.sort(persons);
}
}
class Person {
private int id;
Person(int id) {
this.id = id;
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
6. What is Race condition? How to prevent it? [2 marks]
7. How many synchronization methods do you know and compare the differences. [2 marks]
8. Explain what are the “mutual exclusion”, “deadlock”, “livelock”, and “eventual entry”, with
the traffic intersection as an example like dinning philosophy. [2 marks]
9. For memory allocation, what are the difference between internal fragmentation and external
fragmentation. Explain with an example. [2 marks]
10. How can the virtual memory map to the physical memory. Explain with an example. [2
marks]
Your answers normally have 50 words. Less than 50 words will not get marks.
1. What is context switch between multiple processes? [2 marks]
2. Draw the memory layout for a C program. [2 marks]
3. How many states does a process has? [2 marks]
4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks]
5. Given 4 process and their arrival times and next CPU burst times, what are the average times
and average Turnaround time, for different scheduling algorithms including:
a. First Come, First-Served (FCFS) Scheduling [2 marks]
b. Shortest-Job-First (SJF) Scheduling [2 marks]
c. Shortest-remaining-time-first [2 marks]
d. Priority Scheduling [2 marks]
e. Round Robin (RR) [2 marks]
Process
Arrival Time
Burst Time
P1
0
8
P2
1
9
P3
3
2
P4
5
4
a database with multiple tables from attributes as shown above that are in 3NF,
showing PK, non-key attributes, and FK for each table? Assume the tables are already in
1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 13.2 - Prob. 13.2.1CPCh. 13.2 - The getArea() and getPerimeter() methods may be...Ch. 13.2 - True or false? a.An abstract class can be used...Ch. 13.3 - Prob. 13.3.1CPCh. 13.3 - Prob. 13.3.2CPCh. 13.3 - Prob. 13.3.3CPCh. 13.3 - What is wrong in the following code? (Note the...Ch. 13.3 - What is wrong in the following code? public class...Ch. 13.4 - Can you create a Calendar object using the...Ch. 13.4 - Prob. 13.4.2CP
Ch. 13.4 - How do you create a Calendar object for the...Ch. 13.4 - For a Calendar object c, how do you get its year,...Ch. 13.5 - Prob. 13.5.1CPCh. 13.5 - Prob. 13.5.2CPCh. 13.5 - Prob. 13.5.3CPCh. 13.5 - Prob. 13.5.4CPCh. 13.6 - Prob. 13.6.1CPCh. 13.6 - Prob. 13.6.2CPCh. 13.6 - Can the following code be compiled? Why? Integer...Ch. 13.6 - Prob. 13.6.4CPCh. 13.6 - What is wrong in the following code? public class...Ch. 13.6 - Prob. 13.6.6CPCh. 13.6 - Listing 13.5 has an error. If you add list.add...Ch. 13.7 - Can a class invoke the super.clone() when...Ch. 13.7 - Prob. 13.7.2CPCh. 13.7 - Show the output of the following code:...Ch. 13.7 - Prob. 13.7.4CPCh. 13.7 - What is wrong in the following code? public class...Ch. 13.7 - Show the output of the following code: public...Ch. 13.8 - Prob. 13.8.1CPCh. 13.8 - Prob. 13.8.2CPCh. 13.8 - Prob. 13.8.3CPCh. 13.9 - Show the output of the following code: Rational r1...Ch. 13.9 - Prob. 13.9.2CPCh. 13.9 - Prob. 13.9.3CPCh. 13.9 - Simplify the code in lines 8285 in Listing 13.13...Ch. 13.9 - Prob. 13.9.5CPCh. 13.9 - The preceding question shows a bug in the toString...Ch. 13.10 - Describe class-design guidelines.Ch. 13 - (Triangle class) Design a new Triangle class that...Ch. 13 - (Shuffle ArrayList) Write the following method...Ch. 13 - (Sort ArrayList) Write the following method that...Ch. 13 - (Display calendars) Rewrite the PrintCalendar...Ch. 13 - (Enable GeometricObject comparable) Modify the...Ch. 13 - Prob. 13.6PECh. 13 - (The Colorable interface) Design an interface...Ch. 13 - (Revise the MyStack class) Rewrite the MyStack...Ch. 13 - Prob. 13.9PECh. 13 - Prob. 13.10PECh. 13 - (The Octagon class) Write a class named Octagon...Ch. 13 - Prob. 13.12PECh. 13 - Prob. 13.13PECh. 13 - (Demonstrate the benefits of encapsulation)...Ch. 13 - Prob. 13.15PECh. 13 - (Math: The Complex class) A complex number is a...Ch. 13 - (Use the Rational class) Write a program that...Ch. 13 - (Convert decimals to fractious) Write a program...Ch. 13 - (Algebra: solve quadratic equations) Rewrite...Ch. 13 - (Algebra: vertex form equations) The equation of a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What will the following code display? for number in range(10, 5, 1): print(number)
Starting Out with Python (4th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Empl Id Name Address SSN Job Id Job Title Skill Cod Dept Start Date Term Date 25X15 Joe E. Baker 33 Nowhere St ...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Show a snippet of PHP code for creating a recordset. Explain the meaning of the code.
Database Concepts (8th Edition)
Is the following comment a single-line style comment or a multi-line style comment? / This program was written ...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Rainfall Program Modification Recall that Programming Exercise 3 in Chapter 8 asked you to design a program tha...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forwardIf a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forward
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- Please answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forward
- Why is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
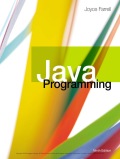
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
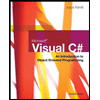
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
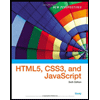
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
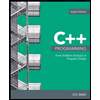
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
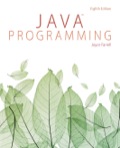
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
6 Stages of UI Design; Author: DesignerUp;https://www.youtube.com/watch?v=_6Tl2_eM0DE;License: Standard Youtube License