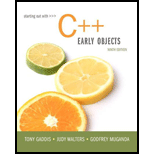
What is the output of the following
#include <iostream>
using namespace std;
// Function prototype
void mess(char []);
int main()
{
char stuff[] = "Tom Talbert Tried Trains";
cout << stuff << endl;
mess(stuff);
cout << stuff << endl;
return 0;
}
// Definition of function mess
void mess(char str[])
{
int step = 0;
while (str[step] != '\0')
{
if (str[step] == 'T')
strf[step] = 'D';
step++;
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Database Concepts (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Electric Circuits. (11th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- C++arrow_forward1. C++: Complete the following incomplete code to a executable C++ program): [4C Open with Google Docs class Array{ int* dataPtr; int noofElements; float avg; public: // define necessary getter and setter method (s) // define other necessary method (s) to support the main () //NO friend is ALLOWED }; class ArrayOfArrays { Array* ptrToArrays; int noofArrays; public: // define method: "setArrayofArrays" // set random integers and calculate avg to the fields for // each of ptrToArrays [i] [see RUN] // define method: "showArrayofArrays" // show elements and avg as per the RUN // define method: "getAvgofAvgs" // display the average of array-averages as per the RUN //NO friend is ALLOWED }; int main ()) { ArrayofArrays obj; int n = rand () 810, max = 100; // n = no of Array to be pointed by ptrToArrays in obj, i.e. noofArrays // max is the upper limit of random values for Array elements in dataPtr obj.setArrayOfArrays (n, max).showArrayofArrays () ; // Explanation of setArrayofArrays (n,…arrow_forwardC++ need help with part d to g only Assume the Product structure is declared as follows: struct Product { string description; // Product description int partNum; // Part number double cost; // Product cost }; (c) Declare a dynamic array of Products of size 5 and name it ”items”. Then initilize it with user input values. (d) Write a print function (not as a member of the struct) and pass a pointer to the pointer that points to the array (double pointer) and print all the items of the array in that function. (e) Define a max function (not as a member of the struct) that gets an array of items as an input and returns a pointer to the max element of the array. (f) Declare a 3 by 3 two dimensional dynamic array and populate it. (g) Define an output function that takes a stream object and a pointer to a 2D array as arguments and outputs data members of objects in format of 3*3 table into the given stream. Test your function both with an output file…arrow_forward
- True or False : The concept of function abstraction hinders our code development by confusing us with the details of the function.arrow_forwardProgramming Skills: Complete the function egg_category which returns a str describing an egg's category given its int weight in grams. Here is a table specifying the weight ranges if an egg's weight is on the boundary between two category ranges, it is assigned the smaller category. Category Small Meduim Weight no more than 50 grams 50-57 grams Category Large Jumbo Weight 57-64 grams more than 64 grams def egg_category (weight): %# Return a str describing the category of an egg of the specified int weight.arrow_forwardAssume the Product structure is declared in c++ as follows:struct Product{string description; // Product descriptionint partNum; // Part numberdouble cost; // Product cost}; Now write a definition for an array of 100 Product structures. Do not initialize the array. ( Drop screenshot of output as well )arrow_forward
- c++ Here are struct data members: integer for the ID of a student character array for the name. The size of the array is 15 floating-point numbers for the GPA an integer for a student status Write the declaration of the struct, lines of code to dynamically allocate size of the struct defined previously, the user will be asked to enter the size of the array, and a function that receives an array of the struct, the length of the array and the student ID as parameters. It should return the name, GPA and student status if found. Make sure to address if not found.arrow_forwardIn C Programming: You will need all the functions from the previous assignment, as well as the structure course:• Department (string, 15 characters)• Course number (integer, 4 digits, leading 0 if necessary)• Course title (string, 30 characters)• Credits (short, 1 digit) Write a function saveAllCoursesText() which receives an array of course pointers and the array’s size, then outputs the content of the array in text format to a file named “courses.txt”.• Save the entire structure on 1 line (all the members of the structure should be saved in 1 fprintf() command)arrow_forward6: Write a program in C++ that contains overloaded functions with name óvoperation(). When the main program makes a call to Ovoperation the program performs the following according to the arguments of the function call: • Ovoperation(): returns an int 0. • Ovoperation(float, float) returns the maximum between the arguments. • Ovoperation(int, int, int) returns the minimum value among the arguments. • Ovoperation{char) return the next ASCII character (e.g. OpOperation('a') returns b').arrow_forward
- dreaaarrow_forwardSTRUCTURES IN C The circle has two data members, a Point representing the center of the circle and a float value representing the radius as shown below. typedef struct{ Point center; float radius;} Circle; typedef struct{ int x; int y;}Point; Implement the following functions:a. float distance (Line l1);- computes and returns the distance between the two points of the line.distance = sqrt((x2-x1)^2+(y2-y1)^2) b. float diameter(Circle circ);- computes the diameter of a circle.arrow_forwardfunction carLambda = [rank, &price] ()->int { cout name); model Car:: carModel; auto testLambdaPtr = testLambda (); cout << testLambdaPtr () << endl; return 0; I a. In which memory area is this element stored? Please state your choice and explain why? b. The lifetime, beginning & end, of this element? Why?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
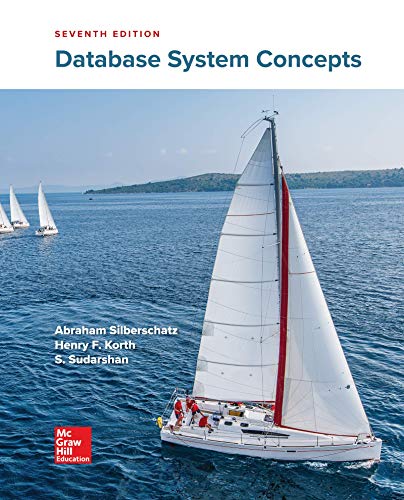
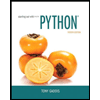
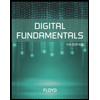
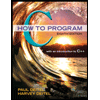
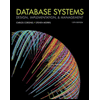
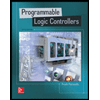