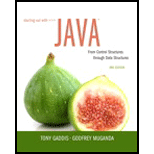
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 12, Problem 2FTE
Explanation of Solution
The given statement:
//define a class which implements ActionListener
private class ButtonListener implements ActionListener
{
//override the method actionPerformed()
public void actionPerformed()
{
// Code appears here.
}
}
Error in the code:
The “actionPerformed()” method must have an “ActionEvent” parameter...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 12 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 12.2 - Prob. 12.1CPCh. 12.2 - Prob. 12.2CPCh. 12.2 - Prob. 12.3CPCh. 12.2 - Prob. 12.4CPCh. 12.2 - Prob. 12.5CPCh. 12.2 - Prob. 12.6CPCh. 12.2 - If you are writing an event listener class for a...Ch. 12.2 - Prob. 12.8CPCh. 12.2 - Prob. 12.9CPCh. 12.3 - Prob. 12.10CP
Ch. 12.3 - Prob. 12.11CPCh. 12.3 - Which layout manager arranges components in a row,...Ch. 12.3 - Prob. 12.13CPCh. 12.3 - Prob. 12.14CPCh. 12.3 - Prob. 12.15CPCh. 12.3 - Prob. 12.16CPCh. 12.3 - Prob. 12.17CPCh. 12.4 - Prob. 12.18CPCh. 12.4 - Prob. 12.19CPCh. 12.4 - Prob. 12.20CPCh. 12.4 - Prob. 12.21CPCh. 12.4 - Prob. 12.22CPCh. 12.4 - Prob. 12.23CPCh. 12.4 - Prob. 12.24CPCh. 12.4 - Prob. 12.25CPCh. 12.5 - Prob. 12.26CPCh. 12.5 - Prob. 12.27CPCh. 12 - With Swing, you use this class to create a frame....Ch. 12 - Prob. 2MCCh. 12 - Prob. 3MCCh. 12 - Prob. 4MCCh. 12 - Prob. 5MCCh. 12 - Prob. 6MCCh. 12 - Prob. 7MCCh. 12 - Prob. 8MCCh. 12 - Prob. 9MCCh. 12 - Prob. 10MCCh. 12 - Prob. 11MCCh. 12 - Prob. 12TFCh. 12 - Prob. 13TFCh. 12 - Prob. 14TFCh. 12 - Prob. 15TFCh. 12 - Prob. 16TFCh. 12 - Prob. 17TFCh. 12 - Prob. 18TFCh. 12 - Prob. 19TFCh. 12 - Prob. 20TFCh. 12 - The following statement is in a class that uses...Ch. 12 - Prob. 2FTECh. 12 - Prob. 3FTECh. 12 - Prob. 4FTECh. 12 - Prob. 5FTECh. 12 - Prob. 1AWCh. 12 - Prob. 2AWCh. 12 - The variable myWindow references a JFrame object....Ch. 12 - Prob. 4AWCh. 12 - Prob. 5AWCh. 12 - Prob. 6AWCh. 12 - Prob. 7AWCh. 12 - Prob. 8AWCh. 12 - Prob. 9AWCh. 12 - Prob. 1SACh. 12 - Prob. 2SACh. 12 - Prob. 3SACh. 12 - Prob. 4SACh. 12 - Retail Price Calculator Create a GUI application...Ch. 12 - Prob. 2PCCh. 12 - Prob. 3PCCh. 12 - Travel Expenses Create a GUI application that...Ch. 12 - Prob. 5PCCh. 12 - Joes Automotive Joes Automotive performs the...Ch. 12 - Prob. 8PC
Knowledge Booster
Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
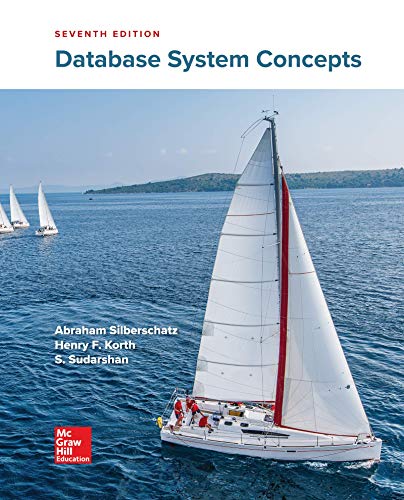
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
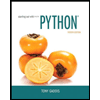
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
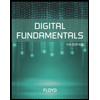
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
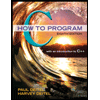
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
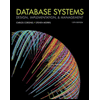
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
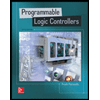
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education