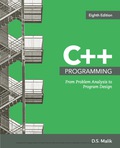
Explanation of Solution
The errors are in the following lines:
class hourlyEmployee: public class employee - has the word “class” mentioned before the base class.
public:: - should be public:
void setHoursWorked(double hrsWk) const; - set functions should not be constant functions as they modify data members.
void setPay() const; - set functions should not be constant functions as they modify data members.
private; - should be private:
Hence the correct program is:
class hourlyEmployee: public employee
{
public:
void setData(string n = "", string d = "", int a = 0,
double p = 0, double hrsWk = 0,
double payRate = 0.0);
// Data members are set according to the parameters.
// Values assigned to numeric data is nonnegative.
void setHoursWorked(double hrsWk);
// Function to set hours worked.
// if hrsWk>= 0, hoursWorked = hrsWk;
// Otherwise hoursWorked = 0;
double getHoursWorked() const;
// returns the value of hoursWorked...

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
EBK C++ PROGRAMMING: FROM PROBLEM ANALY
- class Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardclass Vehicle { private: int wheels; protected : int passenger: public : void inputdata(int, int); void outputdata(); }; class Heavyvehicle : protected Vehicle { int diesel_petrol; protected : int load; public: void readdata(int, int); void writedata(); }; class Bus : private Heavyvehicle char make[20]; public : void fetchdata(char); void displaydata(); (1) Name the base class and derived class of the class Heavyvehicle. (ii) Name the data member(s) that can be accessed from function displaydata(). (iii) Name the data member's that can be accessed by an object of Bus class. (iv) Is the member function outputdata() accessible to the objects of Heavyvehicle class.arrow_forwardpublic class Application { public static void main(String[] args) { Employee empl = new Employee(); empl.firstName = "Ali"; empl.lastName = "Omar"; empl.salary = 20000; Employee emp2 = new Employee(); emp2.firstName = "Mohamed"; emp2.lastName = "Nour"; emp2.salary = 30000; System.out.println("- System.out.printin("- empl.employeeSalary(); System.out.println("- empl.checkEmployeeSalary(emp1.salary, emp2.salary); System.out.printin("- System.out.println("- printEmployee(emp2); System.out.printin("- Employee employeeArr[] = new Employee[2]: employeeArr[0] = empl; employeeArr[1] = emp2; Application a = new Åpplication(); a.printArrayofEmployee(employeeArr); Non static (instance) start to call Static function to print ---------"); "); - Non Static function to print - --"); Non static (instance) start to call same class Static function to print --------); -"); Non Static function to print ----); System.out.println("- System.out.printin("- System.out.printin("Salary : " + Employee.salary);…arrow_forward
- Trace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forwardthis is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12,…arrow_forwardclass Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String courseName) { this.courseName = courseName; } public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }} class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList<MyCourse> courseList; public String…arrow_forward
- class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String courseName) { this.courseName = courseName; } public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }}class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList courseList; public String getFullName() {…arrow_forwardPublic classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forward26. Assume the declaration of Exercise 24. A. Write the statements that derive the class dummyClass from class base as a private inheritance. (2 lines are { and }.) "A1 is { "A2 is "A3 is )) B. Determine which members of class base are private, protected, and public in class dummyClass. "B1 is dummyClass is a dearrow_forward
- For the following four classes, choose the correct relationship between each pair. public class Room ( private String m type; private double m area; // "Bedroom", "Dining room", etc. // in square feet public Room (String type, double area) m type type; m area = area; public class Person { private String m name; private int m age; public Person (String name, int age) m name = name; m age = age; public class LivingSSpace ( private String m address; private Room[] m rooms; private Person[] m occupants; private int m countRooms; private int m countoccupants; public LivingSpace (String address, int numRooms, int numoccupants) m address = address; new int [numRooms]; = new int [numOceupants]; m rooms %3D D occupants m countRooms = m countOccupants = 0; public void addRoom (String type, double area)arrow_forwardpublic class Stadium extends baseStadium{ public int typeTicket; int floors; int seating; String type; public float ticketPrice; public String leagueName=""; public void SetTecketName(String val) { leagueName=val; } public void setType() { if (typeTicket == 1) { type = "VIP"; } else { type = "Regular"; } } public String getType() { return type; } public void setPrice() { if (typeTicket == 1) { ticketPrice = 250; } else { ticketPrice = 100; } } public void setFloors(int floors) { this.floors = floors; } public int getFloors() { return floors; } public void setSeating(int seating) { this.seating = seating; } public int getSeating() { return seating; } public void printDetails() { System.out.println("Ticket Details:"); System.out.println("Ticket Type:…arrow_forwardStudent Name: 8) Consider the following ciass definitions. public class LibraryID{ private String name; // Person's name private int ID; // Person's library ID # private ArrayList Books; // Loaned books public LibraryID (String n, int num) { name = n; ID = num; Books = new ArrayList(); } public int getID () { return ID; } public String getName() { return name; } /* CheckOut () and ReturnBook () methods not shown */ Complete the following override method, contained in the LibraryID class. Two LibraryID's are considered the same if their ID numbers are the same. public boolean equals (Object other) { if (other == null){ return false;} LibraryID o = (LibraryID) other; 9) Assuming the code written in 8 is correct, write the call to compare LibraryID one and two inside the if statement. LibraryID one = new LibraryID("Mankind", 106); LibraryID two = new LibraryID ("Mick Foley", 106); if System.out.println("ID's belong to the same person"); else System.out.println("ID's belong to the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
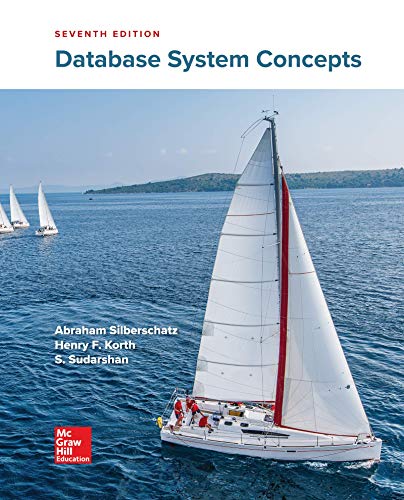
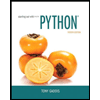
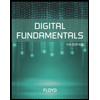
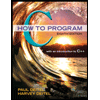
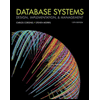
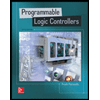