Concept explainers
Palindrome Testing
A palindrome is a string that reads the same backward as forward. For example, the words worn, dad, madam and radar are all palindromes. Write a class Pstring that is derived from the STL string class. The Pstring class adds a member function
bool isPalindrome( )
that determines whether the string is a palindrome. Include a constructor that takes an STL string object as parameter and passes it to the string base class constructor. Test your class by having a main
You may find it useful to use the subscript operator [ ] of the string class: If str is a string object and k is an integer, then str[k] returns the character at position k in the string.

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Starting Out with Programming Logic and Design (4th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Problem Solving with C++ (9th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- In C++ yhou will create a new class named StringMod that will have several functions specific tostring. Here is a UML diagram:StringMod- str : string+ StringMod()+ StringMod(string);+ size() const : int+ backwards() const : string+ count(char) const : int+ getString() const : string+ uppercase() const : string+ lowercase() const : string+ erase() : void+ setString(string) : voidFirst of all, none of these functions should contain output statements! No cout, no cin, in any of thesefunctions!As you can see, several of the methods of StringMod are const, meaning they won’t change theinternal string. You’ll likely declare a local string which will be updated and then returned.The default constructor just sets str to an empty string, "". The constructor with parameters should justcall setString() to set str to the value passed to the constructor.backwards() returns a new string that is the reverse of the string in str.uppercase() takes every letter in the string and capitalizes it, while…arrow_forwardTerm by CodeChum Admin (JAVA CODE) Construct a class called Term. It is going to represent a term in polynomial expression. It has an integer coefficient and an exponent. In this case, there is only 1 independent variable that is 'x'. There should be two operations for the Term: public Term times(Term t) - multiplies the term with another term and returns the result public String toString() - prints the coefficient followed by "x^" and appended by the exponent. But with the following additional rules: if the coefficient is 1, then it is not printed. if the exponent is 1, then it is not printed ( the caret is not printed as well) if the exponent is 0, then only the coefficient is printed. Input The first line contains the coefficient and the exponent of the first term. The second line contains the coefficient and the exponent of the second term. 1·1 4·3 Output Display the resulting product for each of the test case. 4x^4arrow_forwardComputer sciencearrow_forward
- javascript Need help defining a function frequencyAnalysis that accepts a string of lower-case letters as a parameter. frequencyAnalysis should return an object containing the amount of times each letter appeared in the string. example frequencyAnalysis('abca'); // => {a: 2, b: 1, c: 1}arrow_forwardIN C++ Write code that: creates 3 integer values - one can be set to 0, the other two should NOT multiples of one another (for example 3 and 6, or 2 and 8) take you largest value and perform a modulus operation using the smaller (non zero) value as the divisor print out the result. create an alias for the string type call the alias "name" then create an instance of the "name" class and assign it a value using an appropriate cout statement - print out the value Please screenshot your input and output, as the format tends to get messed up. Thank you!arrow_forwardJAVA PROGRAM SEE ATTACHED PHOTO FOR THE PROBLEMarrow_forward
- Write a class BCheckString that is derived from the STL string class. This new class will have two member functions: A) A BCheckString(string s) constructor that receives a string object passed by value and passes it on to the base class constructor.B) An char operator[](int k) function that throws a BoundsException object if k is negative or is greater than or equal to the length of the string. If k is within the bounds of the string, this function will return the character at position k in the string. You will need to write the definition of the BoundsException class. Test your class with a main function that attempts to access characters that are within and outside the bounds of a suitably initialized BCheckString object.arrow_forwardC++arrow_forwardFix the errors in the Customer class and the Program. #include <iostream>#include <fstream>#include <string>using namespace std; class Customer {// Constructorvoid Customer(string name, string address) : cust_name(name), cust_address(address){acct_number = this.getNextAcctNumber();} // Accessor to get the account numberconst int getAcctNumber() { return acct_number; } // Accessor to get the customer namestring getCustName(} const { return cust_name; } // Accessor to get the customer addressstring getCustAddress() const { return cust_address; } // Set a customer name and addressstatic void set(string name, string address); // Set a customer addressvoid setAddress(string cust_address) { cust_address = cust_address; } // Get the next account number for the next customer.static const unsigned long getNextAcctNumber() { ++nextAcctNum; } // input operatorfriend Customer operator>> (istream& ins, Customer cust); // output operatorfriend void operator<<…arrow_forward
- Computer Science You are required to develop a small chatting application where two or more friends can communicate each other through messages. Create a class Message which has Date d, and message (string). Provide getters/setters, constructors, toString. Create a class Friend having String name, String contact, email and ArrayList of Messages provide getters/setters, constructors, toString addMessage(Message m) method which will add new message to the list. Provide following options to the user using JFrame. Login which will help user login to the application. View Friends (Display List of All Friends) View Messages ( This should display all message of a Friend) Send message (This should ask for friend name and message match the friend name and write that message to the array list).arrow_forwardFill in the blanksarrow_forward1. Use the Python to declare the following classes. Hint: Document is the parent class, and Book and Email are child classes inheriting the document class. Document -authors : String[ ] -date : Date +getAuthors() : String[ ] +addAuthor(name) : void +getDate() : date Вook EMail -title : String -subject : String -to : String[ ] +getTitle() : String +getSubject() : String +getTo() : String[ ] 2. Use O(n) to measure the following formular 10000 + log (n) + n Answer: • n? + nlog (n) + 5000 n Answer: 2 n20 + 2" Answer:arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
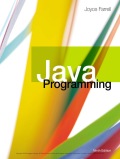
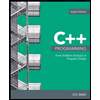
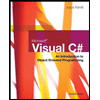