Concept explainers
What will the following
#include <iostream>
#include <memory>
using namespace std;
class Base
{
public:
Base () { cout << "Entering the base .\n"; }
virtual ~Base () { cout << "Leaving the base.\n"; }
} ;
class Camp : public Base
{
public :
Camp() { cout << "Entering the camp.\n"; }
virtual ~Camp() { cout << "Leaving the camp.\n"; }
};
int main()
{
shared _ptr<Camp> outpost = make_shared<Camp> ();
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Software Engineering (10th Edition)
Problem Solving with C++ (10th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Concepts of Programming Languages (11th Edition)
- class Vehicle { private: int wheels; protected : int passenger: public : void inputdata(int, int); void outputdata(); }; class Heavyvehicle : protected Vehicle { int diesel_petrol; protected : int load; public: void readdata(int, int); void writedata(); }; class Bus : private Heavyvehicle char make[20]; public : void fetchdata(char); void displaydata(); (1) Name the base class and derived class of the class Heavyvehicle. (ii) Name the data member(s) that can be accessed from function displaydata(). (iii) Name the data member's that can be accessed by an object of Bus class. (iv) Is the member function outputdata() accessible to the objects of Heavyvehicle class.arrow_forwardC++arrow_forward// volunteer.h #include class Volunteer { public: Volunteer () { } std::string Name () void SetName (const private: std::string name_; }; const { return name_; } std::string& name) { name_ = name; }arrow_forward
- #include <iostream> using namespace std; class Creature { public: Creature(); void run() const; protected: int distance;}; Creature::Creature(): distance(10){} void Creature::run() const{ cout << "running " << distance << " meters!\n";} class Wizard : public Creature { public: Wizard(); void hover() const; private: int distFactor;}; Wizard::Wizard() : distFactor(3){} void Wizard::hover() const{ cout << "hovering " << (distFactor * distance) << " meters!\n";} int main(){ cout << "Creating an Creature.\n"; Creature c; c.run(); cout << "\nCreating a Wizard.\n"; Wizard w; w.run(); w.hover(); return 0;} Reorganize your previous file’s (creature.cpp) content into three parts: declaration, imple-mentation of the classes and your test program. Name the files Creature.h,Creature.cpp and testcreature.cpp.arrow_forward#include <iostream> using namespace std; class Creature { public: Creature(); void run() const; protected: int distance;}; Creature::Creature(): distance(10){} void Creature::run() const{ cout << "running " << distance << " meters!\n";} class Wizard : public Creature { public: Wizard(); void hover() const; private: int distFactor;}; Wizard::Wizard() : distFactor(3){} void Wizard::hover() const{ cout << "hovering " << (distFactor * distance) << " meters!\n";} int main(){ cout << "Creating an Creature.\n"; Creature c; c.run(); cout << "\nCreating a Wizard.\n"; Wizard w; w.run(); w.hover(); return 0;}arrow_forward#include <iostream> using namespace std; class Rectangle { // define class public: Rectangle() { length = 0; width = 0; } // constructor float getlength() { return length; } // return length float getWidth() { return width; } // return width float area() { return length* width; } // return area float perimeter() { return 2 * length + 2 * width; } void setValues(float, float); private: float length, width; }; void Rectangle::setValues(float len, float wid) { if (len <= 0 || len >= 20) { cout << "Enter new value for length "; cin >> len; } length = len; if (wid <= 0 || wid >= 20) { cout << "Enter new value for width "; cin >> wid; } width = wid; } int main() { float length, width; Rectangle r; cout << "Enter a value for length and width between 0 and…arrow_forward
- #include #include using namespace std; class Staff{ public: int code; string name; public: Staff(){} Staff(int code, string name){ this->code = code; this->name = name; } Staff(const Staff &s){ this->code=s.code; this->name = s.name; } int getCode(){ return code; } void setCode(int c){ code = c; } string getName(){ return name; } void setName(string n){ name = n; }; class Faculty: public Staff{ public: int department; string subjectTaken; string researchArea; Faculty(int code,string name,int department, string subjectTaken, string researchArea):Staff(code,name) { this->department = department; this->subjectTaken = subjectTaken; this->researchArea = researchArea; } Faculty() { } Faculty(const Faculty &f){ code = f.code; name = f.name; department = f.department; subjectTaken = f.subjectTaken; researchArea = f.researchArea; } int getDepartment() { return department; } void setDepartment(int department) {…arrow_forwardNonearrow_forwardDate class to use: #ifndef DATE_H_ #define DATE_H_ #include <iostream> #include <iomanip> using namespace std; class Date { friend ostream &operator<<( ostream &, const Date & ); private: int day; int month; int year; static const int days[]; // array of days per month void helpIncrement(); // utility function for incrementing date public: Date(int=1, int=1, int=0); void setDate(int,int,int); bool leapYear( int ) const; // is date in a leap year? bool endOfMonth( int ) const; // is date at the end of month? Date &operator++(); // prefix increment operator Date operator++( int ); // postfix increment operator const Date &operator+=( int ); // add days, modify object bool operator<(const Date&) const; void showdate(); }; const int Date::days[] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 }; Date::Date(int d, int m, int y) { day = d; month = m; year = y; // initialize static member at file scope; one classwide copy } // set month,…arrow_forward
- C++arrow_forwardC++ #include <string>#include <cmath> class DollarAmount {public:// initialize amount from an int64_t valueexplicit DollarAmount(int64_t value) : amount{value} { } // add right's amount to this object's amountvoid add(DollarAmount right) {// can access private data of other objects of the same classamount += right.amount; } // subtract right's amount from this object's amountvoid subtract(DollarAmount right) {// can access private data of other objects of the same classamount -= right.amount;} // uses integer arithmetic to calculate interest amount, // then calls add with the interest amountvoid addInterest(int rate, int divisor) {// create DollarAmount representing the interestDollarAmount interest{(amount * rate + divisor / 2) / divisor}; add(interest); // add interest to this object's amount} // return a string representation of a DollarAmount objectstd::string toString() const {std::string dollars{std::to_string(amount / 100)};std::string…arrow_forwardclass Currency { protected: int whole; int fraction; virtual std::string get_name() = 0; public: Currency() { whole = 0; fraction = 0; } Currency(double value) { if (value < 0) throw "Invalid value"; whole = int(value); fraction = std::round(100 * (value - whole)); } Currency(const Currency& curr) { whole = curr.whole; fraction = curr.fraction; } /* This algorithm gets the whole part or fractional part of the currency Pre: whole, fraction - integer numbers Post: Return: whole or fraction */ int get_whole() { return whole; } int get_fraction() { return fraction; } /* This algorithm adds an object to the same currency Pre: object (same currency) Post: Return: */ void set_whole(int w) { if (w >= 0) whole = w; } void set_fraction(int f) { if (f >= 0 && f <…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
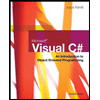