Concept explainers
If a member variable is declared _____, all objects of that class share that variable.

If a member variable is declared as a “static”, then all objects of that class share have access to that member variable.
Explanation of Solution
Static member variable:
In object-oriented programming, the member variable is declared with the keyword of “static” is referred as static member variable.
- In memory, it allocates only one copy of the static member variable for that class.
- If changes are made to static variable, then it reflects to all other instances of that class.
- The static member variables can be re-declared and reuse outside the class using the scope resolution operator (::).
- The static member variables are used anywhere in the program. Even though it is declared outside or inside of the class.
Example:
Consider the example of static member variable declaration is as follows:
//Header file
#include<iostream>
using namespace std;
//class definition
class sample
{
//declaration of instance variable
int c;
//declaration of static variable
static int ct;
//access specifier
public:
//constructor
sample()
{
//increment the variable
c = ++ct;
}
//function definition
void show_code()
{
//display the output
cout << "Object number is: " << c << endl;
}
//static member function definition
static void show_count()
{
//display the output
cout << "The number of objects in the program: " << ct<< endl;
}
};
//definition of static member variable "ct"
int sample::ct=0;
//definition of main method
int main()
{
//Create two Objects for "sample" class
sample o1, o2;
//call the functions
o1.show_count();
o1.show_code();
o2.show_count();
o2.show_code();
//return statement
return 0;
}
Explanation:
- Here, the variable “ct” is declared as a static member variable in the class “sample”.
- In main() function,
- Create two objects for “sample”class.
- Call the show_count() function by using two objects such as “o1” and “o2”.
- Call the show_code() function by using two objects such as “o1” and “o2”.
- Therefore, all the objects of the class have rights to access the static member variable “ct”.
Output:
The number of objects in the program: 2
Object number is: 1
The number of objects in the program: 2
Object number is: 2
Want to see more full solutions like this?
Chapter 11 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Modern Database Management
Starting Out with Programming Logic and Design (4th Edition)
Problem Solving with C++ (10th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- Object composition is useful for creating a(n) _____relationship between two classes.arrow_forwardTrue or False: 1. Data items in a class may be public. 2. Class members are public by default. 3. Friend functions have access only to public members of the class.arrow_forwardThe _____ key word prevents member functions from being overridden in a derived class. which one: final virtual virtual void lastarrow_forward
- Class members specified as________ are accessible only to member functions of the class and friend s of the class.arrow_forwardT/F: Instance variables are shared by all the instances of the class. T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class. T/F: To declare static variables, constants, and methods, use the static modifier.arrow_forwardDescribe the processes involved in making a class's member variable static in programming terms.arrow_forward
- Employee and ProductionWorker ClassesCreate an Employee class that has properties for the following data:Employee nameEmployee numberNext, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data:Shift number (an integer, such as 1, 2, or 3)Hourly pay rateThe workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2.Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values. this.ReportViewer1.Employee(); cannot reference?arrow_forwardCar Class in C++ language whole task is in the picturearrow_forwardThis type of member function may be called from a function that is a member of the same class or a derived class. static private protected O None of thesearrow_forward
- A constructor is a special kind of member function. It is automatically called when an object of that class is declared.t or f?arrow_forwardTrue/False: a member function in a class can access all of its class's member variables, but not if the variables are private. A) True B) Falsearrow_forwardA constructor that takes a single parameter of a type different from the class type is a_________ constructor.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
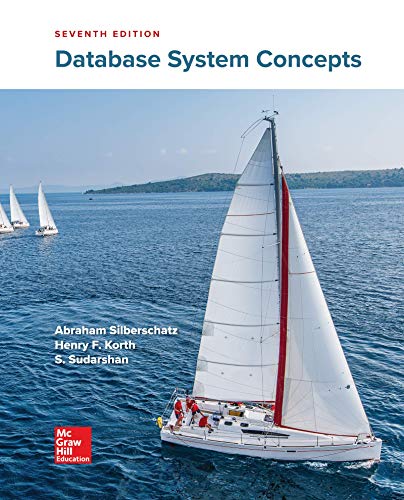
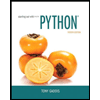
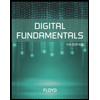
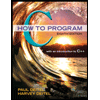
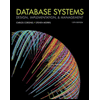
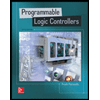