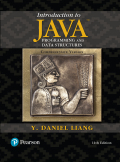
Concept explainers
Sections 11.2–11.4
11.1 (The Triangle class) Design a class named Triangle that extends GeometricObject. The class contains:
■ Three double data fields named side1, side2, and side3 with default values 1.0 to denote three sides of a triangle.
■ A no-arg constructor that creates a default triangle.
■ A constructor that creates a triangle with the specified side1, side2, and side3.
■ The accessor methods for all three data fields.
■ A method named getArea() that returns the area of this triangle.
■ A method named getPerimeter() that returns the perimeter of this triangle.
■ A method named toString() that returns a string description for the triangle.
For the formula to compute the area of a triangle, see
return "Triangle: side1 = " + side1 + " side2 = " + side2 + " side3 = " + side3;
Draw the UML diagrams for the classes Triangle and GeometricObject and implement the classes. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.

Program Plan:
- Include the required import statement.
- Define the main class.
- Define the main method using public static main.
- Declare the input scanner.
- Get the three sides of the triangle from the user.
- Create an object for the “Triangle” class.
- Get the color from the user and call the “setColor” method with the parameter “color”.
- Get the Boolean value for filled the triangle and call the “setFilled” method with the parameter “filled”.
- Display the output.
- Define the main method using public static main.
- Define the “GeometricObject” class.
- Declare the required variables.
- Define the default constructor and constructor for the class.
- Define the accessor and matator.
- The “isFilled” and “setColor” method will return the value to the main class.
- Define the derived class “Triangle” from the “GeometricObject” class.
- Declare the required variables.
- Define the default constructor and constructor for the class.
- Define the accessor.
- The “getArea()” method will calculate the area of triangle and then return the result.
- The “getPerimeter()” method will return the perimeter of the triangle.
- The “toString()” method will return the three sides of the triangle.
The below program is used to display the area, perimeter and sides of the triangle as follows:
Explanation of Solution
Program:
//import statement
import java.util.Scanner;
//class Excersise11_01
public class Exercise11_01
{
// main method
public static void main(String[] args)
{
// declare the scanner variable
Scanner input = new Scanner(System.in);
//get the input from the user
System.out.print("Enter three sides: ");
//declare the variables
double side1 = input.nextDouble();
double side2 = input.nextDouble();
double side3 = input.nextDouble();
//create an object for the "Triangle" class
Triangle triangle = new Triangle(side1, side2, side3);
//get the input from the user
System.out.print("Enter the color: ");
String color = input.next();
//call the "setColor" function
triangle.setColor(color);
//get the input from the user
System.out.print("Enter a boolean value for filled: ");
boolean filled = input.nextBoolean();
//call the "setFilled" function
triangle.setFilled(filled);
//print the output
System.out.println("The area is " + triangle.getArea());
System.out.println("The perimeter is "
+ triangle.getPerimeter());
System.out.println(triangle);
}
}
//definition of class "GeometricObject"
class GeometricObject
{
/* declare the required variables and initialize it */
private String color = "white";
private boolean filled;
private java.util.Date dateCreated;
//definition of default constructor
public GeometricObject()
{
//create an object
dateCreated = new java.util.Date();
}
//definition of constructor
public GeometricObject(String color, boolean filled)
{
//create an object
dateCreated = new java.util.Date();
//set the value
this.color = color;
this.filled = filled;
}
//definition of accessor
public String getColor()
{
//return the color
return color;
}
//definition of mutator
public void setColor (String color)
{
//set the color
this.color = color;
}
//definition of the "isFilled" method
public boolean isFilled()
{
//return the value
return filled;
}
//definition of the "setFilled" method
public void setFilled(boolean filled)
{
//set the value
this.filled = filled;
}
//definition of the "getDateCreated" method
public java.util.Date getDateCreated()
{
//return the value
return dateCreated;
}
//definition of the "toString" method
public String toString()
{
//return the value
return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled;
}
}
//definition of derived class "Triangle"
class Triangle extends GeometricObject
{
/* declare the required variables and initialize it */
private double side1 = 1.0, side2 = 1.0, side3 = 1.0;
/*definition of Constructor */
public Triangle()
{
}
/* definition of Constructor */
public Triangle(double side1, double side2, double side3)
{
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
//definition of accessor
public double getSide1()
{
//return the value
return side1;
}
//definition of accessor
public double getSide2()
{
//return the value
return side2;
}
//definition of accessor
public double getSide3()
{
//return the value
return side3;
}
/*override method of "getArea" in GeometricObject */
public double getArea()
{
//declare and calculate the value
double s = (side1 + side2 + side3) / 2;
//return the value
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
/*override method of "getPerimeter" in GeometricObject */
public double getPerimeter()
{
//return the value
return side1 + side2 + side3;
}
//definition of "toString" method
public String toString()
{
// return the three sides
return "Triangle: side1 = " + side1 + " side2 = " + side2 +" side3 = " + side3;
}
}
UML diagram:
Explanation:
The above UML diagram the “GeometricObject” is a parent class which contains “color”, “filled”, “dateCreated” variables and “GeometricObject()”, “GeometricObject(String color, boolean filled)”, “getColor()”, “getColor(String color)”, “isFilled()”, “setFilled(boolean filled)”, “getDateCreated()” and “toString()” methods.
The “Triangle” is the child class extended from “GeometricObject” class it contains “side1”, “side2” and “side3” variables and “Triangle()”, “Triangle(double side1, double side2, double side3)”, “getSide1()”, “getSide2()”, “getSide3()”, “getArea()”, “getPerimeter()” and “toString()” methods.
Enter three sides: 2
3
4
Enter the color: black
Enter a boolean value for filled: true
The area is 2.9047375096555625
The perimeter is 9.0
Triangle: side1 = 2.0 side2 = 3.0 side3 = 4.0
Want to see more full solutions like this?
Chapter 11 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with Python (4th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forward
- You are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forwardConsider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
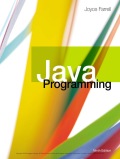
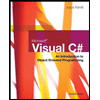
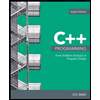
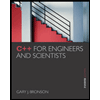