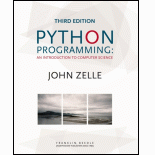
Three Button Monte
Program Plan:
- Import the required packages.
- Declare a main function. Inside the main function,
- Create the application window.
- Draw the interface widget by creating three buttons.
- Assign the text to the interface.
- Call the method “draw()”.
- Get the random number.
- Assign the values to “false”.
- Check the condition.
- Assign “point1” to “true”.
- Check the condition.
- Assign the “point2” to “true”.
- Otherwise, Assign the “point3” to “true”
- Activate all the three doors.
- Get the action mouse clicked.
- Check the condition using “while” loop.
- Check the condition for selecting the door 1 to be clicked.
- Check the condition for selecting the door 2 to be clicked.
- Check the condition for selecting the door 3 to be clicked.
- Call the “getMouse()” function
- Call the main function.

Explanation of Solution
Program:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py:
#Import the required packages
from button import Button
from graphics import GraphWin, Point, Text
from random import random
#Definition of main method
def main():
#Creating the application window by setting title, cords and background
win = GraphWin("Three Button Monte", 500, 300)
win.setCoords(-12, -12, 12, 12)
win.setBackground("green3")
#Draw the interface widget by creating three button
door1 = Button(win, Point(-7.5, -3), 5, 6, "Door 1")
door2 = Button(win, Point(0, -3), 5, 6, "Door 2")
door3 = Button(win, Point(7.5, -3), 5, 6, "Door 3")
#Assign the text to the interface
direction = Text(Point(0, 10), "Pick a Door")
#Call the method draw
direction.draw(win)
#Get the random number
x = random() * 3
#Assign the values to false
point1 = point2 = point3 = False
#check the condition
if 0 <= x <1:
#Assign point1 to True
point1 = True
#Chcek the condition
elif 1 <= x < 2:
#Assign the point2 to True
point2 = True
#Otherwise
else:
#Assign the point3 to True
point3 = True
#Activate all the three doors
door1.activate()
door2.activate()
door3.activate()
#Get the action mouse click
click = win.getMouse()
#Check the condition using "while" loop
while door1.clicked(click) or door2.clicked(click) or door3.clicked(click):
#If door1 is clicked
if door1.clicked(click):
#Assign point1 to true
if point1 == True:
#Update the interface
door1.update(win,"Victory!")
else:
#If door1 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door3.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#If door2 is clicked
elif door2.clicked(click):
#Assign point2 to true
if point2 == True:
#Update the interface
door2.update(win,"Victory!")
else:
#If door2 is clicked
if door3.clicked(click):
#Update the interface
door3.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
else:
#Assign point3 to true
if point3 == True:
#Update the interface
door3.update(win,"Victory!")
else:
#If door2 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#Call the getMouse()
win.getMouse()
#Call the main function
main()
Output:
Screenshot of output
Screenshot of output
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
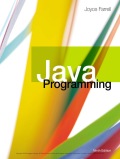
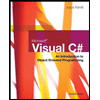
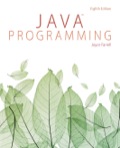
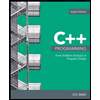
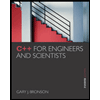