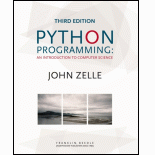
Concept explainers
Three Button Monte
Program Plan:
- Import the required packages.
- Declare a gameplay function. Inside the function,
- Create the application window using the “button.py” module.
- Activate the doors.
- Get the random number.
- Assign the values to “false”.
- Check the condition.
- Assign “point1” to “true”.
- Check the condition.
- Assign the “point2” to “true”.
- Otherwise, Assign the “point3” to “true”
- Activate all the three doors.
- Get the action mouse clicked.
- Check the condition using “while” loop.
- Check the condition for selecting the door 1 to be clicked.
- Check the condition for selecting the door 2 to be clicked.
- Check the condition for selecting the door 3 to be clicked.
- Definition of “printSummary()”.
- Print the result.
- Definition of main function.
- Creating the application window by setting title, cords and background.
- Define “Quit” button and set to active.
- Assign the text to the interface.
- Call the method draw.
- Initialize variables to enter loop
- Check the condition for “Quit” not clicked.
- Call the method “gameplay()”
- Call the method “printSummary()”
- Call the main function.

Explanation of Solution
Program:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py:
#Import required packages
from button import Button
from graphics import GraphWin, Point, Text
from random import random
from time import sleep
#Definition of gameplay method
def gameplay(click, win, wins, losses):
#use button.py module to create doors and activate them
door1 = Button(win, Point(-7.5, -3), 5, 6, "Door 1")
door2 = Button(win, Point(0, -3), 5, 6, "Door 2")
door3 = Button(win, Point(7.5, -3), 5, 6, "Door 3")
#Activate the doors
door1.activate()
door2.activate()
door3.activate()
#Get the random number
x = random() * 3
#Assign the values to false
point1 = point2 = point3 = False
#check the condition
if 0 <= x <1:
#Assign point1 to True
point1 = True
#Check the condition
elif 1 <= x < 2:
#Assign the point2 to True
point2 = True
#Otherwise
else:
#Assign the point2 to True
point3 = True
#Get the action mouse click
click = win.getMouse()
#Check the condition using "while" loop
while door1.clicked(click) or door2.clicked(click) or door3.clicked(click):
#If door1 is clicked
if door1.clicked(click):
#Assign point1 to true
if point1 == True:
#Update the interface
door1.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door1.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the losses
losses = losses + 1
break
#Check the door2 is clicked
elif door2.clicked(click):
#Assign point2 to true
if point2 == True:
#Update the interface
door2.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door2.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the value of losses
losses = losses + 1
break
else:
if point3 == True:
door3.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door3.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the value of losses
losses = losses + 1
break
#Return the values
return click, wins, losses
#Definition of printSummary
def printSummary(wins, losses):
#Print the result
print("Wins: {0:5} Losses: {1:5}".format(wins, losses))
#Definition of main
def main():
#Creating the application window by setting title, cords and background
win = GraphWin("Three Button Monte", 500, 300)
win.setCoords(-12, -12, 12, 12)
win.setBackground("green3")
#define Quit button and set to active
gameover = Button(win, Point(9, 10), 3, 3, "Quit")
gameover.activate()
#Assign the text to the interface
direction = Text(Point(0, 10), "Pick a Door")
#Call the method draw
direction.draw(win)
#initialize variables to enter loop
click = Point(0,0)
wins = losses = 0
#Check the condition
while not gameover.clicked(click):
#Call the method
click, wins, losses = gameplay(click, win, wins, losses)
#Call the method printSummary
printSummary(wins, losses)
#Call the main function
main()
Output:
Screenshot of output
Clicking the “Exit” button:
Clicking the “Door1”, “Door2”, “Door3” will displays the opened door leads to “Victory!” or “Try Again”. After clicking the “Exit” button it prints the count of the corresponding result.
Wins: 1 Losses: 2
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science
- Can you please solve this. Thanksarrow_forwardcan you solve this pleasearrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forward
- In the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardSend me the lexer and parserarrow_forwardHere is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forward
- Create 2 charts using this data. One without using wind speed and one including max speed in mph. Write a Report and a short report explaining your visualizations and design decisions. Include the following: Lead Story: Identify the key story or insight based on your visualizations. Shaffer’s 4C Framework: Describe how you applied Shaffer’s 4C principles in the design of your charts. External Data Integration: Explain the second data and how you integrated it with the Halloween dataset. Compare the two datasets. Attach screenshots of the two charts (Bar graph or Line graph) The Shaffer 4 C’s of Data Visualization Clear - easily seen; sharply defined• who's the audience? what's the message? clarity more important than aestheticsClean - thorough; complete; unadulterated, labels, axis, gridlines, formatting, right chart type, colorchoice, etc.Concise - brief but comprehensive. not minimalist but not verboseCaptivating - to attract and hold by beauty or excellence does it capture…arrow_forwardHow can I resolve the following issue?arrow_forwardI need help to resolve, thank you.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
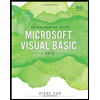
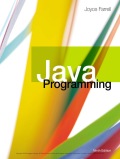
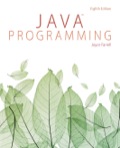
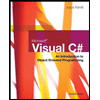