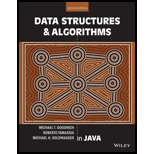
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781119278023
Author: Michael T. Goodrich; Roberto Tamassia; Michael H. Goldwasser
Publisher: Wiley Global Education US
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 1, Problem 9R
Program Plan Intro
Removing all the punctuations from a string
Program plan:
- Create a class StringBuilder to remove all the punctuation stored in a string.
- In main() function,
- Initially, assign the original string in variable.
- Then, invoke the removeIt()function to remove all the punctuation in a string and store the final value in original string.
- Display the string after removing the punctuation in console screen.
- In the method removeAt(),
- It passes input parameter “s” to check whether the character contains punctuation in the string. If yes, then it removes all the punctuation stored in the string “s”.
- For loop read all the characters from a sentence until the condition leads to false.
- Then, it calls the function ok() and check whether the character at “j” position matches with the character or not.
- If the result returns the Boolean value false, then delete the character at the specified position in “j” otherwise store the character using Tostring() method in “s” variable.
- In the method ok(),
- It passes input parameter “i” to check whether the character in the sentence matches with the corresponding ASCII values.
- If yes, then it returns the Boolean value true otherwise it return false.
- It passes input parameter “i” to check whether the character in the sentence matches with the corresponding ASCII values.
- In main() function,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
need help with thi
Next, you are going to combine everything you've learned about HTML and CSS to make a static site portfolio piece.
The page should first introduce yourself. The content is up to you, but should include a variety of HTML elements, not just text.
This should be followed by an online (HTML-ified) version of your CV (Resume).
The following is a minimum list of requirements you should have across all your content:
Both pages should start with a CSS reset (imported into your CSS, not included in your HTML)
Semantic use of HTML5 sectioning elements for page structure
A variety other semantic HTML elements
Meaningful use of Grid, Flexbox and the Box Model as appropriate for different layout components
A table
An image
Good use of CSS Custom Properties (variables)
Non-trivial use of CSS animation
Use of pseudeo elements
An accessible colour palette
Use of media queries
The focus of this course is development, not design. However, being able to replicate a provided design…
Using the notation
you can select multipy options
Chapter 1 Solutions
Data Structures and Algorithms in Java
Ch. 1 - Prob. 1RCh. 1 - Suppose that we create an array A of GameEntry...Ch. 1 - Write a short Java method, isMultiple, that takes...Ch. 1 - Write a short Java method, isEven, that takes an...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that counts the number...Ch. 1 - Prob. 9RCh. 1 - Prob. 10R
Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Prob. 12RCh. 1 - Modify the declaration of the first for loop in...Ch. 1 - Prob. 14CCh. 1 - Write a pseudocode description of a method for...Ch. 1 - Write a short program that takes as input three...Ch. 1 - Write a short Java method that takes an array of...Ch. 1 - Prob. 18CCh. 1 - Write a Java program that can take a positive...Ch. 1 - Write a Java method that takes an array of float...Ch. 1 - Write a Java method that takes an array containing...Ch. 1 - Prob. 22CCh. 1 - Write a short Java program that takes two arrays a...Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Modify the CreditCard class to add a toString()...Ch. 1 - Write a short Java program that takes all the...Ch. 1 - Write a Java program that can simulate a simple...Ch. 1 - A common punishment for school children is to...Ch. 1 - The birthday paradox says that the probability...Ch. 1 - (For those who know Java graphical user interface...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forwardPlease help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forward
- What does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forwardPlease assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forwardneed help with a html code and css code that will match this image.arrow_forward
- need help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forwardWhy all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forward
- Why is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forwardWrite the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of services that can (also)be initiated face-to-face, and it will provide managed, private, secure access to a repository ofpublic and/or personal information. Ideally, the database will have basic details of pensionplans recorded in the registry, member plan statistics, and cash inflows and outflows frompension funds.For example, insured persons accumulate contributions. Records for these persons will includeinformation on the insured persons able to acquire various benefits once work is interrupteddue to sickness, death, retirement, and maternity or employment injury. They will also includeinformation on pensions such as invalidity, disability, and survivors that stem from…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
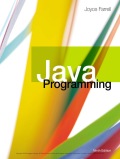
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
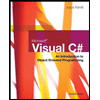
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
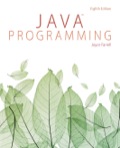
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
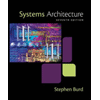
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
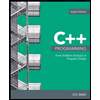
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning