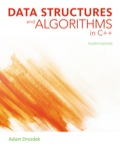
Concept explainers
a)
The content of array is been initialized with given values and “*p” is assigned to the beginning of the array, now after executing each instructions individually results are obtained given below.
Explanation of Solution
Explanantion:
In the above program , an integer array is been declared first. A pointer “p” points to array. The pointer “p” is been incremented to obtain second element of integer array. Finally,program displays contents of array and value of “p”
Therefore, the content of “intArray” will be
Explanation of Solution
b)
(*p)++;
The integer pointer “p” points to first elemenst of the array. So, “*(p)++” denotes that first element of array is been incremented first and then it is assigned to “*p”. Hence, first element of array increments as a result of this instruction.
This program shows execution of the construct and displays the result of the array and value of “p”.
Program:
//Select header files
#include <stdio.h>
#include<iostream>
using namespace std;
// Main function
int main(void)
{
// Integer array with declaration
int arr[] = {1,2,3};
Here, “p” denotes pointer to integer array...
Explanation of Solution
Explanantion:
In the above program an integer array is declared first. A pointer “p” points to array. The content pointed to by “p” is been incremented . Finally program displays contents of array and value of “p”.
Therefore, the content of “intArray” will be
Explanation of Solution
c)
*p++; (*p)++;
The integer pointer “p” points to first element of array. So, *p++ denotes second element of array. Hence, second element of array increments and the incremented value is stored in “p”.
This program shows the execution of the construct and displays result of array and value of “p”.
Program:
//Select header files
#include <stdio.h>
#include<iostream>
using namespace std;
// Main function
int main(void)
{
// Integer array with declaration
int arr[] = {1,2,3};
...Explanation of Solution
Explanantion:
In the above program an integer array is declared first. A pointer “p” points to array. The pointer is incremented, so that it now points to second element of array . Now, the contents pointed to by “p” is been incremented. Finally the program displays contents of array and value of “p”
Therefore, the content of “intArray” will be

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- b) Consider a program component Binary_Search (list, searched_string) which search a string in an array of maximum 100000 elements. i) What are test cases you would like to test this procedure based on equivalence classes? ii) What would be additional test cases based on boundary values.arrow_forwardWrite a C++ code for a function that creates a one-dimensional dynamic array to emulate a two-dimensional array and returns a pointer to the one-dimensional dynamic array. int* EmulatedTwoDimensionalArray(int DesiredRows, int DesiredColumns). Therefore, int ptr=EmulatedTwoDimensionalArray(4,6); would be the same as int ptr[4][6]; Also, write a driver to test this function.arrow_forwardCode: #include <bits/stdc++.h> using namespace std; void BUBBLE(int A[],int N){ for(int k=0;k<N-1;++k){ for(int ptr=0;ptr<N-k-1;++ptr){ if(A[ptr]>A[ptr+1]){ int temp = A[ptr]; A[ptr] = A[ptr+1]; A[ptr+1]=temp; } } }} //function to print the arrayvoid printArray(int arr[],int n){ int i; for(i=0;i<n;i++) cout<<arr[i]<<" "; cout<<endl;} //driver function to test the modulesint main(){ int arr[] ={15,17,5,3,25,66,14,7,59,100}; int n=sizeof(arr)/sizeof(arr[0]); cout<<"\nOriginal array: "; printArray(arr,n); cout<<"\n\nOutput of Bubble sort are shown below:\n"; BUBBLE(arr,n); printArray(arr, n); return 0;} Q: Remove the Function from the above codearrow_forward
- function myCompose(f,g){// TODO: return (f o g);// that is, a function that returns f(g(x)) when invoked on x.}arrow_forwardQUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forwardIn C++ The function below, zeroesToFives takes an array of integers and changes any zeroes in the array to fives and returns the count of array elements that were updated. Write at least ten test cases using assertions for zeroesToFives. You should test at least three boundary/edge cases.arrow_forward
- void deleteRange( int from, int to) { int i, j = 0; for (i = 0; i < counter; i++) { if (i <= from - 1 || i >= to + 1) { A[j] = A[i]; j++; } } for (int i = 0; i < j; i++) cout << A[i] << " "; } Above method deletes range of elements from an array. Please explain the logic of above code in simple english (algorithm and comments).arrow_forwardAn array is a container object that holds a fixed number of values of a single type. To create an array in C, we can do int arr[n];. Here, arr, is a variable array which holds up to integers. The above array is a static array that has memory allocated at compile time. A dynamic array can be created in C, using the malloc function and the memory is allocated on the heap at runtime. To create an integer array, of size , int *arr = (int*)malloc(n * sizeof(int)), where points to the base address of the array. When you have finished with the array, use free(arr) to deallocate the memory. In this challenge, create an array of size dynamically, and read the values from stdin. Iterate the array calculating the sum of all elements. Print the sum and free the memory where the array is stored. While it is true that you can sum the elements as they are read, without first storing them to an array, but you will not get the experience working with an array. Efficiency will be required later.…arrow_forwardExplain this C code line per line please #include <stdio.h> #include <malloc.h> void printArray(int**, int); int main() { int i = 0, j = 0, n = 5; int **arr = (int**)malloc(n * sizeof(int*)); for(int i=0;i<n;i++){ *(arr+i) = (int*)malloc(n*sizeof(int)); } for(i=0;i<n;i++){ for(int j=0;j<n;j++){ *(*(arr+i) + j) = 0; } } printArray(arr, n); for(i=0;i<n;i++){ *( *(arr + i) + i) = i+1; } printArray(arr,n); return 0; } void printArray(int ** array, int size) { int i,j; for(i = 0;i<size;i++){ for(j=0;j<size;j++){ printf("%d ", *( *(array + i) + j)); } printf("\n"); } printf("\n"); }arrow_forward
- void deleteRange( int from, int to) {int i, j = 0;for (i = 0; i < counter; i++) {if (i <= from - 1 || i >= to + 1) {A[j] = A[i];j++;}}for (int i = 0; i < j; i++)cout << A[i] << " ";} Above method deletes range of elements from an array. Consider array A[] globally declared and counter is its size. Please explain the logic of above code in simple english (algorithm and comments).arrow_forward1. Trace the execution of the following: {0, 1, 2, 3, 4, 5, 6, 7}; int[] anArray for (int i = 3; i 3; i--) 1]; What are the contents of anArray after the execution of each loop?arrow_forwardImplement in C Programming 8.2.2: Printing with pointers. If the input is negative, make numItemsPointer be null. Otherwise, make numItemsPointer point to numItems and multiply the value to which numItemsPointer points by 10. Ex: If the user enters 99, the output should be:Items: 990 #include <stdio.h> int main(void) { int* numItemsPointer; int numItems; scanf("%d", &numItems); /* Your solution goes here */ if (numItemsPointer == NULL) { printf("Items is negative\n"); } else { printf("Items: %d\n", *numItemsPointer); } return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
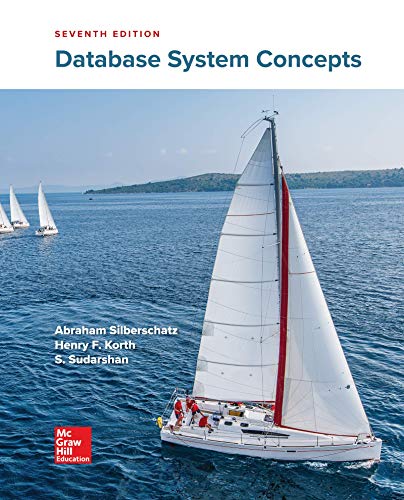
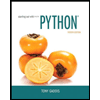
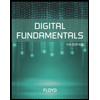
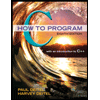
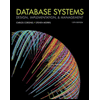
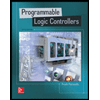