You are helping a small business to collect and calculate weekly pay for its employees. Your java program will prompt the user for employee name, hours worked and hourly rate for 10 of its employees. Then your program will provide a way to search for an employee name and display the employe information and calculated weekly pay. The program has been partially created for you. You will just have to fill in the missing part. Use the code below and complete the program. Step 1 and Step 2 are already done. You will complete Step 3 and Step 4.. Note: Use Step 2 code to model your code for Step 3 Note: Use the instructions given in method displayEmployeePay to complete step 4 import javax.swing.JOptionPane; public class Lab1 { public static void main(String[] args) { final int NUM_EMPLOYEES = 10; //Intialize 3 arrays to store employee names, hoursWorked and their hourlyRates. String[] names = new String[NUM_EMPLOYEES]; double[] hoursWorked = new double[NUM_EMPLOYEES]; double[] hourlyRates = new double[NUM_EMPLOYEES]; //Step 1 : fill/populate names array from user input //Lab instruction: You do not need to write this method; it is done for you populateNames(names); //Step2 : fill/populate horur
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
You are helping a small business to collect and calculate weekly pay for its employees. Your java program will prompt the user for employee name, hours worked and hourly rate for 10 of its employees. Then your program will provide a way to search for an employee name and display the employe information and calculated weekly pay.
The program has been partially created for you. You will just have to fill in the missing part. Use the code below and complete the program.
Step 1 and Step 2 are already done.
You will complete Step 3 and Step 4..
Note: Use Step 2 code to model your code for Step 3
Note: Use the instructions given in method displayEmployeePay to complete step 4
import javax.swing.JOptionPane;
public class Lab1 {
public static void main(String[] args) {
final int NUM_EMPLOYEES = 10;
//Intialize 3 arrays to store employee names, hoursWorked and their hourlyRates.
String[] names = new String[NUM_EMPLOYEES];
double[] hoursWorked = new double[NUM_EMPLOYEES];
double[] hourlyRates = new double[NUM_EMPLOYEES];
//Step 1 : fill/populate names array from user input
//Lab instruction: You do not need to write this method; it is done for you
populateNames(names);
//Step2 : fill/populate horursWorked array from user input
//Lab instruction: You do not need to write this method; it is done for you
populateHoursWorked(hoursWorked);
//Step 3 (Student to complete) : fill/populate scores array from user input
//Note: You need to write this method and all subsequent methods that this method will call.
// follow the code provided in Step 2 to complete this.
// Note: Hourly rate must be greater than 0 and cannot exceed 100.00
populateHourlyRate(hourlyRates);
//Step 4: (Student to Complete) create a method that will prompt the user for a name and will display the employee name, hours worked, his/her hourly rate and pay.
// The pay is calculated by multiplying hours worked by hourly rate. i.e. hoursWorked * hourlyRate
// Sample output of this method:
// Name - Hours Worked - hourly Rate
// John Smith - 38 - $45.95
// Total Pay : $1746.10
// Lab Instruction: you need to create this mathod and all subsequent methods that this method will call
displayEmployeePay(names,hoursWorked,hourlyRates);
}
public static void populateNames(String[] names) {
//Use for loop to fill array from user input one employee at a time
for (int i=0; i < names.length; i++){
names[i] = getEmployeeName();
}
}
public static String getEmployeeName() {
boolean isValid = true;
String employeeName;
do {
isValid = true;
employeeName = JOptionPane.showInputDialog("Enter Employee Name");
if (employeeName.length() == 0 || employeeName.equals("")){
JOptionPane.showMessageDialog(null,"Employee Name cannot be empty.");
isValid = false;
}
}while (isValid == false);
return employeeName;
}
public static void populateHoursWorked(double[] hoursWorked) {
//Use for loop to fill array from user input one employee at a time
for (int i=0; i < hoursWorked.length; i++){
hoursWorked[i] = getEmployeeHoursWorked();
}
}
public static double getEmployeeHoursWorked() {
double hoursWorked = 0.0;
boolean isValid = true;
do {
try {
isValid = true;
String input = JOptionPane.showInputDialog("Enter Hours Worked (0 - 40):");
hoursWorked = Double.parseDouble(input);
if (!(hoursWorked >= 0 && hoursWorked <= 40)) {
JOptionPane.showMessageDialog(null, "Hours Worked must be between 0 and 40 hours.");
isValid = false;
hoursWorked = 0.0;
}
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, "Invalid input. Please enter a valid number.");
isValid = false;
}
}while (isValid == false);
return hoursWorked;
}
public static void populateHourlyRate(double[] hourlyRates) {
//write you code here - follow methods to populate hours worked above
}
public static double getEmployeeHourlyRate() {
double hourlyRate = 0.0;
//write you code here - follow methods to populate hours worked above
//Hourly rate must be greater than 0 and cannot exceed 100.00
return hourlyRate;
}
public static void displayEmployeePay(String[] names, double[] hoursWorked, double[] hourlyRates) {

Step by step
Solved in 4 steps with 3 images

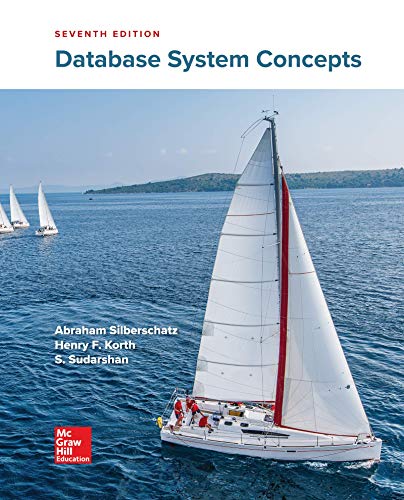
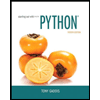
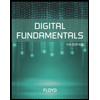
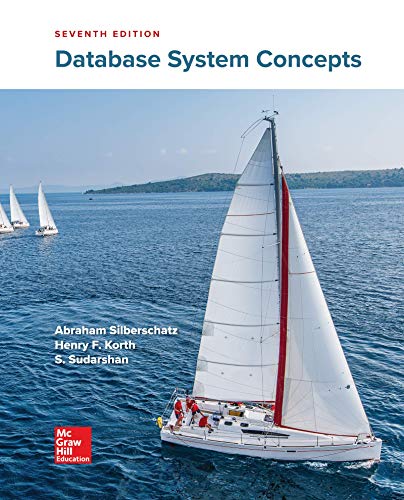
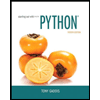
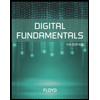
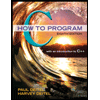
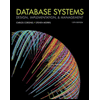
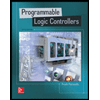