First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects). Then create a new Java application called "RecursiveTriangle" (without the quotation marks) according to the following guidelines. Modify the example below so that the triangle displays “in reverse order”, which allows the user to set the number of lines to print and the String used for printing the triangle. public static void printTriangle(int sideLength) { // quits if sidelength is at 0 or smaller if (sideLength < 1) { return; } // recursion - method is calling itself printTriangle(sideLength - 1); // main loop that prints out the body of the triangle for (int i = 0; i < sideLength; i ++) { System.out.println("[]"); } System.out.println(); } Use a method to prompt the user for the number of lines (between 1 and 10) to print. This method should take no parameters and return an int value. Use a method to prompt the user for the string to be used when printing the pattern. This method should take no parameters and return a String value. Use a method called printTriangle that takes an int and a String as parameters (i.e., the return values from the two methods mentioned above) and has no return value. The use of recursion is OPTIONAL. If you want to challenge yourself - try it with recursion. EXAMPLE: The call to printTriangle(numLines, pattern) should print the following, assuming numLines has the value 4 and pattern has the value “[]”. [][][][] [][][] [][] []
First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects).
Then create a new Java application called "RecursiveTriangle" (without the quotation marks) according to the following guidelines.
- Modify the example below so that the triangle displays “in reverse order”, which allows the user to set the number of lines to print and the String used for printing the triangle.
public static void printTriangle(int sideLength)
{
// quits if sidelength is at 0 or smaller
if (sideLength < 1) { return; }
// recursion - method is calling itself
printTriangle(sideLength - 1);
// main loop that prints out the body of the triangle
for (int i = 0; i < sideLength; i ++)
{
System.out.println("[]");
}
System.out.println();
}
- Use a method to prompt the user for the number of lines (between 1 and 10) to print. This method should take no parameters and return an int value.
- Use a method to prompt the user for the string to be used when printing the pattern. This method should take no parameters and return a String value.
- Use a method called printTriangle that takes an int and a String as parameters (i.e., the return values from the two methods mentioned above) and has no return value. The use of recursion is OPTIONAL. If you want to challenge yourself - try it with recursion.
EXAMPLE: The call to printTriangle(numLines, pattern) should print the following, assuming numLines has the value 4 and pattern has the value “[]”.
[][][][]
[][][]
[][]
[]

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

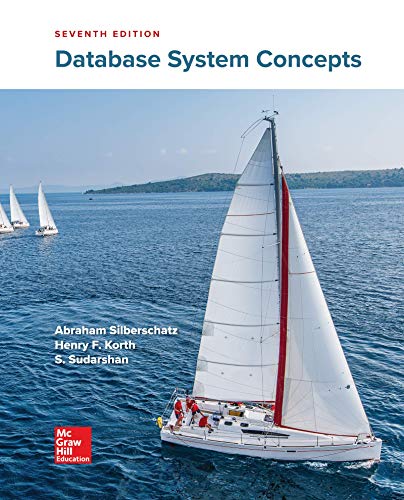
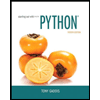
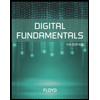
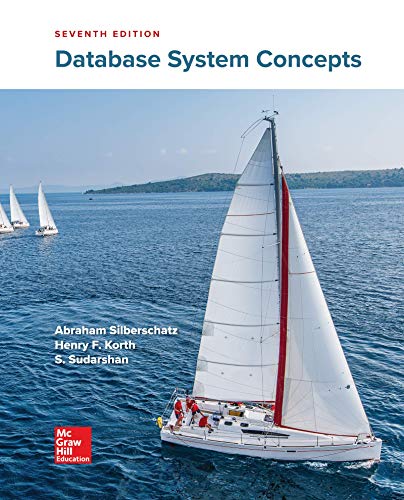
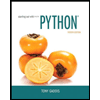
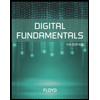
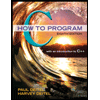
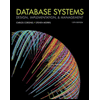
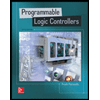