Write C++ code for the following programming exercises. (All inputs must be done in main(), and all four functions must be called from main()) Unfortunately I can't use more "advanced" coding techniques. I have to use functions, so I've included a sample of what the code should look similar to. I'm stuck on this and any help is much appreciated.
Write C++ code for the following programming exercises. (All inputs must be done in main(), and all four functions must be called from main()) Unfortunately I can't use more "advanced" coding techniques. I have to use functions, so I've included a sample of what the code should look similar to. I'm stuck on this and any help is much appreciated.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write C++ code for the following
Unfortunately I can't use more "advanced" coding techniques. I have to use functions, so I've included a sample of what the code should look similar to. I'm stuck on this and any help is much appreciated.

Transcribed Image Text:The following formula gives the distance between two points, (x1, Y1)
and (x2, y2) in the Cartesian plane:
11.
V(x2 – x1 )² + (y2 – yı)?
Given the center and a point on the circle, you can use this formula to
find the radius of the circle. Write a program that prompts the user to
enter the center and a point on the circle. The program should then
output the circle's radius, diameter, circumference, and area. Your pro-
gram must have at least the following functions:
distance: This function takes as its parameters four numbers that rep-
resent two points in the plane and returns the distance between them.
a.
radius: This function takes as its parameters four numbers that
represent the center and a point on the circle, calls the function
distance to find the radius of the circle, and returns the circle's radius.
b.
circumference: This function takes as its parameter a number
that represents the radius of the circle and returns the circle's cir-
cumference. (If r is the radius, the circumference is 2tr.)
C.
area: This function takes as its parameter a number that repre-
sents the radius of the circle and returns the circle's area. (If r is the
radius, the area is Tr².)
d.
Assume that t =
3.1416.

Transcribed Image Text:FSyn
1
#include <iostream>
es
#include<conio.h>
#include <cmath>
2
I
4
using namespace std;
6.
void getHoursRate (double *p,double *q);
double payCheck (double p, double q);
void printCheck (double p, double q, double r);
double distance (double x1, double x2, double y1, double y2)
7
Intel
8.
PerfLogs
9
Program File
Program File
10 E{
return (sqrt (pow (x2-x1,2) + pow(y2-y1,2)));
}
11
12
Users
13
double radius (double x1, double x2, double y1, double y2)
Windows
14
15
return distance (x1, x2, y1, y2);
16
}
17
double circumference (double r)
18 E{
19
return (2*PI*r);
20
}
21
double area (double r)
22 E{
23
return (PI*pow (r,2));
24
25
int main ()
26 E{
27
|//Program 1
28
double x1, y1, x2, y2, PI = 3.14159;
cout << "Enter center of circle: ";
cin >> x1 >> y1;
29
30
// read center of circle (x, y)
cout << "Enter point on circle: ";
cin >> x2 >> y2;
31
// read point on circle (x, y)
x1, 2) + pow (y2 -
32
33
double r =
sqrt (pow (x2
y1, 2)); // find radius using given formula
34
35
cout << "Radius is
<< r << endl;
// print radius
Logs & others
CppCheck/Vera++ X CppCheck/Vera++ messages X
Cscope X
Debugger X
DoxyBlocks X
F Fortran info X
Closed files list X
Thread search X
Build messages X
F... L.. Message
=== Build file: "no target" in "no project" (compiler: unknown)
In function 'double circumference (double)':
===
C...
C... 19
error:
'PI' was not declared in this scope
C...
In function 'double area (double)':
C... 23
error:
'PI' was not declared in this scope
=== Build failed: 2 error (s), 0 warning (s) (0 minute (s), 0 second (s))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
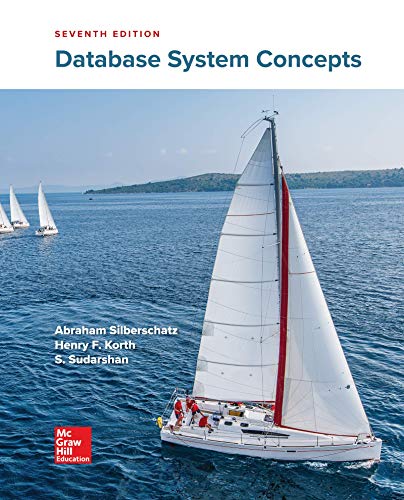
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
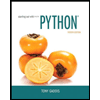
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
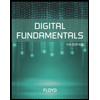
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
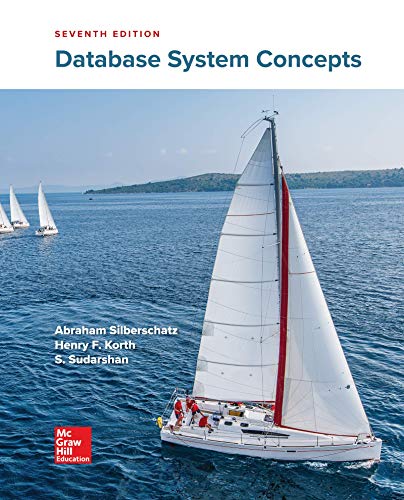
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
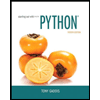
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
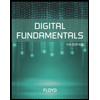
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
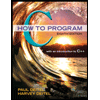
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
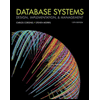
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
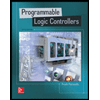
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education