1. You are to create a well-formed Python recursive function (i.e, there must be a stopping/base case and the recursive case must present a smaller problem), along with a call to that function. 2. Trace your function and call. Remember that you must keep track of memory for each function call and provide output. How would I trace a function? and keep track of its memory for each function call?
1. You are to create a well-formed Python recursive function (i.e, there must be a stopping/base case and the recursive case must present a smaller problem), along with a call to that function.
2. Trace your function and call. Remember that you must keep track of memory for each function call and provide output.
How would I trace a function? and keep track of its memory for each function call?

Tracing a function and keep tracking its memory:
- The below python program computes the sum of natural numbers using recursive function.
- The value of num is passed to the function "sum".
- If the num value is 1 or 0, it return the n value to main. It the base case for the program.
- Now compute the sum of natural number using a recursive call “res=sum+sum(num-1)”.
- The resource library is used to keep the memory size.
- Then print the size of memory block and sum of natural numbers.
PROGRAM:
#Import module
import resource
#Define the function
def sum(num):
#Check if n is either 1 or 0/base case
if num == 1 or num == 0:
#Return n
return num
#Update num with recursive function call
res = num+sum(num-1)
#Keep tracking the memory block size
print ("Size of allocated memory block := ", resource.getrusage(resource.RUSAGE_SELF).ru_maxrss)
#/Print the sum
print ("Sum of first %d natural numbers := %d\n" %(num, res))
#Return value
return res
#Call the function
sum(5)
Step by step
Solved in 4 steps with 2 images

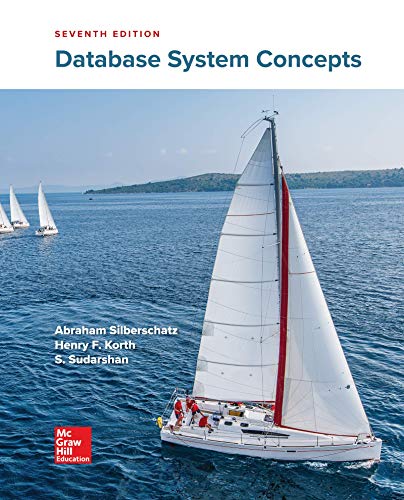
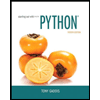
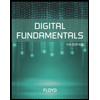
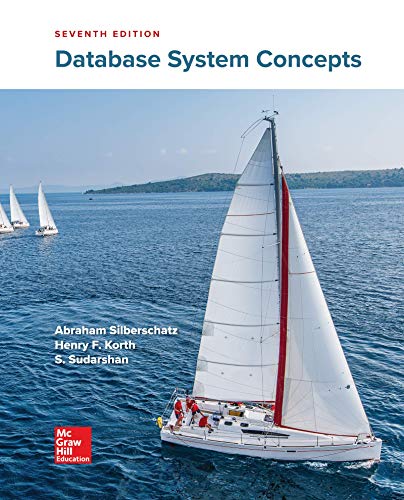
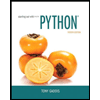
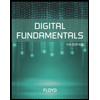
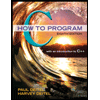
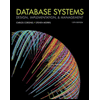
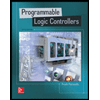