Write a program that reads 10 pairs of Cartesian coordinates from a file called "points.txt" and sorts them by increasing x-values, decreasing y-values, and increasing distance from the origin. Use only one sorting routine. Use an enumerated data type to keep track of the field on which the list is being sorted. The number of pairs should be in a global constant c++
Write a program that reads 10 pairs of Cartesian coordinates from a file called "points.txt" and sorts them by increasing x-values, decreasing y-values, and increasing distance from the origin. Use only one sorting routine. Use an enumerated data type to keep track of the field on which the list is being sorted. The number of pairs should be in a global constant c++
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JUST ONLY USE <iostream>.
Write a program that reads 10 pairs of Cartesian coordinates from a file called "points.txt" and sorts them by increasing x-values, decreasing y-values, and increasing distance from the origin. Use only one sorting routine. Use an enumerated data type to keep track of the field on which the list is being sorted. The number of pairs should be in a global constant c++

Transcribed Image Text:The image contains a code snippet and an illustrative diagram related to calculating the distance of a point from the origin using a struct in a programming context. Here's a detailed breakdown:
### Code Snippet:
- **Enum Definition:**
```c
enum fieldType {X, Y, DISTANCE};
```
This line defines an enumerated type named `fieldType` with three possible values: `X`, `Y`, and `DISTANCE`.
- **Struct Definition:**
```c
struct pointType
{
double x;
double y;
double dist;
};
```
This segment defines a structure `pointType` with three fields: `x`, `y`, and `dist`, all of which are of type `double`. This struct is designed to hold the coordinates `x` and `y` of a point, as well as the calculated distance `dist` from the origin.
### Illustrative Diagram:
- **Visual Elements:**
- A cartoon character (a goat) poses a question: "How far is the point (x, y) from the origin?"
- Another character (a wizard) responds with the formula for calculating the distance from the origin: "It’s the square root of x² + y²."
### Instructional Text:
- **Objective:**
At the bottom, instructional text guides the user to:
- Use the defined struct to hold points and calculate their distances from the origin.
- Read x and y coordinates from a file.
- Use the Pythagorean theorem (`√(x² + y²)`) to determine the distance of the point from the origin.
This content is designed to teach users how to represent points in a programming structure and perform basic mathematical operations to compute distances in a 2D plane.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
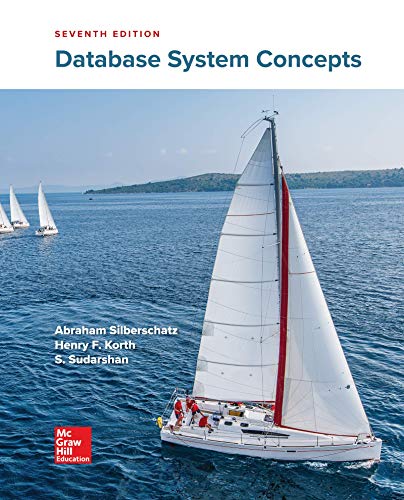
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
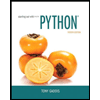
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
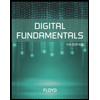
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
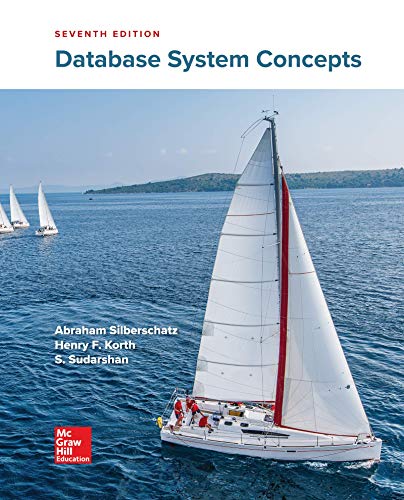
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
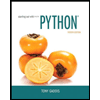
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
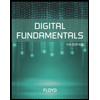
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
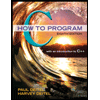
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
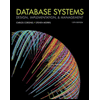
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
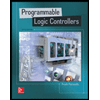
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education