Write a Python program that will read in an input file named RandomNumbers.txt and place all the positive values in a list. From there, display this list sorted in ascending order. Use at least 4 functions within the program. Remember, main() should become a to-do list calling the functions in the order in which they should execute. Each function should perform 1 task only. In addition to the above, be sure your code handles the following exception:
Write a Python program that will read in an input file named RandomNumbers.txt and place all the positive values in a list. From there, display this list sorted in ascending order.
Use at least 4 functions within the program. Remember, main() should become a to-do list calling the functions in the order in which they should execute. Each function should perform 1 task only.
In addition to the above, be sure your code handles the following exception:
- any IOError exception that is thrown when the file is opened and data is read from it
REQUIREMENTS:
- Use functions throughout. The function main should only call functions in the order needed.
- Keep the functions modular and performing only one task.
- Add a beginning and ending statement so the user will know when the program begins and ends.
- Comment throughout the code.
- The program should look for the file in the current directory.
Example:
If the input file contains the following data:
99 |
The program output should be:
Sorted Positive Values Opening input file.... Processing input file.... The list of positive integers is: 44 Closing input file.... End of results. |

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

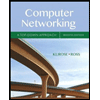
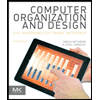
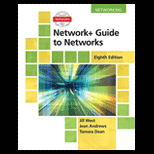
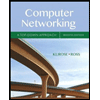
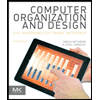
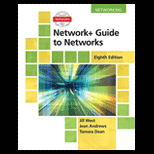
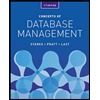
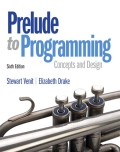
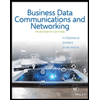