Write a program that reads words from a filename, which is given as a string argument. It should return the words from the file in a list, sorted in reverse alphabetical order (case insensitive) . For instance, if the file has bell tea Zebra apple yellow Then the output should be ['Zebra', 'yellow', 'tea', 'bell', 'apple'] def reverse_sorted_words(filename): # YOUR CODE HERE raise NotImplementedError()
Write a program that reads words from a filename, which is given as a string argument. It should return the words from the file in a list, sorted in reverse alphabetical order (case insensitive) . For instance, if the file has bell tea Zebra apple yellow Then the output should be ['Zebra', 'yellow', 'tea', 'bell', 'apple'] def reverse_sorted_words(filename): # YOUR CODE HERE raise NotImplementedError()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a program that reads words from a filename, which is given as a string argument. It should return the words from the file in a list, sorted in reverse alphabetical order (case insensitive) .
For instance, if the file has
bell tea Zebra apple yellow
Then the output should be
['Zebra', 'yellow', 'tea', 'bell', 'apple']
def reverse_sorted_words(filename):
# YOUR CODE HERE
raise NotImplementedError()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
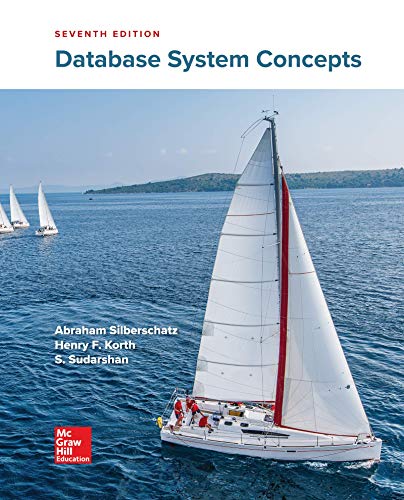
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
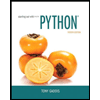
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
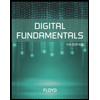
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
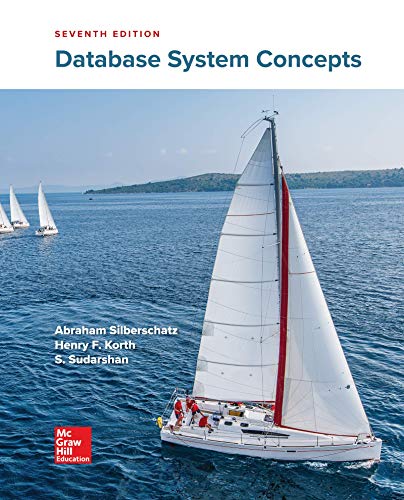
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
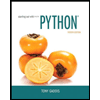
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
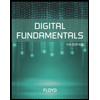
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
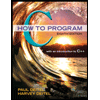
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
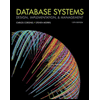
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
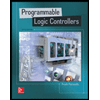
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education