Write a function path that returns the path from the root of the tree to the given value item if it exists and [] if it does not. You can assume all values are unique.
Write a function path that returns the path from the root of the tree to the given value item if it exists and [] if it does not. You can assume all values are unique.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Write a function `path` that returns the path from the root of the tree to the given value `item` if it exists and `[]` if it does not. You can assume all values are unique.
```python
def path(t, item):
"""
>>> t = Tree(9, [Tree(7, [Tree(3), Tree(2)]), Tree(5)])
>>> path(t, 2)
[9, 7, 2]
>>> path(t, 5)
[9, 5]
>>> path(t, 8)
[]
"""
"*** YOUR CODE HERE ***"
```](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2dbec8a5-7cbc-4cb4-99f8-f776f520cd0c%2F691af2b0-12cd-4654-a181-c735257786ba%2Fddo5p4j_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a function `path` that returns the path from the root of the tree to the given value `item` if it exists and `[]` if it does not. You can assume all values are unique.
```python
def path(t, item):
"""
>>> t = Tree(9, [Tree(7, [Tree(3), Tree(2)]), Tree(5)])
>>> path(t, 2)
[9, 7, 2]
>>> path(t, 5)
[9, 5]
>>> path(t, 8)
[]
"""
"*** YOUR CODE HERE ***"
```

Transcribed Image Text:### Python Tree Class Explanation
The following Python code defines a `Tree` class used for creating and manipulating tree-like data structures. Let's break down the components of this class:
```python
class Tree:
def __init__(self, value, branches=()):
self.value = value
for branch in branches:
assert isinstance(branch, Tree)
self.branches = list(branches)
def __repr__(self):
if self.branches:
branches_str = ', ' + repr(self.branches)
else:
branches_str = ''
return 'Tree({0}{1})'.format(self.value, branches_str)
def __str__(self):
def print_tree(t, indent=0):
tree_str = ' ' * indent + str(t.value) + "\n"
for b in t.branches:
tree_str += print_tree(b, indent + 1)
return tree_str
return print_tree(self).rstrip()
def is_leaf(self):
return not self.branches
```
#### Key Components:
1. **Initialization (`__init__` method):**
- `self.value`: Stores the value of the node.
- `self.branches`: Initializes as a list from the `branches` tuple, ensuring each branch is an instance of `Tree` using the `assert` statement.
2. **Representation (`__repr__` method):**
- Provides a string representation of the `Tree` object for debugging.
- Displays the tree's value and a string of its branches if they exist.
3. **String Representation (`__str__` method):**
- Nested function `print_tree` prints the tree in a structured format with indentation for branches.
- Trailing whitespace is removed by `rstrip()` for cleaner output.
4. **Leaf Check (`is_leaf` method):**
- Returns `True` if the tree node is a leaf (i.e., it has no branches), otherwise returns `False`.
This implementation is useful for understanding the basic construction and traversal of trees in Python. The `Tree` class supports creating hierarchical data structures with potentially multiple branches at each node.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
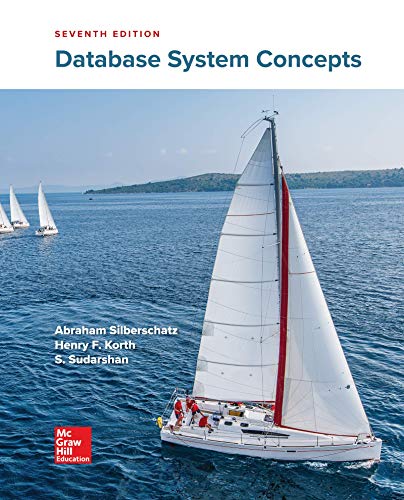
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
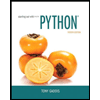
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
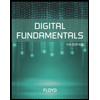
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
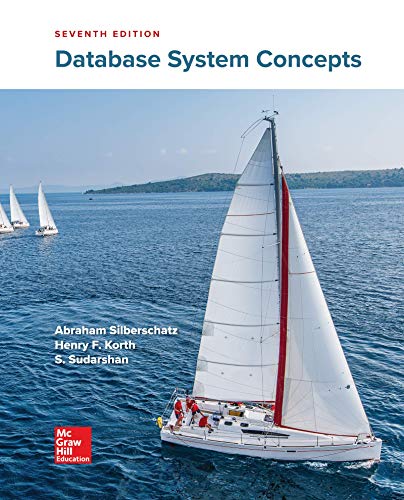
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
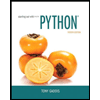
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
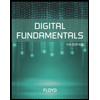
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
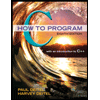
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
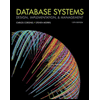
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
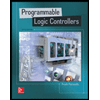
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education