Write a class called Circle that contains: a. Three private instance variables: radius (of the type double), color (of the type String), and area (of type double). b. Two constructors - a default constructor with no argument (default value of radius is 2.0, and color is Green) and a parameterized constructor, which initializes only the radius and the color variables. c. Getter and setter for the radius and color variables. d. Two public methods: calculateArea(), and getPerimeter, which return the area and perimeter of a circle instance, respectively. Both methods have return. Then write a driver class that tests the Circle class. In the driver class, create two objects of Circle class, one that tests the default constructor of the Circle class (here no user input is required) and then get the color, radius, area, and perimeter of that object and display them. The second object should test the parameterized constructor (here you need to ask user to give you input to initialize the class variables). The code should ensure that the radius given by the user is positive. If the radius is not positive, the code will keep asking the user to reenter a value. Once the radius is positive, the code should get the color, radius, area, and perimeter of that object accordingly and display them. Then you need to ask the user again to give you a new radius for the second object of the class Circle. Again, the code should ensure that the new radius given by the user is positive. If the radius is not positive, the code will keep asking the user to reenter a value. Then you need to read the new radius and calculate the new area and perimeter accordingly and display them. You need to format your output to look as below (print only 4 values after decimal). • To ensure that the radius is positive, loop (with sentinel) and conditional should be used otherwise you will lose marks. • If the class variables are not private, you will lose marks. • If you don’t create setter/getter methods, you will lose marks. • If you don’t do formatting for the output as requested, you will lose marks. • Don’t hardcode the output otherwise marks will be deducted.
Write a class called Circle that contains:
a. Three private instance variables: radius (of the type double), color (of the
type String), and area (of type double).
b. Two constructors - a default constructor with no argument (default value of radius
is 2.0, and color is Green) and a parameterized constructor, which initializes only
the radius and the color variables.
c. Getter and setter for the radius and color variables.
d. Two public methods: calculateArea(), and getPerimeter, which return the area
and perimeter of a circle instance, respectively. Both methods have return.
Then write a driver class that tests the Circle class. In the driver class, create two objects
of Circle class, one that tests the default constructor of the Circle class (here no user input
is required) and then get the color, radius, area, and perimeter of that object and display
them.
The second object should test the parameterized constructor (here you need to ask user
to give you input to initialize the class variables). The code should ensure that the radius
given by the user is positive. If the radius is not positive, the code will keep asking the user
to reenter a value. Once the radius is positive, the code should get the color, radius, area,
and perimeter of that object accordingly and display them. Then you need to ask the user
again to give you a new radius for the second object of the class Circle. Again, the code
should ensure that the new radius given by the user is positive. If the radius is not positive, the code will keep asking the user to reenter a value. Then you need to read the new
radius and calculate the new area and perimeter accordingly and display them.
You need to format your output to look as below (print only 4 values after decimal).
• To ensure that the radius is positive, loop (with sentinel) and conditional should
be used otherwise you will lose marks.
• If the class variables are not private, you will lose marks.
• If you don’t create setter/getter methods, you will lose marks.
• If you don’t do formatting for the output as requested, you will lose marks.
• Don’t hardcode the output otherwise marks will be deducted.


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

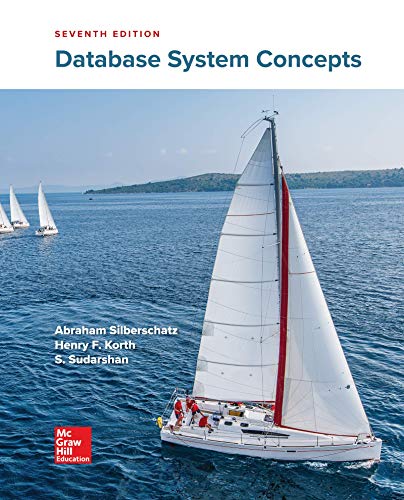
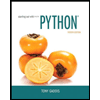
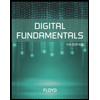
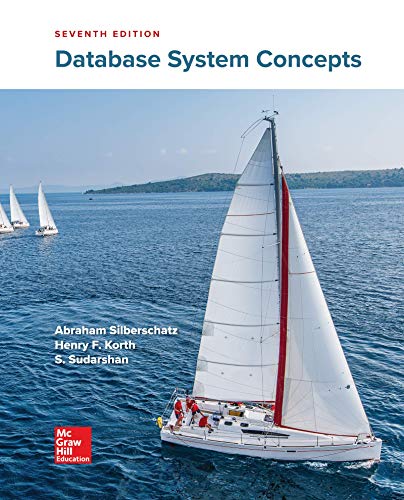
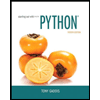
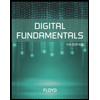
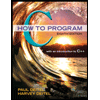
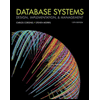
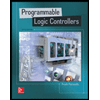