Hi can you assist me on the problem below for a class and a test class that conforms to the following specifications: 1. The name of the class is RightTriangle 2. The class has member variables base and height 3. The class has a constructor that initializes base and height 4. The class has accessors and mutators for base and height 5. The class has a toString method that produces String representation of a RightTriangle like the following example for a triangle with base 12 and height 10. Base : 12 Height 10 6. The class has a method called findArea to compute the area of the right triangle. Here is a suggested implementation. public double findArea() { return 0.5* this.base*this.height; } 7. The class using proper naming conventions Write a test class called RightTriangleTest that conforms to the following: 1. Create an object with base = 10 and height = 20, for example myTriangle. 2. To print the toString for myTriangle. 3. To print the area of myTriangle. System.out.println(“The area is : “+ myTriangle.findArea(); 4. Use the mutators to change the base to 5 and the height to 4. 5. To print the toString with the new values of base and height 6. To print the new value of area
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Hi can you assist me on the problem below for a class and a test class that conforms to the
following specifications:
1. The name of the class is RightTriangle
2. The class has member variables base and height
3. The class has a constructor that initializes base and height
4. The class has accessors and mutators for base and height
5. The class has a toString method that produces String representation of a RightTriangle
like the following example for a triangle with base 12 and height 10.
Base : 12 Height 10
6. The class has a method called findArea to compute the area of the right triangle. Here is a
suggested implementation.
public double findArea()
{
return 0.5* this.base*this.height;
}
7. The class using proper naming conventions
Write a test class called RightTriangleTest that conforms to the following:
1. Create an object with base = 10 and height = 20, for example myTriangle.
2. To print the toString for myTriangle.
3. To print the area of myTriangle.
System.out.println(“The area is : “+ myTriangle.findArea();
4. Use the mutators to change the base to 5 and the height to 4.
5. To print the toString with the new values of base and height
6. To print the new value of area
Unlock instant AI solutions
Tap the button
to generate a solution
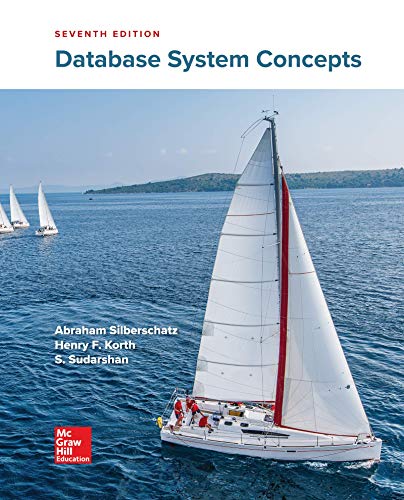
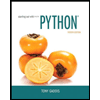
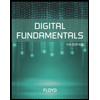
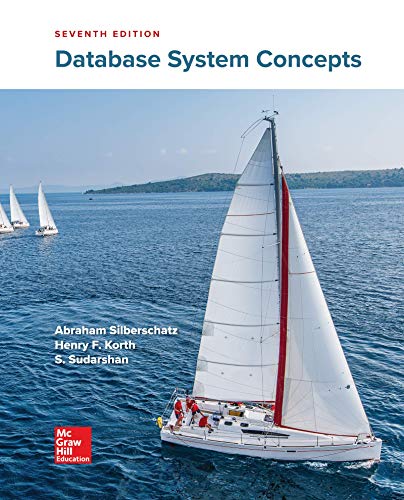
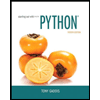
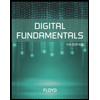
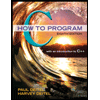
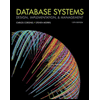
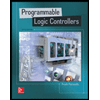