Write a C++ program that opens a file named data.txt which contains data written in three lines. Each line starts with a word that represents one of the following mathmetical functions: sin, cos, tan, asin, acos, atan, exp, log, log10, pow, sqrt, ciel, floor, min, and max. The word is followed by either one or two double floating-point values which represent the input**(s)** for the function. Notice that functions pow, min, and max will be followed by two values while all other functions will be followed by a single value. The program should read the data in the file and compute the result for each line. Lastly, the program should output the results on the console using the table format shown in the sample test case and in the deascending order of the mathmatical functions names in the file. Notice that the width of the first column in the table (which contains the function name) is 5 and it is aligned to the left. The second column in the table which contains the input**(s)** for the function enclosed by paranthesis has a width of 15. Notice that each input value must be printed using exactly four fractional digits. The third column always contains the three characters: "=". Lastly, the fourth column contains the result of each line printed with exactly four fractional digits. 1/0 Program Input: The data that is read from the file data.txt. Program Output: The data and the output in the table format shown in the sample test case and described above. Sample Test Case Input: Suppose the contents of the file data.txt are as follow: floor 7.74327 asin 0.87564 pow 1.5 4 Output: (1.5000, 4.0000) = pow floor (7.7433) asin (0.8756) II • Do not add any cout statements except for the final outputs as shown in the sample case. Do not add "Enter the file name", "the result =" or any similar prompts. • The width of each table column is given in the problem statement. Do not add any extra spaces. • In the third column, there is exactly one space before the assignment operator and one space after it. • Your program must use data.txt as the name of the file. • Your program must produce correct results for any possible values for the functions and the floating point numbers in the file data.txt. However, notice that the floating point numbers in the file are always in the range from 0 to 10 exclusive (i.e. 0 and 10 are not part of the range). • Your program can assume that the names of the functions in the data.txt file are always different and in lowercase and must be printed in lowercase as well. • You can include other libraries in your code if needed. 3
Write a C++ program that opens a file named data.txt which contains data written in three lines. Each line starts with a word that represents one of the following mathmetical functions: sin, cos, tan, asin, acos, atan, exp, log, log10, pow, sqrt, ciel, floor, min, and max. The word is followed by either one or two double floating-point values which represent the input**(s)** for the function. Notice that functions pow, min, and max will be followed by two values while all other functions will be followed by a single value. The program should read the data in the file and compute the result for each line. Lastly, the program should output the results on the console using the table format shown in the sample test case and in the deascending order of the mathmatical functions names in the file. Notice that the width of the first column in the table (which contains the function name) is 5 and it is aligned to the left. The second column in the table which contains the input**(s)** for the function enclosed by paranthesis has a width of 15. Notice that each input value must be printed using exactly four fractional digits. The third column always contains the three characters: "=". Lastly, the fourth column contains the result of each line printed with exactly four fractional digits. 1/0 Program Input: The data that is read from the file data.txt. Program Output: The data and the output in the table format shown in the sample test case and described above. Sample Test Case Input: Suppose the contents of the file data.txt are as follow: floor 7.74327 asin 0.87564 pow 1.5 4 Output: (1.5000, 4.0000) = pow floor (7.7433) asin (0.8756) II • Do not add any cout statements except for the final outputs as shown in the sample case. Do not add "Enter the file name", "the result =" or any similar prompts. • The width of each table column is given in the problem statement. Do not add any extra spaces. • In the third column, there is exactly one space before the assignment operator and one space after it. • Your program must use data.txt as the name of the file. • Your program must produce correct results for any possible values for the functions and the floating point numbers in the file data.txt. However, notice that the floating point numbers in the file are always in the range from 0 to 10 exclusive (i.e. 0 and 10 are not part of the range). • Your program can assume that the names of the functions in the data.txt file are always different and in lowercase and must be printed in lowercase as well. • You can include other libraries in your code if needed. 3
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
write a c++ code, please test it

Transcribed Image Text:Write a C++ program that opens a file named
data.txt which contains data written in three
lines. Each line starts with a word that
represents one of the following mathmetical
functions: sin, cos, tan, asin, acos, atan, exp,
log, log10, pow, sqrt, ciel, floor, min, and max.
The word is followed by either one or two
double floating-point values which represent
the input**(s)** for the function. Notice that
functions pow, min, and max will be followed
by two values while all other functions will be
followed by a single value.
The program should read the data in the file
and compute the result for each line. Lastly,
the program should output the results on the
console using the table format shown in the
sample test case and in the deascending order
of the mathmatical functions names in the file.
Notice that the width of the first column in the
table (which contains the function name) is 5
and it is aligned to the left. The second column
in the table which contains the input**(s)** for
the function enclosed by paranthesis has a
width of 15. Notice that each input value must
be printed using exactly four fractional digits.
The third column always contains the three
characters: " = ". Lastly, the fourth column
contains the result of each line printed with
exactly four fractional digits.
1/0
Program Input:
The data that is read from the file
data.txt.
Program Output:
The data and the output in the table
format shown in the sample test case
and described above.
Sample Test Case
Input:
Suppose the contents of the file data.txt
are as follow:
floor 7.74327
asin 0.87564
pow 1.5 4
Output:
(1.5000, 4.0000) =
pow
floor (7.7433)
asin (0.8756)
• Do not add any cout statements
except for the final outputs as
shown in the sample case. Do not
add "Enter the file name", "the
result =" or any similar prompts.
• The width of each table column is
given in the problem statement. Do
not add any extra spaces.
. In the third column, there is exactly
one space before the assignment
operator and one space after it.
• Your program must use data.txt
as the name of the file.
• Your program must produce
correct results for any possible
values for the functions and the
floating point numbers in the file
data.txt. However, notice that the
floating point numbers in the file
are always in the range from 0 to
10 exclusive (i.e. 0 and 10 are not
part of the range).
• Your program can assume that the
names of the functions in the
data.txt file are always different
and in lowercase and must be
printed in lowercase as well.
• You can include other libraries in
your code if needed.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
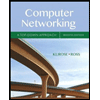
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
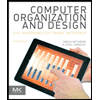
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
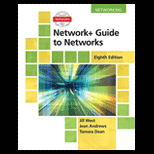
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
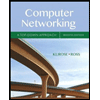
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
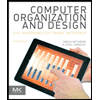
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
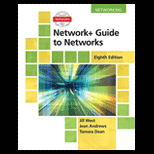
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
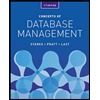
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
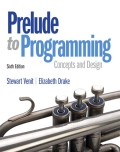
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
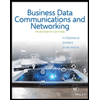
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY