Write a program digits sum.cpp that asks the user to enter a series of single-digit with nothing separating them (no negative number). Read the input as a C-string with a maximum length of 10. The program should display the sum of all the single-digit numbers in the string. For example, if the user enters 25 14, the program should display 12, which is the sum of 2, 5, 1, and 4. The program will continue asking the user input until the user enters a zero (i.e., 0) number. Note & Hint:
Write a program digits sum.cpp that asks the user to enter a series of single-digit with nothing separating them (no negative number). Read the input as a C-string with a maximum length of 10. The program should display the sum of all the single-digit numbers in the string. For example, if the user enters 25 14, the program should display 12, which is the sum of 2, 5, 1, and 4. The program will continue asking the user input until the user enters a zero (i.e., 0) number. Note & Hint:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I have no idea how to complete this C++ assignment

Transcribed Image Text:### Program Explanation
The objective is to write a C++ program named `digits_sum.cpp` that prompts the user to enter a string of single-digit numbers (with no separators), and then calculates the sum of these digits. The program should continue to prompt for new inputs until the user enters zero (i.e., the digit '0').
#### Key Steps:
- Use a **C-string** to handle the input, with a maximum length of 10 characters.
- Ensure each character of the input is a valid digit.
- Use the `isdigit()` function from the `<cctype>` library for digit validation.
- Each valid character is converted to its numerical value by subtracting the character '0'.
- Continue processing until the input '0' is entered.
#### Notes & Hints:
- Allocate a sufficiently large character array to manage input.
- Validate all characters within the input string.
- Example code to convert a character to its integer form:
```cpp
char aDigit = '4';
int aNum;
aNum = aDigit - '0'; // now aNum = 4;
```
- Use the C-string library `<cstring>` to validate input length.
### Sample Output Explained
The output section illustrates various interactions with the `digits_sum.cpp` program:
1. **Input of "2514":**
- Prompt: “Please enter a number (max. length of 10) to sum: 2514”
- Result: “The sum of the digits 2514 is 12”
2. **Input of "1234567890":**
- Prompt: “Please enter a number (max. length of 10) to sum: 1234567890”
- Result: “The sum of the digits 1234567890 is 45”
3. **Input exceeding allowed length:**
- Prompt: “Please enter a number (max. length of 10) to sum: 123456789012”
- Result: “The number exceeds the length of 10”
4. **Invalid input containing non-digit characters:**
- Prompt: “Please enter a number (max. length of 10) to sum: 123ABC”
- Result: “Invalid input: non-digit character detected!”
5. **Termination condition:**
- Prompt: “Please enter a number (max. length of 10) to sum: 0”
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
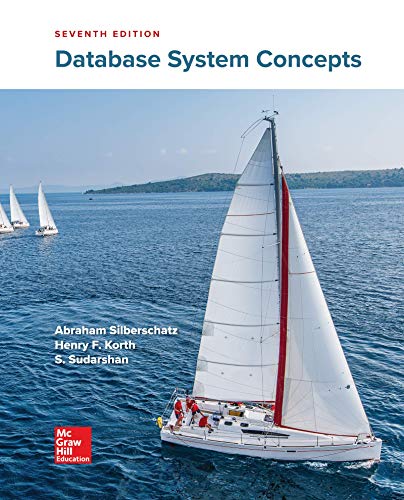
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
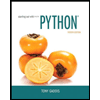
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
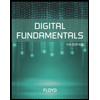
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
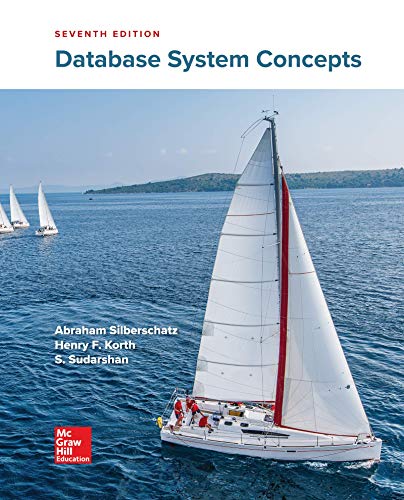
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
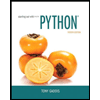
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
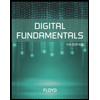
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
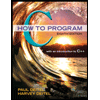
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
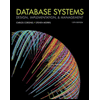
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
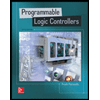
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education