Why is the line "input.read(blocks[i].getBlock(), blockSize);" giving me an access violation error?
Why is the line "input.read(blocks[i].getBlock(), blockSize);" giving me an access violation error?
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <memory>
static const int BLOCKSIZE = 4096; //buffer blocksize
static const int POOL_SIZE = 5; //number of buffer block in the buffer pool
using namespace std;
class BufferBlock
{
public:
//sz is the size of the character array data
//points to.
BufferBlock() {
}
BufferBlock(char* data, int sz = 4096) {
block = new char[sz];
for (int i = 0; i < sz; i++)
{
block[i] = data[i];
}
}
~BufferBlock() {}
//read the block from pos to pos + sz-1 (or to the end of the block)
void getData(int pos, int sz, char* data) {
int i = 0;
int temp = 0;
temp = pos;
while (temp < pos + sz) {
data[i] = block[temp];
i++;
temp++;
}
}
//setID
void setID(int id) {
blockID = id / BLOCKSIZE;
}
//getID
int getID() const {
return blockID;
}
//getBlocksize
int getBlocksize() const { return BLOCKSIZE; }
//return the block
char* getBlock() const {
return block;
}
//set the block
void setBlock(char* blk) {
blk = block;
}
char* block;
int blockID;
private:
};
class BufferPool {
public:
BufferPool(string filename, int poolSize, int blockSize) {
input.open(filename, fstream::in | fstream::binary);
blocks = make_unique<BufferBlock[]>(poolSize);
for (int i = 0; i < poolSize; i++)
{
int pos = i * blockSize;
input.seekg(pos);
input.read(blocks[i].getBlock(), blockSize);
}
}
void getBytes(char* space, int sz, int pos) {
input.seekg(pos);
input.read(space, sz);
}
private:
/* BufferBlock* blocks;*/
std::unique_ptr<BufferBlock[]> blocks;
fstream input;
};
void printChars(char* ch, int sz, int blkid) {
cout << "My data for block " << blkid << " is: \"";
for (int i = 0; i < sz; i++) {
cout << ch[i];
}
cout << "\"\n";
}
int main() {
int pos = 5030;
int size = 10;
char* buffer = new char[size];
BufferPool* bp = new BufferPool("mydatafile.txt", 10, 10);
bp->getBytes(buffer, size, pos);
printChars(buffer, size, pos/4096);
delete[] buffer;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

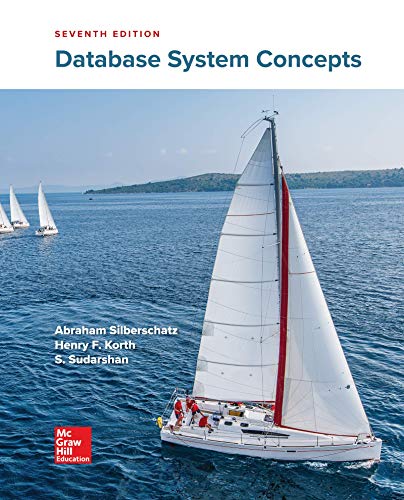
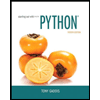
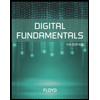
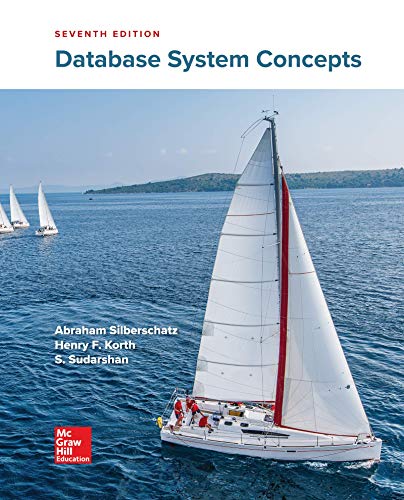
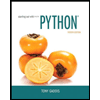
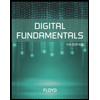
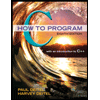
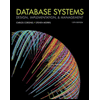
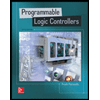