I need some help making this code more simple. 2) Algorithm tokenList = "{}()[]" initialize stack to empty Get file name from user: fileName Open the file (fileName) read line from fileName into strLine while not EOF (end of file) find tokenList items in strLine for each token in strLine if token = "[" then push "]" into stack else if token = "(" then push ")" into stack else if token = "{" then push "}" into stack else if token = "]" OR token = ")" OR token = "}" then pop stack into poppedToken if poppedToken not equal token print "Incorrect Grouping Pairs" exit end if end if next token end while if stack is empty print "Correct Grouping Pairs" else print "Incorrect Grouping Pairs" end if
I need some help making this code more simple.
2)
tokenList = "{}()[]"
initialize stack to empty
Get file name from user: fileName
Open the file (fileName)
read line from fileName into strLine
while not EOF (end of file)
find tokenList items in strLine
for each token in strLine
if token = "[" then
push "]" into stack
else if token = "(" then
push ")" into stack
else if token = "{" then
push "}" into stack
else if token = "]" OR token = ")" OR token = "}" then
pop stack into poppedToken
if poppedToken not equal token
print "Incorrect Grouping Pairs"
exit
end if
end if
next token
end while
if stack is empty
print "Correct Grouping Pairs"
else
print "Incorrect Grouping Pairs"
end if
![6) Let's pop one more time
poppeditem = "]"
Design Strategy
We have 3 types of tokens we're looking for:
1) Parenthesis ( )
2) Brackets []
3) Curly Brackets {}
There's an open and closed version of each token.
Look at this line: x = [a, b(1,2,3], c] This is an unmatched pair, because the closed bracket after the
number 3, should have been a closed parenthesis, to complete the open parenthesis before the number
1. Here's what the correct version looks like: x = [a, b(1,2,3), c]
A stack is a perfect data structure to keep track of the tokens in the order they were encountered. For
the tokens to be matched, there always has to be a closed token the directly precedes the same version
of an open token. Anytime we come across a closed token we can just pop off the latest item in a stack
to see if it matches.
Algorithm
Let's read a file a line at a time and scan for tokens on each line. As we encounter an open token, push
it into the stack. When we encounter a closed token we pop an item from the stack and see if it
matches the open token. If it doesn't we print a message saying "Unmatched Pair". When we finish
reading the file we check to see if the queue is empty. If it is "Success" or else it's an unmatched pair.
You can use the partial algorithm below and fill in the rest of it if you'd like. Iľ'm not expecting you to
provide details for managing push and pop functions. You can assume that there's a pop and push
function built in that you can utilize.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc43e03e1-b353-4d50-a726-bab1a8232fef%2Fb40ea67a-c4dc-424b-8dc5-1bde55af32cc%2F02mpv9_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

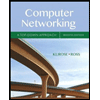
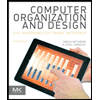
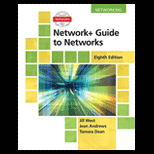
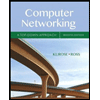
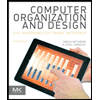
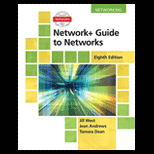
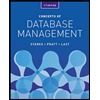
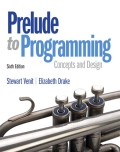
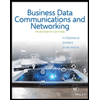