Why is it giving me an error and what do I have to change? PYTHON # Problem 2 # Implement a hashtable using an array. Your implementation should include public methods for insertion, deletion, and # search, as well as helper methods for resizing. The hash table is resized when the max chain length becomes greater # than 3 during insertion of a new item. You will be using linear chaining technique for collision resolution. Assume # the key to be an integer and use the hash function h(k) = k mod m where m is the size of the hashtable. You can use # python list methods in your implementation of the chain or you can also use your linked list implementation from # coding assignment 2, problem 1. You can make necessary changes to __hashtable initialization in the __init__ method # if you are using your linked list implementation. The provided code uses python lists for the __hashtable variable. class HashTableChain: def __init__(self, size=10): # Initialize the hashtable with the given size and an empty array to hold the key-value pairs. self.__size = size # size of the hashtable self.__hashtable = [[] for _ in range(size)] self.__maxcount = (0, 0) # tuple to keep track of the maximum chain length and index in the current hashtable def __contains__(self, key): return self.__search(key) def __next_prime(self, x): def is_prime(x): return all(x % i for i in range(2, x)) return min([a for a in range(2 * x + 1, x * x) if is_prime(a)]) def __updatemaxcount(self): # Method updating the __maxcount to the index and length of maximum chain. On inserting, deletion, resizing this # should be called. i = 0 while i < self.__size: if len(self.__hashtable[i]) > self.__maxcount[1]: self.__maxcount = (i, len(self.__hashtable[i])) i += 1 def __hash(self, key): # Hash function to be used for mapping a key to the indices in the hashtable. bucket = key % self.__size return bucket def __resize(self): # Resizes the hashtable to the next prime number after current table size. Also, rehashes all the current items. pass def insert(self, key, value): # Insert a new key-value pair (as a python list) into the hashtable. When the max chain length becomes greater # than 3 resizes the current hash table before inserting the new item. bucket = self.__hash(key) item = self.__hashtable[bucket] new = [key, value] for self.__hashtable in item: if key == item[0]: item[1] = value return True item.append(new) self.__updatemaxcount() def delete(self, key): # Remove a key-value pair from the hashtable. bucket = self.__hash(key) item = self.__hashtable[bucket] for self.__hashtable in item: if key == item[0]: self.__hashtable.remove(item) self.__updatemaxcount() return True def __search(self, key): # Find the item associated with a given key in the hashtable. bucket = self.__hash(key) item = self.__hashtable[bucket] for self.__hashtable in item: if key == item[0]: return item[0] return None def showitems(self): # prints all the items in the hashtable. count = 0 m = self.__size print(self.__maxcount[0]) print(self.__maxcount[1]) while count < m: print(self.__hashtable[count]) count += 1 return False # Problem 2 Test Code testb = HashTableChain() print("Start") testb.showitems() testb.insert(0, 'first') testb.insert(5, 'second') testb.insert(2, 'third') print("Test insert") testb.showitems() testb.delete(0) print("Test delete:") testb.showitems
Why is it giving me an error and what do I have to change?
PYTHON
# Problem 2
# Implement a hashtable using an array. Your implementation should include public methods for insertion, deletion, and
# search, as well as helper methods for resizing. The hash table is resized when the max chain length becomes greater
# than 3 during insertion of a new item. You will be using linear chaining technique for collision resolution. Assume
# the key to be an integer and use the hash function h(k) = k mod m where m is the size of the hashtable. You can use
# python list methods in your implementation of the chain or you can also use your linked list implementation from
# coding assignment 2, problem 1. You can make necessary changes to __hashtable initialization in the __init__ method
# if you are using your linked list implementation. The provided code uses python lists for the __hashtable variable.
class HashTableChain:
def __init__(self, size=10):
# Initialize the hashtable with the given size and an empty array to hold the key-value pairs.
self.__size = size # size of the hashtable
self.__hashtable = [[] for _ in range(size)]
self.__maxcount = (0, 0) # tuple to keep track of the maximum chain length and index in the current hashtable
def __contains__(self, key):
return self.__search(key)
def __next_prime(self, x):
def is_prime(x):
return all(x % i for i in range(2, x))
return min([a for a in range(2 * x + 1, x * x) if is_prime(a)])
def __updatemaxcount(self):
# Method updating the __maxcount to the index and length of maximum chain. On inserting, deletion, resizing this
# should be called.
i = 0
while i < self.__size:
if len(self.__hashtable[i]) > self.__maxcount[1]:
self.__maxcount = (i, len(self.__hashtable[i]))
i += 1
def __hash(self, key):
# Hash function to be used for mapping a key to the indices in the hashtable.
bucket = key % self.__size
return bucket
def __resize(self):
# Resizes the hashtable to the next prime number after current table size. Also, rehashes all the current items.
pass
def insert(self, key, value):
# Insert a new key-value pair (as a python list) into the hashtable. When the max chain length becomes greater
# than 3 resizes the current hash table before inserting the new item.
bucket = self.__hash(key)
item = self.__hashtable[bucket]
new = [key, value]
for self.__hashtable in item:
if key == item[0]:
item[1] = value
return True
item.append(new)
self.__updatemaxcount()
def delete(self, key):
# Remove a key-value pair from the hashtable.
bucket = self.__hash(key)
item = self.__hashtable[bucket]
for self.__hashtable in item:
if key == item[0]:
self.__hashtable.remove(item)
self.__updatemaxcount()
return True
def __search(self, key):
# Find the item associated with a given key in the hashtable.
bucket = self.__hash(key)
item = self.__hashtable[bucket]
for self.__hashtable in item:
if key == item[0]:
return item[0]
return None
def showitems(self):
# prints all the items in the hashtable.
count = 0
m = self.__size
print(self.__maxcount[0])
print(self.__maxcount[1])
while count < m:
print(self.__hashtable[count])
count += 1
return False
# Problem 2 Test Code
testb = HashTableChain()
print("Start")
testb.showitems()
testb.insert(0, 'first')
testb.insert(5, 'second')
testb.insert(2, 'third')
print("Test insert")
testb.showitems()
testb.delete(0)
print("Test delete:")
testb.showitems()

Step by step
Solved in 2 steps with 3 images

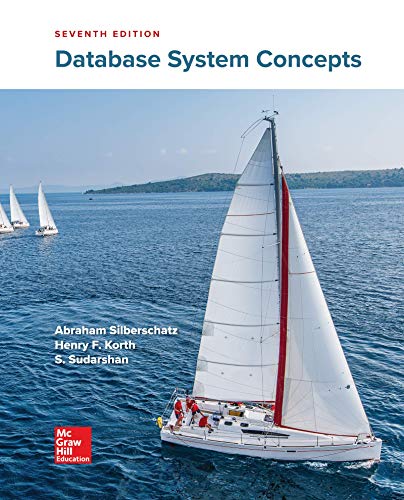
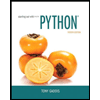
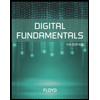
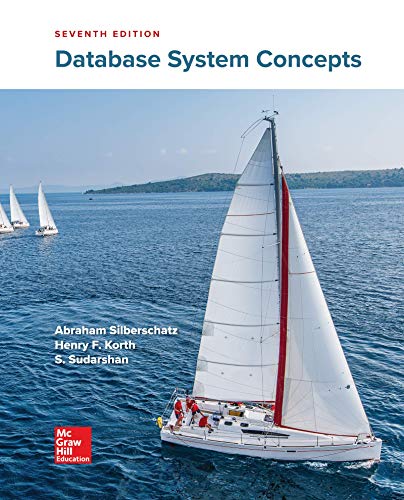
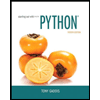
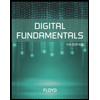
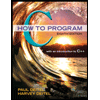
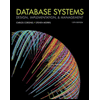
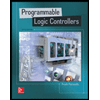