write a simple hashing function that uses addition and multiplication and then find a pair of strings that will return the same hash value for different strings (i.e you will cause a Hash Collision). The algorithm uses multiplication based on the position of a letter in the hash to avoid a hash collision when two letters are transposed like in 'ABCDE' and 'ABDCE'. Your strings need to be at least three characters long and no more than 10 characters long. Here is the code that computes your hash: while True: txt = input("Enter a string: ") if len(txt) < 1 : break hv = 0 pos = 0 for let in txt: pos = ( pos % 2 ) + 1 hv = (hv + (pos * ord(let))) % 1000000 print(let, pos, ord(let), hv) print(hv, txt) For simplicity we will only use upper and lower case ASCII letters in our text strings. Enter a string: ABCDE A 1 65 65 B 2 66 197 C 1 67 264 D 2 68 400 E 1 69 469 469 ABCDE Enter a string: BACDE B 1 66 66 A 2 65 196 C 1 67 263 D 2 68 399 E 1 69 468 468 BACDE
write a simple hashing function that uses addition and multiplication and then find a pair of strings that will return the same hash value for different strings (i.e you will cause a Hash Collision). The
Here is the code that computes your hash:
while True:
txt = input("Enter a string: ")
if len(txt) < 1 : break
hv = 0
pos = 0
for let in txt:
pos = ( pos % 2 ) + 1
hv = (hv + (pos * ord(let))) % 1000000
print(let, pos, ord(let), hv)
print(hv, txt)
For simplicity we will only use upper and lower case ASCII letters in our text strings.
Enter a string: ABCDE
A 1 65 65
B 2 66 197
C 1 67 264
D 2 68 400
E 1 69 469
469 ABCDE
Enter a string: BACDE
B 1 66 66
A 2 65 196
C 1 67 263
D 2 68 399
E 1 69 468
468 BACDE

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

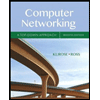
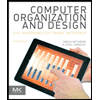
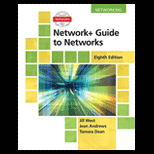
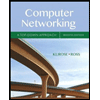
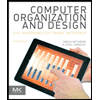
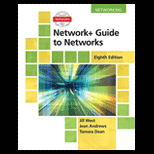
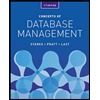
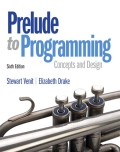
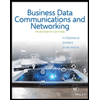