Whats wrong with the Java code below? Thank you! Source code: package FlowLayout; public class FlowLayout extends FlowLayout2 { public static void main(String[] args) // This is int main() { FlowLayout2 frame = new FlowLayout2(); frame.setSize(300, 200); frame.setVisible(true); } } ====================================================================================== ====================================================================================== package FlowLayout; // Import needed packages import java.awt.event.ActionListener; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JTextField; public class FlowLayout2 extends JFrame implements ActionListener { // Declare protected class members protected JFrame frame; protected JPanel panel; protected JTextField textBox; protected JComboBox comboBox; public FlowLayout2() // Class Constructor { // Create a JFrame to hold the components frame = new JFrame("Dropdown App"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Create a panel to hold the components panel = new JPanel(); // Create a text box textBox = new JTextField(13); // Create a ComboBox String[] options = {"Date & Time", "Save", "Change Color", "Logout"}; comboBox = new JComboBox<>(options); comboBox.addActionListener(this); comboBox.setEditable(true); // Add the ComboBox to the panel panel.add(comboBox); // Add the Text Field panel.add(textBox); // Set the layout manager for the panel panel.setLayout(new java.awt.FlowLayout()); // Add the panel to the frame frame.getContentPane().add(panel); } } ========================================================================================== ========================================================================================== package FlowLayout; // Import needed packages import java.awt.Color; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.FileWriter; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import javax.swing.JFrame; import javax.swing.JOptionPane; public class FlowLayout3 extends FlowLayout2 implements ActionListener { public void actionPerformed(ActionEvent eventOccurred) // method for listening for actions { if(eventOccurred.getSource() == "Date & Time") // If date_time is heard, display date and time { DateTimeFormatter dateTimeFormat = DateTimeFormatter.ofPattern("MM/dd/YYYY hh:mm:ss"); LocalDateTime current=LocalDateTime.now(); textBox.setText(dateTimeFormat.format(current)); } if(eventOccurred.getSource()== "Save") // If write_to_file is heard, write { // whatever is displayed to a .txt file String save = textBox.getText(); try // Try block for exceptions { FileWriter saving = new FileWriter("Log.txt"); saving.write(save); saving.close(); // So that the User is aware that the data was saved to a file JOptionPane.showMessageDialog(this, "Everything displayed has been saved in a file named \'Log.txt\'."); } catch(Exception menuException) // Catch block for the exceptions thrown { textBox.setText("Exception is " + menuException); } } if(eventOccurred.getSource() == "Change Color") // If change_color is selected, { // Make the background green. frame.getContentPane().setBackground(Color.GREEN); } if(eventOccurred.getSource() == "Logout") // If exit_application is selected { // logout of the application frame.setVisible(false); JOptionPane.showMessageDialog(this, "See ya, have a great day!"); } frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Make sure the application closes } }
Whats wrong with the Java code below?
Thank you!
Source code:
package FlowLayout;
public class FlowLayout extends FlowLayout2
{
public static void main(String[] args) // This is int main()
{
FlowLayout2 frame = new FlowLayout2();
frame.setSize(300, 200);
frame.setVisible(true);
}
}
======================================================================================
======================================================================================
package FlowLayout;
// Import needed packages
import java.awt.event.ActionListener;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class FlowLayout2 extends JFrame implements ActionListener
{
// Declare protected class members
protected JFrame frame;
protected JPanel panel;
protected JTextField textBox;
protected JComboBox<String> comboBox;
public FlowLayout2() // Class Constructor
{
// Create a JFrame to hold the components
frame = new JFrame("Dropdown App");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create a panel to hold the components
panel = new JPanel();
// Create a text box
textBox = new JTextField(13);
// Create a ComboBox
String[] options = {"Date & Time", "Save", "Change Color", "Logout"};
comboBox = new JComboBox<>(options);
comboBox.addActionListener(this);
comboBox.setEditable(true);
// Add the ComboBox to the panel
panel.add(comboBox);
// Add the Text Field
panel.add(textBox);
// Set the layout manager for the panel
panel.setLayout(new java.awt.FlowLayout());
// Add the panel to the frame
frame.getContentPane().add(panel);
}
}
==========================================================================================
==========================================================================================
package FlowLayout;
// Import needed packages
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.FileWriter;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class FlowLayout3 extends FlowLayout2 implements ActionListener
{
public void actionPerformed(ActionEvent eventOccurred) // method for listening for actions
{
if(eventOccurred.getSource() == "Date & Time") // If date_time is heard, display date and time
{
DateTimeFormatter dateTimeFormat = DateTimeFormatter.ofPattern("MM/dd/YYYY hh:mm:ss");
LocalDateTime current=LocalDateTime.now();
textBox.setText(dateTimeFormat.format(current));
}
if(eventOccurred.getSource()== "Save") // If write_to_file is heard, write
{ // whatever is displayed to a .txt file
String save = textBox.getText();
try // Try block for exceptions
{
FileWriter saving = new FileWriter("Log.txt");
saving.write(save);
saving.close();
// So that the User is aware that the data was saved to a file
JOptionPane.showMessageDialog(this, "Everything displayed has been saved in a file named \'Log.txt\'.");
}
catch(Exception menuException) // Catch block for the exceptions thrown
{
textBox.setText("Exception is " + menuException);
}
}
if(eventOccurred.getSource() == "Change Color") // If change_color is selected,
{ // Make the background green.
frame.getContentPane().setBackground(Color.GREEN);
}
if(eventOccurred.getSource() == "Logout") // If exit_application is selected
{ // logout of the application
frame.setVisible(false);
JOptionPane.showMessageDialog(this, "See ya, have a great day!");
}
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Make sure the application closes
}
}

Step by step
Solved in 3 steps

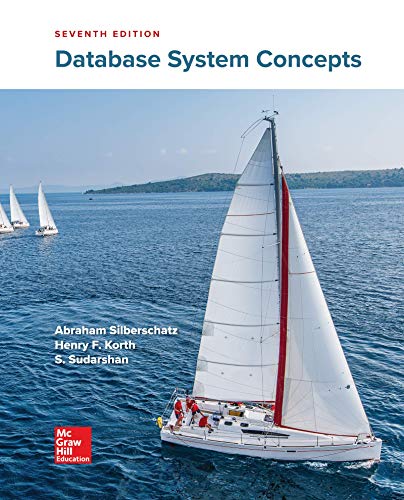
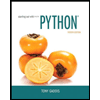
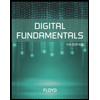
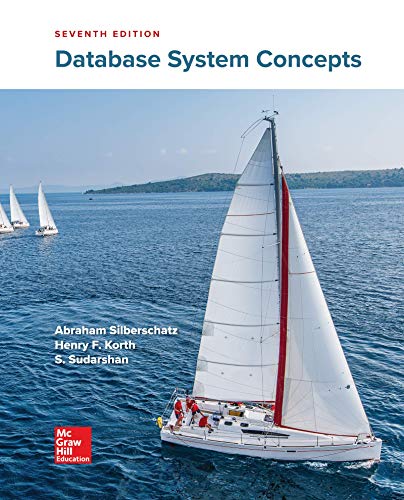
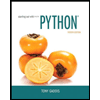
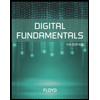
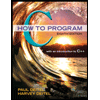
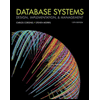
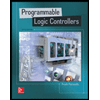