What would pseudocode look like for the following Java application? Java source code: package application; public class JavaConcurrency { private static final Object lock = new Object(); private static boolean isCountingUpDone = false; public static void main(String[] args) { Runnable countUp = () -> { for(int i = 0; i <= 20; i++) { synchronized (lock) { System.out.println("Thread 1: " + i); if (i == 20) { isCountingUpDone = true; lock.notifyAll(); // Notify thread 2 to start counting down System.out.println("\nThread 1 complete\n"); } } try { Thread.sleep(400); // Slow down thread } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } }; Runnable countDown = () -> { synchronized (lock) { while (!isCountingUpDone) { try { lock.wait(); // Wait for thread 1 to finish } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } } for (int i = 20; i >= 0; i--) { System.out.println("thread 2: " + i); try { Thread.sleep(400); // Slow down thread } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } System.out.println("\nThread 2 complete"); }; new Thread(countUp).start(); new Thread(countDown).start(); }}
What would pseudocode look like for the following Java application?
Java source code:
package application;
public class JavaConcurrency {
private static final Object lock = new Object();
private static boolean isCountingUpDone = false;
public static void main(String[] args) {
Runnable countUp = () -> {
for(int i = 0; i <= 20; i++) {
synchronized (lock) {
System.out.println("Thread 1: " + i);
if (i == 20) {
isCountingUpDone = true;
lock.notifyAll(); // Notify thread 2 to start counting down
System.out.println("\nThread 1 complete\n");
}
}
try {
Thread.sleep(400); // Slow down thread
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
};
Runnable countDown = () -> {
synchronized (lock) {
while (!isCountingUpDone) {
try {
lock.wait(); // Wait for thread 1 to finish
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
for (int i = 20; i >= 0; i--) {
System.out.println("thread 2: " + i);
try {
Thread.sleep(400); // Slow down thread
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
System.out.println("\nThread 2 complete");
};
new Thread(countUp).start();
new Thread(countDown).start();
}
}
Unlock instant AI solutions
Tap the button
to generate a solution
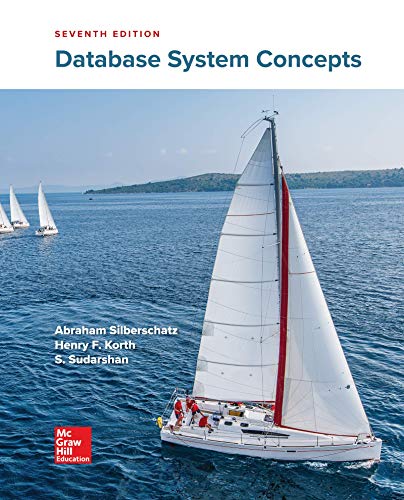
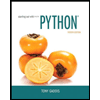
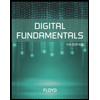
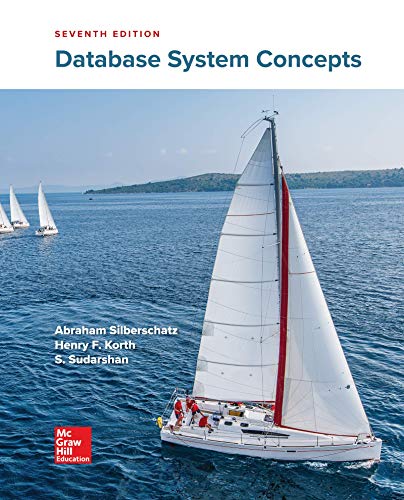
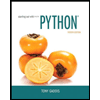
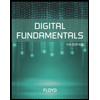
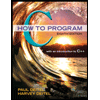
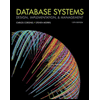
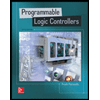