Why does this not work
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Why does this not work

Transcribed Image Text:The image displays an error message output commonly seen in programming environments. Below is a transcription of the text that would be typical for an educational setting:
---
**Java Compiler Error Example: Understanding "Cannot Find Symbol"**
In this example, we observe a common compilation error that occurs when the Java compiler cannot find a referenced variable or method. This typically happens when the variable is either not declared or out of scope. Here is a breakdown of the error output:
```
MyProgram.java:20: error: cannot find symbol
if (rsvp = true && number == 1) {
^
symbol: variable number
location: class MyProgram
MyProgram.java:23: error: cannot find symbol
else if (rsvp = true && number == 2) {
^
symbol: variable number
location: class MyProgram
MyProgram.java:26: error: cannot find symbol
else if (rsvp = true && number == 3) {
^
symbol: variable number
location: class MyProgram
```
**Key Points:**
1. **Error Type**: `cannot find symbol`
- This indicates that the compiler cannot resolve a reference to a variable or method.
2. **Mistake in the Code**:
- The variable `number` is not recognized in the context it's being used. This could be due to it not being declared before use or not being in the scope of the method or class.
3. **Lines with Errors**:
- The errors occur on lines 20, 23, and 26, where the code attempts to evaluate a condition involving `number`.
4. **Probable Causes**:
- The variable `number` might not have been declared in the class `MyProgram`.
- It might be a case of a typo or incorrect variable name.
**Resolution Tips**:
- Check if the variable `number` has been declared appropriately within the class or method.
- Ensure correct spelling and capitalization as Java is case-sensitive.
- Consider the scope of variable `number`; it should be accessible within the class `MyProgram`.
Understanding and fixing such compilation errors is essential for efficient Java programming and debugging.
---
This transcription and explanation provide a foundational understanding of the "cannot find symbol" error, helping learners effectively troubleshoot and resolve similar issues in their code.
![```java
import java.util.Scanner;
public class MyProgram
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("Answer true or false, Are you attending? ");
boolean rsvp = sc.nextBoolean();
if(!rsvp) {
System.out.println("Sorry you can't make it");
}
if(rsvp == true){
System.out.println("Please enter a menu number: ");
int number = sc.nextInt();
if(rsvp == true && number == 1) {
System.out.println("Thanks for attending. You will be served beef.");
}
else if(rsvp == true && number == 2) {
System.out.println("Thanks for attending. You will be served chicken.");
}
else if(rsvp == true && number == 3) {
System.out.println("Thanks for attending. You will be served pasta.");
}
else {
System.out.println("Thank you for attending. You will be served fish.");
}
}
}
}
```
### Description
This Java program, named `MyProgram`, is designed to handle the attendance status and menu preference of a guest. It utilizes the `Scanner` class to accept user input. Here is an explanation of how the program operates:
1. The program begins by outputting a prompt asking whether the user is attending: "Answer true or false, Are you attending?" This expects a boolean (true/false) input.
2. If the response (`rsvp`) is `false`, the program will respond with "Sorry you can't make it" and terminate further operations.
3. If `rsvp` is `true`, the user is prompted to enter a menu number: "Please enter a menu number:".
4. Depending on the number entered (1, 2, or 3), a corresponding message is printed:
- Number 1: "Thanks for attending. You will be served beef."
- Number 2: "Thanks for attending. You will be served chicken."
- Number 3: "Thanks for attending. You will be served pasta."
5. If a different number is entered, the program defaults to: "Thank you for attending. You will be served fish."
This simple program encodes conditional logic to manage event attendance and meal selection based on user input.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F29d9ecf2-8ea2-4c7b-b874-691941710284%2F022d388b-b78f-4998-8c0d-f507d5b434ee%2Fuij865f_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```java
import java.util.Scanner;
public class MyProgram
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("Answer true or false, Are you attending? ");
boolean rsvp = sc.nextBoolean();
if(!rsvp) {
System.out.println("Sorry you can't make it");
}
if(rsvp == true){
System.out.println("Please enter a menu number: ");
int number = sc.nextInt();
if(rsvp == true && number == 1) {
System.out.println("Thanks for attending. You will be served beef.");
}
else if(rsvp == true && number == 2) {
System.out.println("Thanks for attending. You will be served chicken.");
}
else if(rsvp == true && number == 3) {
System.out.println("Thanks for attending. You will be served pasta.");
}
else {
System.out.println("Thank you for attending. You will be served fish.");
}
}
}
}
```
### Description
This Java program, named `MyProgram`, is designed to handle the attendance status and menu preference of a guest. It utilizes the `Scanner` class to accept user input. Here is an explanation of how the program operates:
1. The program begins by outputting a prompt asking whether the user is attending: "Answer true or false, Are you attending?" This expects a boolean (true/false) input.
2. If the response (`rsvp`) is `false`, the program will respond with "Sorry you can't make it" and terminate further operations.
3. If `rsvp` is `true`, the user is prompted to enter a menu number: "Please enter a menu number:".
4. Depending on the number entered (1, 2, or 3), a corresponding message is printed:
- Number 1: "Thanks for attending. You will be served beef."
- Number 2: "Thanks for attending. You will be served chicken."
- Number 3: "Thanks for attending. You will be served pasta."
5. If a different number is entered, the program defaults to: "Thank you for attending. You will be served fish."
This simple program encodes conditional logic to manage event attendance and meal selection based on user input.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
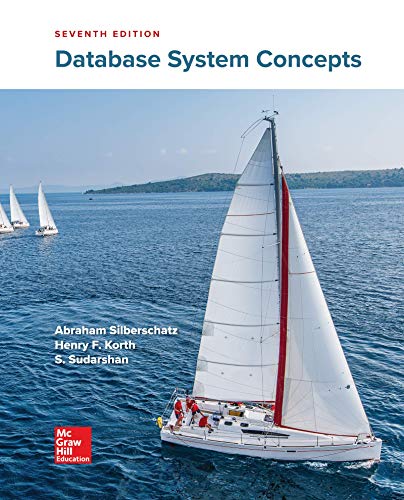
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
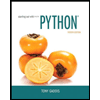
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
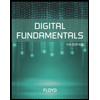
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
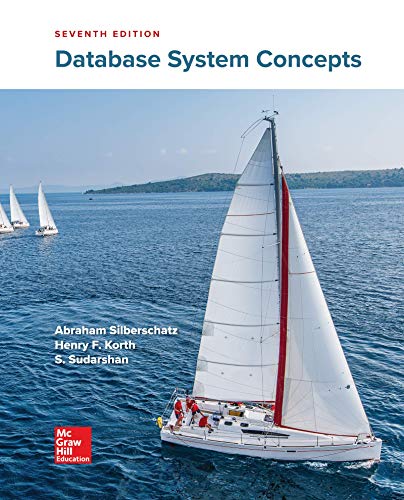
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
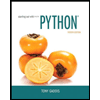
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
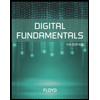
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
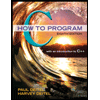
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
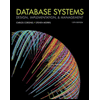
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
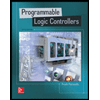
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education