n HexWorld, instead of the decimal (base 10) number system we are used to, the hexadecimal number system is used. The hexadecimal number system uses base 16 with digits 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F, (where A is 10, B is 11, C is 12, D is 13, E is 14, F is 15). To communicate with HexWorld when numbers are involved, we are asking you to help us by coding a Python program, in a file called convert_hex.py, to repeatedly request a positive decimal number from the user and output the hexadecimal value until there are no more decimal values to be converted. We will assume there are no negative values to be converted. To compute the hexadecimal value we have to find the hexadecimal digits hn, hn-1, hn-2, ..., h2, h1, and h0, such that dec_value = hn x 16n + hn-1 x 16n-1 + hn-2 x 16n-2 + ... + h2 x 162 + h1 x 161 + h0 x 160 These hexadecimal digits can be found by successively dividing dec_value by 16 until the quotient is 0; the remainders are h0, h1, ..., hn-1, hn. For example, if dec_value = 589: • 589/16 is 36 with a remainder of 13 where 13 in hexadecimal is 'D' - this is h0 • 36/16 is 2 with a remainder of 4 where 4 in hexadecimal is '4' - this is h1 • 2/16 is 0 with a remainder of 2 where 2 in hexadecimal is '2' - this is h2 So 589 in decimal is 24D in hexadecimal. Your program should include the following functions: • function main() that repeatedly asks the user for a decimal number and, using the functions described below, converts and returns the hexadecimal number and prints the decimal number, as well as the hexadecimal number, until there are no more decimal numbers are to be converted • function decToHex(dec_value) that returns the hexadecimal equivalent of dec_value (as a string) • function getHexChar(dec_digit) that returns the hexadecimal character for dec_digit (note that 0 in decimal would result in ‘0’, 1 in decimal would result in ‘1’, ..., 10 in decimal would result in 'A', 11 in decimal would result in 'B', etc) Sample input/output (input in bold blue): Enter a decimal value, 0 to finish: 589 589 is equal to 24D in hexadecimal. Enter another decimal value, 0 to finish: 321 321 is equal to 141 in hexadecimal. Enter another decimal value, 0 to finish: 256 256 is equal to 100 in hexadecimal. Enter another decimal value, 0 to finish: 1256 1256 is equal to 4E8 in hexadecimal. Enter another decimal value, 0 to finish: 4012 4012 is equal to FAC in hexadecimal. Enter another decimal value, 0 to finish: 0
n HexWorld, instead of the decimal (base 10) number system we are used to, the hexadecimal number system is used. The hexadecimal number system uses base 16 with digits 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F, (where A is 10, B is 11, C is 12, D is 13, E is 14, F is 15). To communicate with HexWorld when numbers are involved, we are asking you to help us by coding a Python program, in a file called convert_hex.py, to repeatedly request a positive decimal number from the user and output the hexadecimal value until there are no more decimal values to be converted. We will assume there are no negative values to be converted. To compute the hexadecimal value we have to find the hexadecimal digits hn, hn-1, hn-2, ..., h2, h1, and h0, such that dec_value = hn x 16n + hn-1 x 16n-1 + hn-2 x 16n-2 + ... + h2 x 162 + h1 x 161 + h0 x 160 These hexadecimal digits can be found by successively dividing dec_value by 16 until the quotient is 0; the remainders are h0, h1, ..., hn-1, hn. For example, if dec_value = 589: • 589/16 is 36 with a remainder of 13 where 13 in hexadecimal is 'D' - this is h0 • 36/16 is 2 with a remainder of 4 where 4 in hexadecimal is '4' - this is h1 • 2/16 is 0 with a remainder of 2 where 2 in hexadecimal is '2' - this is h2 So 589 in decimal is 24D in hexadecimal. Your program should include the following functions: • function main() that repeatedly asks the user for a decimal number and, using the functions described below, converts and returns the hexadecimal number and prints the decimal number, as well as the hexadecimal number, until there are no more decimal numbers are to be converted • function decToHex(dec_value) that returns the hexadecimal equivalent of dec_value (as a string) • function getHexChar(dec_digit) that returns the hexadecimal character for dec_digit (note that 0 in decimal would result in ‘0’, 1 in decimal would result in ‘1’, ..., 10 in decimal would result in 'A', 11 in decimal would result in 'B', etc) Sample input/output (input in bold blue): Enter a decimal value, 0 to finish: 589 589 is equal to 24D in hexadecimal. Enter another decimal value, 0 to finish: 321 321 is equal to 141 in hexadecimal. Enter another decimal value, 0 to finish: 256 256 is equal to 100 in hexadecimal. Enter another decimal value, 0 to finish: 1256 1256 is equal to 4E8 in hexadecimal. Enter another decimal value, 0 to finish: 4012 4012 is equal to FAC in hexadecimal. Enter another decimal value, 0 to finish: 0
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In HexWorld, instead of the decimal (base 10) number system we are used to, the hexadecimal number
system is used. The hexadecimal number system uses base 16 with digits 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E,
F, (where A is 10, B is 11, C is 12, D is 13, E is 14, F is 15). To communicate with HexWorld when numbers are
involved, we are asking you to help us by coding a Python program, in a file called convert_hex.py, to
repeatedly request a positive decimal number from the user and output the hexadecimal value until there are
no more decimal values to be converted. We will assume there are no negative values to be converted.
To compute the hexadecimal value we have to find the hexadecimal digits hn, hn-1, hn-2, ..., h2, h1, and h0, such
that
dec_value = hn x 16n + hn-1 x 16n-1 + hn-2 x 16n-2 + ... + h2 x 162 + h1 x 161 + h0 x 160
These hexadecimal digits can be found by successively dividing dec_value by 16 until the quotient is 0; the
remainders are h0, h1, ..., hn-1, hn.
For example, if dec_value = 589:
• 589/16 is 36 with a remainder of 13 where 13 in hexadecimal is 'D' - this is h0
• 36/16 is 2 with a remainder of 4 where 4 in hexadecimal is '4' - this is h1
• 2/16 is 0 with a remainder of 2 where 2 in hexadecimal is '2' - this is h2
So 589 in decimal is 24D in hexadecimal.
Your program should include the following functions:
• function main() that repeatedly asks the user for a decimal number and, using the functions described
below, converts and returns the hexadecimal number and prints the decimal number, as well as the
hexadecimal number, until there are no more decimal numbers are to be converted
• function decToHex(dec_value) that returns the hexadecimal equivalent of dec_value (as a string)
• function getHexChar(dec_digit) that returns the hexadecimal character for dec_digit (note
that 0 in decimal would result in ‘0’, 1 in decimal would result in ‘1’, ..., 10 in decimal would result in 'A', 11
in decimal would result in 'B', etc)
Sample input/output (input in bold blue):
Enter a decimal value, 0 to finish: 589
589 is equal to 24D in hexadecimal.
Enter another decimal value, 0 to finish: 321
321 is equal to 141 in hexadecimal.
Enter another decimal value, 0 to finish: 256
256 is equal to 100 in hexadecimal.
Enter another decimal value, 0 to finish: 1256
1256 is equal to 4E8 in hexadecimal.
Enter another decimal value, 0 to finish: 4012
4012 is equal to FAC in hexadecimal.
Enter another decimal value, 0 to finish: 0
system is used. The hexadecimal number system uses base 16 with digits 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E,
F, (where A is 10, B is 11, C is 12, D is 13, E is 14, F is 15). To communicate with HexWorld when numbers are
involved, we are asking you to help us by coding a Python program, in a file called convert_hex.py, to
repeatedly request a positive decimal number from the user and output the hexadecimal value until there are
no more decimal values to be converted. We will assume there are no negative values to be converted.
To compute the hexadecimal value we have to find the hexadecimal digits hn, hn-1, hn-2, ..., h2, h1, and h0, such
that
dec_value = hn x 16n + hn-1 x 16n-1 + hn-2 x 16n-2 + ... + h2 x 162 + h1 x 161 + h0 x 160
These hexadecimal digits can be found by successively dividing dec_value by 16 until the quotient is 0; the
remainders are h0, h1, ..., hn-1, hn.
For example, if dec_value = 589:
• 589/16 is 36 with a remainder of 13 where 13 in hexadecimal is 'D' - this is h0
• 36/16 is 2 with a remainder of 4 where 4 in hexadecimal is '4' - this is h1
• 2/16 is 0 with a remainder of 2 where 2 in hexadecimal is '2' - this is h2
So 589 in decimal is 24D in hexadecimal.
Your program should include the following functions:
• function main() that repeatedly asks the user for a decimal number and, using the functions described
below, converts and returns the hexadecimal number and prints the decimal number, as well as the
hexadecimal number, until there are no more decimal numbers are to be converted
• function decToHex(dec_value) that returns the hexadecimal equivalent of dec_value (as a string)
• function getHexChar(dec_digit) that returns the hexadecimal character for dec_digit (note
that 0 in decimal would result in ‘0’, 1 in decimal would result in ‘1’, ..., 10 in decimal would result in 'A', 11
in decimal would result in 'B', etc)
Sample input/output (input in bold blue):
Enter a decimal value, 0 to finish: 589
589 is equal to 24D in hexadecimal.
Enter another decimal value, 0 to finish: 321
321 is equal to 141 in hexadecimal.
Enter another decimal value, 0 to finish: 256
256 is equal to 100 in hexadecimal.
Enter another decimal value, 0 to finish: 1256
1256 is equal to 4E8 in hexadecimal.
Enter another decimal value, 0 to finish: 4012
4012 is equal to FAC in hexadecimal.
Enter another decimal value, 0 to finish: 0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
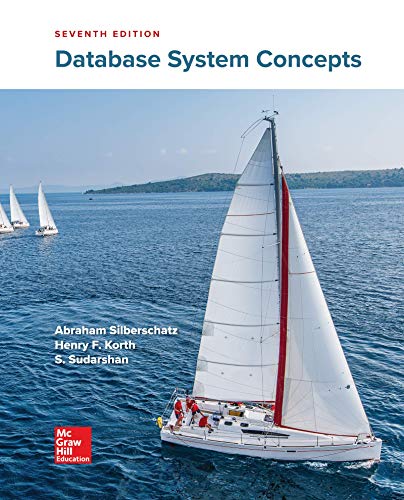
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
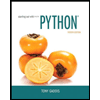
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
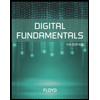
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
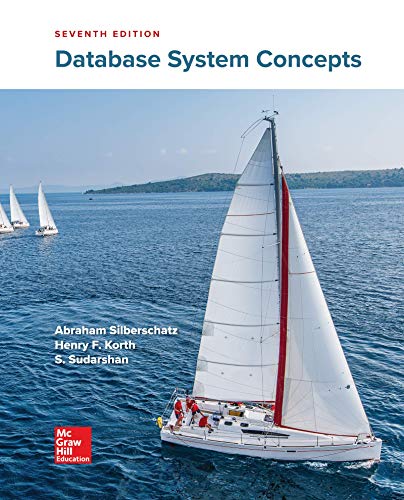
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
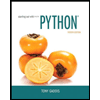
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
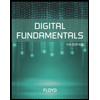
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
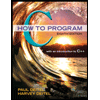
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
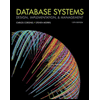
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
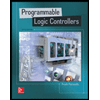
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education