Use a SinglyLinked List to implement a Queue a. Define a Queue interface. b. Define a LinkedQueue class (Node class).. c. Define a Test class to test all methods please help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList { private Node head = null; private Node tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else { tail.setNext(newest); } tail = newest; size++; } public E removeFirst() { if (isEmpty()) { return null; } E firstE = head.getElement(); head = head.getNext(); size--; if(getSize()==0) { tail = null; } return firstE; } public void display() { if (isEmpty()) { System.out.println("Singly linked list is empty."); } else { Node temp = head; System.out.println("=============== begining of lists================"); do { System.out.println( "element =" + temp.getElement()); temp = temp.getNext(); }while (temp != null); System.out.println("============== Ending of lists================="); } } } Secound code package LinkedQueue; public class LinkedQueue implements Queue { private SLinkedList list = new SLinkedList<>(); public LinkedQueue ( ) {} public int size( ) { return list.getSize(); } public boolean isEmpty( ) { return list.isEmpty( ); } public void enqueue (E element) { list.addLast(element); } public E first() { return list.getFirst( ); } public E dequeue() { return list.removeFirst( ); } } third code package LinkedQueue; public interface Queue { int size(); boolean isEmpty( ); void enqueue(E e); E first( ); E dequeue(); }
Use a SinglyLinked List to implement a Queue
a. Define a Queue interface.
b. Define a LinkedQueue class (Node class)..
c. Define a Test class to test all methods
please help me make a test class
first code
third code


Algorithm: Queue using Singly Linked List
1. Create a class SLinkedList for a singly linked list with the following methods:
- Constructor SLinkedList(): Initialize an empty linked list.
- int getSize(): Return the size of the linked list.
- boolean isEmpty(): Check if the linked list is empty.
- E getFirst(): Return the element at the front of the queue.
- E getLast(): Return the element at the rear of the queue.
- void addFirst(E e): Add an element to the front of the queue.
- void addLast(E e): Add an element to the rear of the queue.
- E removeFirst(): Remove and return the element from the front of the queue.
- void display(): Display the elements of the linked list.
2. Create a class Node for the individual nodes of the linked list with the following methods:
- Constructor Node(E e, Node<E> n): Initialize a node with an element and a reference to the next node.
- E getElement(): Return the element stored in the node.
- Node<E> getNext(): Return the reference to the next node.
- void setElement(E e): Set the element of the node.
- void setNext(Node<E> n): Set the reference to the next node.
3. Create an interface Queue<E> with the following methods:
- int size(): Return the size of the queue.
- boolean isEmpty(): Check if the queue is empty.
- void enqueue(E e): Add an element to the rear of the queue.
- E first(): Return the element at the front of the queue.
- E dequeue(): Remove and return the element from the front of the queue.
4. Create a class LinkedQueue<E> that implements the Queue interface. Use an instance of SLinkedList to represent the queue. Implement the methods size(), isEmpty(), enqueue(E e), first(), and dequeue() as follows:
- size(): Call the getSize() method of the SLinkedList instance.
- isEmpty(): Call the isEmpty() method of the SLinkedList instance.
- enqueue(E e): Call the addLast(E e) method of the SLinkedList instance to add an element to the rear of the queue.
- first(): Call the getFirst() method of the SLinkedList instance to return the element at the front of the queue.
- dequeue(): Call the removeFirst() method of the SLinkedList instance to remove and return the element from the front of the queue.
5. Create a Test class (e.g., TestLinkedQueue) to test the LinkedQueue class:
- Instantiate a LinkedQueue object.
- Test enqueue, size, isEmpty, first, and dequeue methods by adding elements to the queue, checking its size, checking if it's empty, getting the first element, and dequeuing elements.
6. Run the Test class to verify the functionality of the Queue implemented using a singly linked list.
Step by step
Solved in 4 steps with 7 images

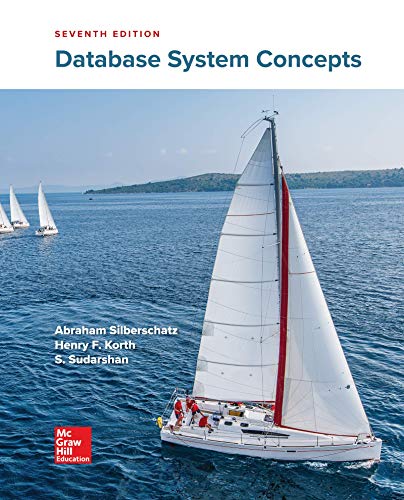
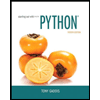
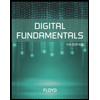
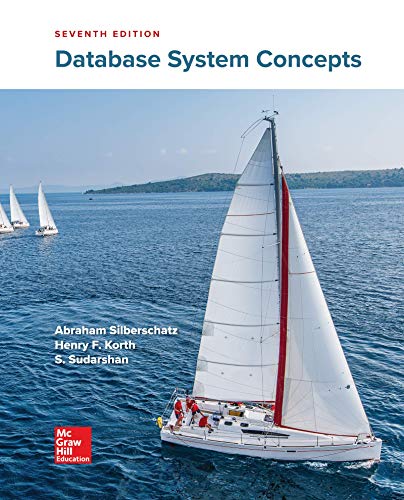
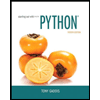
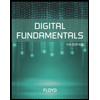
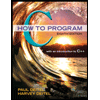
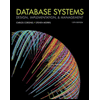
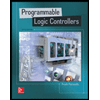