want to turn the code from singly-linked list to doubly-linked list and .Circular-linked list package Problem; class Node{ private Object value; // Holds the data of the node private Node next; // Holds the follwoing node's address Node(Object v, Node n){
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
I want to turn the code from singly-linked list to doubly-linked list and .Circular-linked list
package Problem;
class Node{
private Object value; // Holds the data of the node
private Node next; // Holds the follwoing node's address
Node(Object v, Node n){
this.value =v;
this.next = n;
}
public Node getNext(){
return this.next;
}
public Object getValue(){
return this.value;
}
public void setNext(Node n){
this.next=n;
}
public void setValue(Object v)
{
this.value=v;
}
}
class SLL{
public Node head;
SLL(){
this.head=null;
}
public void display(){
Node curr = this.head;
while(curr != null)
{
System.out.print(curr.getValue());
if(curr.getNext()!=null)
System.out.print("-->");
curr=curr.getNext();
}
System.out.println();
}
public void insertAtFront(Object v){
Node new_node = new Node(v, this.head);
this.head = new_node;
}
public void insertAtEnd(Object v){
Node curr = this.head;
while(curr .getNext()!= null)
curr = curr.getNext();
Node new_node = new Node(v, null);
curr.setNext(new_node);
}
public void insertAfter(Object v, Object key){
Node curr = this.head;
while(curr.getValue() != key)
curr=curr.getNext();
Node new_node = new Node(v, curr.getNext());
curr.setNext(new_node);
}
public void Delete(Object v)
{
if(v == this.head.getValue())
this.head = head.getNext();
else
{
Node curr = this.head;
Node previous = this .head;
while(curr.getValue()!=v)
{
previous = curr;
curr = curr.getNext();
}
previous.setNext(curr.getNext());
}
}
public Node Search(Object v){
Node curr = this.head;
while(curr != null)
{
if(curr.getValue() == v)
return curr;
curr = curr.getNext();
}
return null;
}
//The following function gets the last node in the list
public Node Last(){
Node curr = this.head;
while(curr.getNext()!= null)
curr = curr.getNext();
return curr;
}
// Count the elements of the lsit
public int size(){
Node curr = this.head;
int c=0;
while(curr != null)
{
curr = curr.getNext();
c++;
}
return c;
}
}
public class f {
public static void main(String[] args) {
SLL ls = new SLL();
ls.insertAtFront(1);
ls.insertAtFront(21);
ls.insertAtFront(22);
ls.insertAtFront(3);
ls.display();
//ls.insertAtEnd(33);
//ls.insertAtFront(33);
//ls.insertAfter(33, 22);
//ls.Delete(22);
//Node g = ls.Search(22);
//System.out.println(g.getNext().getValue());
System.out.println(ls.size());
System.out.println(ls.Last().getValue());
//ls.display();
}
}

Step by step
Solved in 2 steps with 1 images

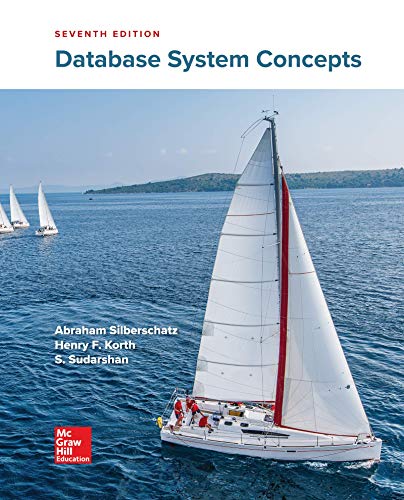
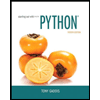
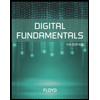
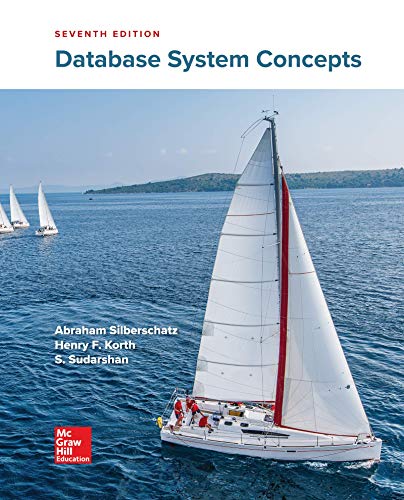
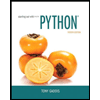
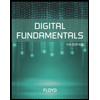
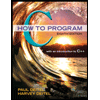
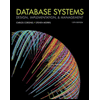
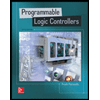