Use a new cell in your notebook 2. Create the following classes in your notebook (paste code into a cell, using proper indentation): class Car(object): def init(self, color='red'): self.color=color class Tire(object): def init(self, miles=0): self.miles = miles def add_miles(self, miles): self.miles += miles 1. Create a class named CustomCar() that is itself, a child-class of car.car 2. Create a class named CustomTire() that is, itself, a child-class of car. Tire 3. Override the class constructor Customcar() has inherited from Car() and, in it, call the car() constructor. To do this, you must call the car.Car() constructor as a class method and not as an instance method. A snippet containing this construction is as below: below: car.car._init__(self, color) This calls the constructor to do its work but instead of creating a new instance of car.car() we pass it the current instance of CustonCar() as self 4. Add an additional argument named tires to the Customcar() constructor 4. Add an additional argument named, tires to the Customcar() constructor. 1. Generally, this should be a list of CustomTire() objects ii. The default of this argument should be None 5. Add a new instance attribute to CustomCar() called tires to store a list of tires 1. Assign the new instance attribute the value of the tires argument ii. If the value of the tires argument is None : a. Create a list b. Create four new instances of the CustomTire() class and append each into the list 6. Add a pseudo-private class attribute to the customTires() class called maximum_miles and assign it a value of 580. 7. Test that your customcar() class works with both a tires argument and without. Expected Output >>> mycar = CustomCar() >>> len(mycar. tires) >>> isinstance(mycar.tires[0], CustomTire) True Code should be submitted via Blackboard.
Use a new cell in your notebook 2. Create the following classes in your notebook (paste code into a cell, using proper indentation): class Car(object): def init(self, color='red'): self.color=color class Tire(object): def init(self, miles=0): self.miles = miles def add_miles(self, miles): self.miles += miles 1. Create a class named CustomCar() that is itself, a child-class of car.car 2. Create a class named CustomTire() that is, itself, a child-class of car. Tire 3. Override the class constructor Customcar() has inherited from Car() and, in it, call the car() constructor. To do this, you must call the car.Car() constructor as a class method and not as an instance method. A snippet containing this construction is as below: below: car.car._init__(self, color) This calls the constructor to do its work but instead of creating a new instance of car.car() we pass it the current instance of CustonCar() as self 4. Add an additional argument named tires to the Customcar() constructor
4. Add an additional argument named, tires to the Customcar() constructor. 1. Generally, this should be a list of CustomTire() objects ii. The default of this argument should be None 5. Add a new instance attribute to CustomCar() called tires to store a list of tires 1. Assign the new instance attribute the value of the tires argument ii. If the value of the tires argument is None : a. Create a list b. Create four new instances of the CustomTire() class and append each into the list 6. Add a pseudo-private class attribute to the customTires() class called maximum_miles and assign it a value of 580. 7. Test that your customcar() class works with both a tires argument and without. Expected Output >>> mycar = CustomCar() >>> len(mycar. tires) >>> isinstance(mycar.tires[0], CustomTire) True Code should be submitted via Blackboard.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

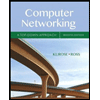
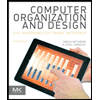
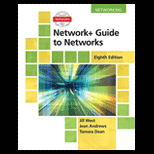
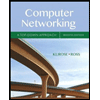
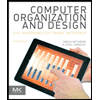
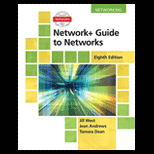
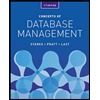
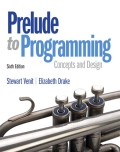
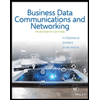