Use a member initialization list to make the TeamInfo constructor initialize the vector listOfPointsInSeason with a size of 6. Note: Including a vector in an initialization list causes that vector's constructor to be called with the value in the parens. #include #include using namespace std; class TeamInfo { public: TeamInfo(); void PrintGamesInSeason() const; private: vector listOfPointsInSeason; };
Use a member initialization list to make the TeamInfo constructor initialize the
#include <iostream>
#include <vector>
using namespace std;
class TeamInfo {
public:
TeamInfo();
void PrintGamesInSeason() const;
private:
vector<int> listOfPointsInSeason;
};
TeamInfo::TeamInfo() : /* Your code goes here */ {
}
void TeamInfo::PrintGamesInSeason() const {
cout << "There are " << listOfPointsInSeason.size() << " games in a season." << endl;
}
int main() {
TeamInfo myTeam;
myTeam.PrintGamesInSeason();
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

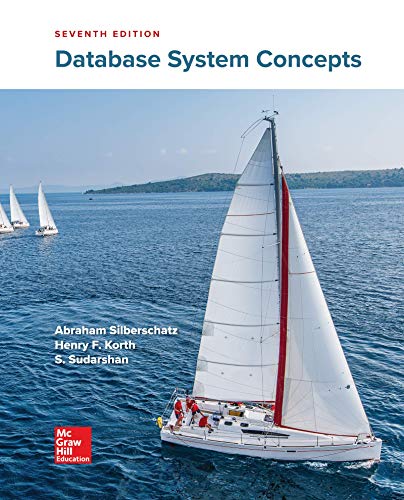
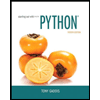
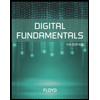
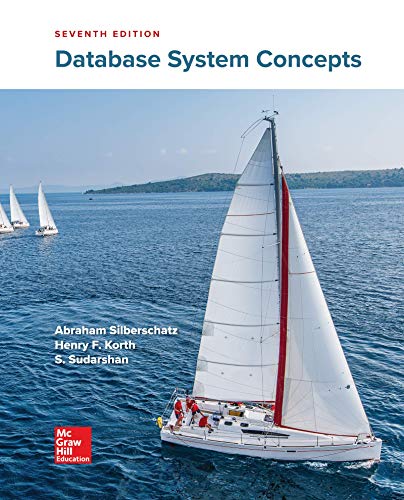
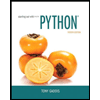
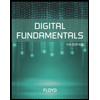
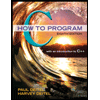
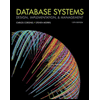
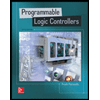