URGENT: Python Expense Management: This system will add, deduct, update, sort, and export expenses to a file. The program will initially load a collection of expenses from a .txt file and store them in a dictionary. All of the necessary functions and unit testing are provided below in expenses.py, expenses_test.py, and expenses.txt: expnses.py: def import_expenses(file): '''Reads data from a given file and stores the expenses in a dictionary, where the expense type is the key and the total expense amount for that expense is the value. The same expense type may appear multiple times in the file. Ignores expenses with missing or invalid amounts.''' # create empty dict expenses = {} #TODO insert your code return expenses def get_expense(expenses, expense_type): '''Returns the value for the given expense type in the given expenses dictionary. Prints a friendly message and returns None if the expense type doesn't exist.''' # TODO insert your code def add_expense(expenses, expense_type, value): '''Adds the given expense type & value to the given expenses dictionary. If the expense type exists, adds the value to the total amount. Otherwise, creates a new expense type with the value. Prints the expense.''' # TODO insert your code def deduct_expense(expenses, expense_type, value): '''Deducts the given value from the given expense type in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Raises a RuntimeError if the value is greater than the existing total of the expense type. Prints the expense.''' # TODO insert your code def update_expense(expenses, expense_type, value): '''Updates the given expense type with the given value in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Prints the expense.''' # TODO insert your code def sort_expenses(expenses, sorting): '''Converts the key:value pairs in the given expenses dictionary to a list of tuples and sorts based on the given sorting argument. If the sorting argument is the string 'expense_type', sorts the list of tuples based on the expense type (e.g. 'rent'), otherwise, if the sorting argument is 'amount', sorts the list based on the total expense amount (e.g. 825) in descending order.''' # TODO insert your code def export_expenses(expenses, expense_types, file): '''Exports the given expense types from the given expenses dictionary to the given file. Iterates over the given expenses dictionary, filters based on the given expense types (a list of strings), and exports to a file.''' # TODO insert your code def main(): #import expense file and store in dictionary expenses = import_expenses('expenses.txt') #for testing purposes #print(expenses) while True: #print welcome and options print('\nWelcome to the expense management system! What would you like to do?') print('1: Get expense info') print('2: Add an expense') print('3: Deduct an expense') print('4: Sort expenses') print('5: Export expenses') print('0: Exit the system') #get user input option_input = input('\n') #try and cast to int try: option = int(option_input) #catch ValueError except ValueError: print("Invalid option.") else: #check options if (option == 1): #get expense type & print expense info expense_type = input('Expense type? ') print(get_expense(expenses, expense_type)) elif (option == 2): #get expense type expense_type = input('Expense type? ') #get amount to add and cast to float amount = float(input('Amount to add? ')) #add expense add_expense(expenses, expense_type, amount) elif (option == 3): #get expense type expense_type = input('Expense type? ') #get amount to deduct and cast to float amount = float(input('Amount to deduct? ')) #deduct expense deduct_expense(expenses, expense_type, amount) elif (option == 4): #get sort type sort_type = input('What type of sort? (\'expense_type\' or \'amount\')') #sort expenses print(sort_expenses(expenses, sort_type)) elif (option == 5): # get filename to export to file_name = input('Name of file to export to?') # get expense types to export expense_types = [] while True: expense_type = input("What expense type you want to export? Input N to quit:") if expense_type == "N": break expense_types.append(expense_type) # export expenses export_expenses(expenses, expense_types, file_name) elif (option == 0): #exit expense system print('Good bye!') break if __name__ == '__main__': main() Example expenses.txt provided: food: 5 coffee: 1.89 phone: rent: 825 clothes: 45 entertainment: 10 coffee: 3.25 entertainment: 125.62 coffee: 2.43 expenses_test.py: (INCLUDE BOTH NORMAL AND EDGE CASES) import unittest from expenses import * class Expenses_Test(unittest.TestCase): def setUp(self): """The setUp function runs before every test function.""" #load expenses file self.expenses = import_expenses('expenses.txt') def test_import_expenses(self): #test existing total expenses self.assertAlmostEqual(45, self.expenses['clothes']) self.assertAlmostEqual(7.57, self.expenses['coffee']) self.assertAlmostEqual(135.62, self.expenses['entertainment']) def test_get_expense(self): #test getting expenses based on expense type self.assertAlmostEqual(7.57, get_expense(self.expenses, "coffee")) self.assertAlmostEqual(5, get_expense(self.expenses, "food")) # TODO insert 2 additional test cases # Hint(s): Test non-existing expense types def test_add_expense(self): #test adding a new expense add_expense(self.expenses, "fios", 84.5) self.assertAlmostEqual(84.5, self.expenses.get("fios")) # TODO insert 2 additional test cases # Hint(s): Test adding to existing expenses def test_deduct_expense(self): # test deducting from expense deduct_expense(self.expenses, "coffee", .99) self.assertAlmostEqual(6.58, self.expenses.get("coffee")) # test deducting from expense deduct_expense(self.expenses, "entertainment", 100) self.assertAlmostEqual(35.62, self.expenses.get("entertainment")) # TODO insert 2 additional test cases # Hint(s): # Test deducting too much from expense # Test deducting from non-existing expense def test_update_expense(self): #test updating an expense update_expense(self.expenses, "clothes", 19.99) self.assertAlmostEqual(19.99, get_expense(self.expenses, "clothes")) # TODO insert 2 additional test cases # Hint(s): # Test updating an expense # Test updating a non-existing expense def test_sort_expenses(self): #test sorting expenses by 'expense_type' expense_type_sorted_expenses = [('clothes', 45.0), ('coffee', 7.57), ('entertainment', 135.62), ('food', 5.0), ('rent', 825.0)] self.assertListEqual(expense_type_sorted_expenses, sort_expenses(self.expenses, "expense_type")) # TODO insert 1 additional test case # Hint: Test sorting expenses by 'amount' if __name__ == '__main__': unittest.main() Thank you and please leave comments with any questions!
URGENT: Python Expense Management: This system will add, deduct, update, sort, and export expenses to a file. The program will initially load a collection of expenses from a .txt file and store them in a dictionary. All of the necessary functions and unit testing are provided below in expenses.py, expenses_test.py, and expenses.txt: expnses.py: def import_expenses(file): '''Reads data from a given file and stores the expenses in a dictionary, where the expense type is the key and the total expense amount for that expense is the value. The same expense type may appear multiple times in the file. Ignores expenses with missing or invalid amounts.''' # create empty dict expenses = {} #TODO insert your code return expenses def get_expense(expenses, expense_type): '''Returns the value for the given expense type in the given expenses dictionary. Prints a friendly message and returns None if the expense type doesn't exist.''' # TODO insert your code def add_expense(expenses, expense_type, value): '''Adds the given expense type & value to the given expenses dictionary. If the expense type exists, adds the value to the total amount. Otherwise, creates a new expense type with the value. Prints the expense.''' # TODO insert your code def deduct_expense(expenses, expense_type, value): '''Deducts the given value from the given expense type in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Raises a RuntimeError if the value is greater than the existing total of the expense type. Prints the expense.''' # TODO insert your code def update_expense(expenses, expense_type, value): '''Updates the given expense type with the given value in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Prints the expense.''' # TODO insert your code def sort_expenses(expenses, sorting): '''Converts the key:value pairs in the given expenses dictionary to a list of tuples and sorts based on the given sorting argument. If the sorting argument is the string 'expense_type', sorts the list of tuples based on the expense type (e.g. 'rent'), otherwise, if the sorting argument is 'amount', sorts the list based on the total expense amount (e.g. 825) in descending order.''' # TODO insert your code def export_expenses(expenses, expense_types, file): '''Exports the given expense types from the given expenses dictionary to the given file. Iterates over the given expenses dictionary, filters based on the given expense types (a list of strings), and exports to a file.''' # TODO insert your code def main(): #import expense file and store in dictionary expenses = import_expenses('expenses.txt') #for testing purposes #print(expenses) while True: #print welcome and options print('\nWelcome to the expense management system! What would you like to do?') print('1: Get expense info') print('2: Add an expense') print('3: Deduct an expense') print('4: Sort expenses') print('5: Export expenses') print('0: Exit the system') #get user input option_input = input('\n') #try and cast to int try: option = int(option_input) #catch ValueError except ValueError: print("Invalid option.") else: #check options if (option == 1): #get expense type & print expense info expense_type = input('Expense type? ') print(get_expense(expenses, expense_type)) elif (option == 2): #get expense type expense_type = input('Expense type? ') #get amount to add and cast to float amount = float(input('Amount to add? ')) #add expense add_expense(expenses, expense_type, amount) elif (option == 3): #get expense type expense_type = input('Expense type? ') #get amount to deduct and cast to float amount = float(input('Amount to deduct? ')) #deduct expense deduct_expense(expenses, expense_type, amount) elif (option == 4): #get sort type sort_type = input('What type of sort? (\'expense_type\' or \'amount\')') #sort expenses print(sort_expenses(expenses, sort_type)) elif (option == 5): # get filename to export to file_name = input('Name of file to export to?') # get expense types to export expense_types = [] while True: expense_type = input("What expense type you want to export? Input N to quit:") if expense_type == "N": break expense_types.append(expense_type) # export expenses export_expenses(expenses, expense_types, file_name) elif (option == 0): #exit expense system print('Good bye!') break if __name__ == '__main__': main() Example expenses.txt provided: food: 5 coffee: 1.89 phone: rent: 825 clothes: 45 entertainment: 10 coffee: 3.25 entertainment: 125.62 coffee: 2.43 expenses_test.py: (INCLUDE BOTH NORMAL AND EDGE CASES) import unittest from expenses import * class Expenses_Test(unittest.TestCase): def setUp(self): """The setUp function runs before every test function.""" #load expenses file self.expenses = import_expenses('expenses.txt') def test_import_expenses(self): #test existing total expenses self.assertAlmostEqual(45, self.expenses['clothes']) self.assertAlmostEqual(7.57, self.expenses['coffee']) self.assertAlmostEqual(135.62, self.expenses['entertainment']) def test_get_expense(self): #test getting expenses based on expense type self.assertAlmostEqual(7.57, get_expense(self.expenses, "coffee")) self.assertAlmostEqual(5, get_expense(self.expenses, "food")) # TODO insert 2 additional test cases # Hint(s): Test non-existing expense types def test_add_expense(self): #test adding a new expense add_expense(self.expenses, "fios", 84.5) self.assertAlmostEqual(84.5, self.expenses.get("fios")) # TODO insert 2 additional test cases # Hint(s): Test adding to existing expenses def test_deduct_expense(self): # test deducting from expense deduct_expense(self.expenses, "coffee", .99) self.assertAlmostEqual(6.58, self.expenses.get("coffee")) # test deducting from expense deduct_expense(self.expenses, "entertainment", 100) self.assertAlmostEqual(35.62, self.expenses.get("entertainment")) # TODO insert 2 additional test cases # Hint(s): # Test deducting too much from expense # Test deducting from non-existing expense def test_update_expense(self): #test updating an expense update_expense(self.expenses, "clothes", 19.99) self.assertAlmostEqual(19.99, get_expense(self.expenses, "clothes")) # TODO insert 2 additional test cases # Hint(s): # Test updating an expense # Test updating a non-existing expense def test_sort_expenses(self): #test sorting expenses by 'expense_type' expense_type_sorted_expenses = [('clothes', 45.0), ('coffee', 7.57), ('entertainment', 135.62), ('food', 5.0), ('rent', 825.0)] self.assertListEqual(expense_type_sorted_expenses, sort_expenses(self.expenses, "expense_type")) # TODO insert 1 additional test case # Hint: Test sorting expenses by 'amount' if __name__ == '__main__': unittest.main() Thank you and please leave comments with any questions!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
URGENT: Python Expense Management:
This system will add, deduct, update, sort, and export expenses to a file. The program will initially load a collection of expenses from a .txt file and store them in a dictionary. All of the necessary functions and unit testing are provided below in expenses.py, expenses_test.py, and expenses.txt:
expnses.py:
def import_expenses(file):
'''Reads data from a given file and stores the expenses in a dictionary, where the expense type is the key and the total expense amount for that expense is the value. The same expense type may appear multiple times in the file. Ignores expenses with missing or invalid amounts.'''
# create empty dict
expenses = {}
#TODO insert your code
return expenses
def get_expense(expenses, expense_type):
'''Returns the value for the given expense type in the given expenses dictionary. Prints a friendly message and returns None if the expense type doesn't exist.'''
# TODO insert your code
def add_expense(expenses, expense_type, value):
'''Adds the given expense type & value to the given expenses dictionary. If the expense type exists, adds the value to the total amount. Otherwise, creates a new expense type with the value. Prints the expense.'''
# TODO insert your code
def deduct_expense(expenses, expense_type, value):
'''Deducts the given value from the given expense type in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Raises a RuntimeError if the value is greater than the existing total of the expense type. Prints the expense.'''
# TODO insert your code
def update_expense(expenses, expense_type, value):
'''Updates the given expense type with the given value in the given expenses dictionary. Prints a friendly message if the expense type doesn't exist. Prints the expense.'''
# TODO insert your code
def sort_expenses(expenses, sorting):
'''Converts the key:value pairs in the given expenses dictionary to a list of tuples and sorts based on the given sorting argument. If the sorting argument is the string 'expense_type', sorts the list of tuples based on the expense type (e.g. 'rent'), otherwise, if the sorting argument is 'amount', sorts the list based on the total expense amount (e.g. 825) in descending order.'''
# TODO insert your code
def export_expenses(expenses, expense_types, file):
'''Exports the given expense types from the given expenses dictionary to the given file. Iterates over the given expenses dictionary, filters based on the given expense types (a list of strings), and exports to a file.'''
# TODO insert your code
def main():
#import expense file and store in dictionary expenses = import_expenses('expenses.txt')
#for testing purposes
#print(expenses)
while True:
#print welcome and options
print('\nWelcome to the expense management system! What would you like to do?')
print('1: Get expense info')
print('2: Add an expense')
print('3: Deduct an expense')
print('4: Sort expenses')
print('5: Export expenses')
print('0: Exit the system')
#get user input
option_input = input('\n')
#try and cast to int
try:
option = int(option_input)
#catch ValueError
except ValueError:
print("Invalid option.")
else:
#check options
if (option == 1):
#get expense type & print expense info
expense_type = input('Expense type? ')
print(get_expense(expenses, expense_type))
elif (option == 2):
#get expense type
expense_type = input('Expense type? ')
#get amount to add and cast to float
amount = float(input('Amount to add? '))
#add expense
add_expense(expenses, expense_type, amount)
elif (option == 3):
#get expense type
expense_type = input('Expense type? ')
#get amount to deduct and cast to float
amount = float(input('Amount to deduct? '))
#deduct expense
deduct_expense(expenses, expense_type, amount)
elif (option == 4):
#get sort type
sort_type = input('What type of sort? (\'expense_type\' or \'amount\')')
#sort expenses
print(sort_expenses(expenses, sort_type))
elif (option == 5):
# get filename to export to
file_name = input('Name of file to export to?')
# get expense types to export
expense_types = []
while True:
expense_type = input("What expense type you want to export? Input N to quit:")
if expense_type == "N":
break
expense_types.append(expense_type)
# export expenses
export_expenses(expenses, expense_types, file_name)
elif (option == 0):
#exit expense system
print('Good bye!')
break
if __name__ == '__main__':
main()
Example expenses.txt provided:
food: 5
coffee: 1.89
phone:
rent: 825
clothes: 45
entertainment: 10
coffee: 3.25
entertainment: 125.62
coffee: 2.43
expenses_test.py: (INCLUDE BOTH NORMAL AND EDGE CASES)
import unittest
from expenses import *
class Expenses_Test(unittest.TestCase):
def setUp(self):
"""The setUp function runs before every test function."""
#load expenses file
self.expenses = import_expenses('expenses.txt')
def test_import_expenses(self):
#test existing total expenses
self.assertAlmostEqual(45, self.expenses['clothes'])
self.assertAlmostEqual(7.57, self.expenses['coffee'])
self.assertAlmostEqual(135.62, self.expenses['entertainment'])
def test_get_expense(self):
#test getting expenses based on expense type
self.assertAlmostEqual(7.57, get_expense(self.expenses, "coffee"))
self.assertAlmostEqual(5, get_expense(self.expenses, "food"))
# TODO insert 2 additional test cases
# Hint(s): Test non-existing expense types
def test_add_expense(self):
#test adding a new expense
add_expense(self.expenses, "fios", 84.5)
self.assertAlmostEqual(84.5, self.expenses.get("fios"))
# TODO insert 2 additional test cases
# Hint(s): Test adding to existing expenses
def test_deduct_expense(self):
# test deducting from expense
deduct_expense(self.expenses, "coffee", .99)
self.assertAlmostEqual(6.58, self.expenses.get("coffee"))
# test deducting from expense
deduct_expense(self.expenses, "entertainment", 100)
self.assertAlmostEqual(35.62, self.expenses.get("entertainment"))
# TODO insert 2 additional test cases
# Hint(s):
# Test deducting too much from expense
# Test deducting from non-existing expense
def test_update_expense(self):
#test updating an expense
update_expense(self.expenses, "clothes", 19.99)
self.assertAlmostEqual(19.99, get_expense(self.expenses, "clothes"))
# TODO insert 2 additional test cases
# Hint(s):
# Test updating an expense
# Test updating a non-existing expense
def test_sort_expenses(self):
#test sorting expenses by 'expense_type'
expense_type_sorted_expenses = [('clothes', 45.0),
('coffee', 7.57),
('entertainment', 135.62),
('food', 5.0),
('rent', 825.0)]
self.assertListEqual(expense_type_sorted_expenses, sort_expenses(self.expenses, "expense_type"))
# TODO insert 1 additional test case
# Hint: Test sorting expenses by 'amount'
if __name__ == '__main__':
unittest.main()
Thank you and please leave comments with any questions!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
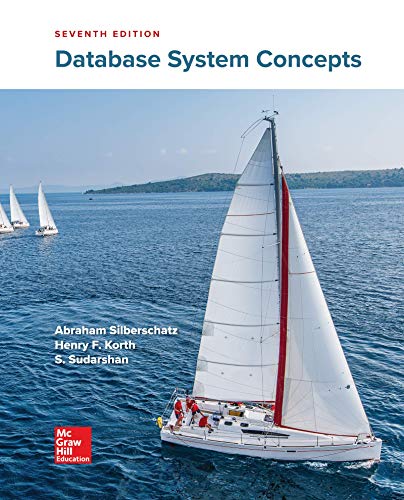
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
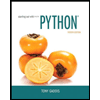
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
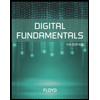
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
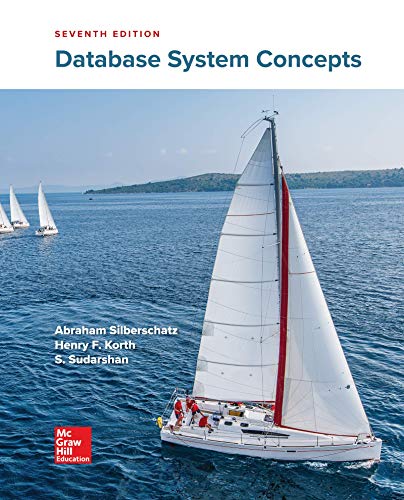
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
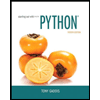
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
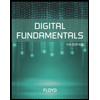
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
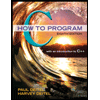
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
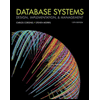
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
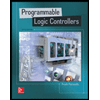
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education