Try to do asap Complete the C code depends on the comments. #include #include // big_integer structure struct two_quadwords{ unsigned long lsq; long msq; }; // big_integer typedef typedef struct two_quadwords big_integer; // Union to store big integers as 16 characters or two quadwords union value{ big_integer binary; char hex[16]; }; // Prototypes of the methods to manipulate big integers // reads a big integer from string input and stores it in the union v // returns 0 if the hexadecimal number is invalid, 1 otherwise int read_big_integer(union value *v, char *input); // writes the value of b to standard output as 32 hex characters void write_big_integer(union value b); // performs b1 & b2 and stores the result in b1_and_b2 void and_big_integers(big_integer b1, big_integer b2, big_integer *b1_and_b2); // performs b1 | b2 and stores the result in b1_or_b2 void or_big_integers(big_integer b1, big_integer b2, big_integer *b1_or_b2); // performs b1 ^ b2 and stores the result in b1_xor_b2 void xor_big_integers(big_integer b1, big_integer b2, big_integer *b1_xor_b2); // performs ~b and stores the result in b void not_big_integer(big_integer *b); // performs b << k and stores the result in b void shift_big_integer_left(big_integer *b, unsigned k); // performs b >> k and stores the result in b void shift_big_integer_right(big_integer *b, unsigned k); // performs b1+b2 and stores the result in sum // returns 1 if overflow occurs, 0 otherwise int add_big_integers(big_integer b1, big_integer b2, big_integer *sum); // prints the message and value followed by the program usage message void print_usage(const char *message, const char* value); // main method int main(int argc, char* argv[]){ if(argc < 3 || argc > 4){ print_usage("Too many or too few arguments", ""); return 0; } if(argc == 3){ // NOT operation (3 arguments) if(strcmp(argv[1], "not")){ // check if operation is 'not' } return 0; } if(argc == 4){ //and, or, xor, sl, sr, add operations (4 arguments) } return 0; } // reads 32 hex characters // returns 0 if the hexadecimal number is invalid, 1 otherwise int read_big_integer(union value *v, char *input){ return 0; } // writes the 32 hex characters b to standard output void write_big_integer(union value b){ } // performs b1 & b2 and stores the result in b1_and_b2 void and_big_integers(big_integer b1, big_integer b2, big_integer *b1_and_b2){ } // performs b1 | b2 and stores the result in b1_or_b2 void or_big_integers(big_integer b1, big_integer b2, big_integer *b1_or_b2){ } // performs b1 ^ b2 and stores the result in b1_xor_b2 void xor_big_integers(big_integer b1, big_integer b2, big_integer *b1_xor_b2){ } // performs ~b and stores the result in b void not_big_integer(big_integer *b){ } // performs b << k and stores the result in b void shift_big_integer_left(big_integer *b, unsigned k){ } // performs b >> k and stores the result in b void shift_big_integer_right(big_integer *b, unsigned k){ } // performs b1+b2 and stores the result in sum // returns 1 if overflow occurs, 0 otherwise int add_big_integers(big_integer b1, big_integer b2, big_integer *sum){ return 0; } // prints the message and value followed by the program usage message void print_usage(const char *message, const char* value){ printf("%s %s\n", message, value); }
Try to do asap Complete the C code depends on the comments. #include #include // big_integer structure struct two_quadwords{ unsigned long lsq; long msq; }; // big_integer typedef typedef struct two_quadwords big_integer; // Union to store big integers as 16 characters or two quadwords union value{ big_integer binary; char hex[16]; }; // Prototypes of the methods to manipulate big integers // reads a big integer from string input and stores it in the union v // returns 0 if the hexadecimal number is invalid, 1 otherwise int read_big_integer(union value *v, char *input); // writes the value of b to standard output as 32 hex characters void write_big_integer(union value b); // performs b1 & b2 and stores the result in b1_and_b2 void and_big_integers(big_integer b1, big_integer b2, big_integer *b1_and_b2); // performs b1 | b2 and stores the result in b1_or_b2 void or_big_integers(big_integer b1, big_integer b2, big_integer *b1_or_b2); // performs b1 ^ b2 and stores the result in b1_xor_b2 void xor_big_integers(big_integer b1, big_integer b2, big_integer *b1_xor_b2); // performs ~b and stores the result in b void not_big_integer(big_integer *b); // performs b << k and stores the result in b void shift_big_integer_left(big_integer *b, unsigned k); // performs b >> k and stores the result in b void shift_big_integer_right(big_integer *b, unsigned k); // performs b1+b2 and stores the result in sum // returns 1 if overflow occurs, 0 otherwise int add_big_integers(big_integer b1, big_integer b2, big_integer *sum); // prints the message and value followed by the program usage message void print_usage(const char *message, const char* value); // main method int main(int argc, char* argv[]){ if(argc < 3 || argc > 4){ print_usage("Too many or too few arguments", ""); return 0; } if(argc == 3){ // NOT operation (3 arguments) if(strcmp(argv[1], "not")){ // check if operation is 'not' } return 0; } if(argc == 4){ //and, or, xor, sl, sr, add operations (4 arguments) } return 0; } // reads 32 hex characters // returns 0 if the hexadecimal number is invalid, 1 otherwise int read_big_integer(union value *v, char *input){ return 0; } // writes the 32 hex characters b to standard output void write_big_integer(union value b){ } // performs b1 & b2 and stores the result in b1_and_b2 void and_big_integers(big_integer b1, big_integer b2, big_integer *b1_and_b2){ } // performs b1 | b2 and stores the result in b1_or_b2 void or_big_integers(big_integer b1, big_integer b2, big_integer *b1_or_b2){ } // performs b1 ^ b2 and stores the result in b1_xor_b2 void xor_big_integers(big_integer b1, big_integer b2, big_integer *b1_xor_b2){ } // performs ~b and stores the result in b void not_big_integer(big_integer *b){ } // performs b << k and stores the result in b void shift_big_integer_left(big_integer *b, unsigned k){ } // performs b >> k and stores the result in b void shift_big_integer_right(big_integer *b, unsigned k){ } // performs b1+b2 and stores the result in sum // returns 1 if overflow occurs, 0 otherwise int add_big_integers(big_integer b1, big_integer b2, big_integer *sum){ return 0; } // prints the message and value followed by the program usage message void print_usage(const char *message, const char* value){ printf("%s %s\n", message, value); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Try to do asap
Complete the C code depends on the comments.
#include <stdio.h> | |
#include <string.h> | |
// big_integer structure | |
struct two_quadwords{ | |
unsigned long lsq; | |
long msq; | |
}; | |
// big_integer typedef | |
typedef struct two_quadwords big_integer; | |
// Union to store big integers as 16 characters or two quadwords | |
union value{ | |
big_integer binary; | |
char hex[16]; | |
}; | |
// Prototypes of the methods to manipulate big integers | |
// reads a big integer from string input and stores it in the union v | |
// returns 0 if the hexadecimal number is invalid, 1 otherwise | |
int read_big_integer(union value *v, char *input); | |
// writes the value of b to standard output as 32 hex characters | |
void write_big_integer(union value b); | |
// performs b1 & b2 and stores the result in b1_and_b2 | |
void and_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_and_b2); | |
// performs b1 | b2 and stores the result in b1_or_b2 | |
void or_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_or_b2); | |
// performs b1 ^ b2 and stores the result in b1_xor_b2 | |
void xor_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_xor_b2); | |
// performs ~b and stores the result in b | |
void not_big_integer(big_integer *b); | |
// performs b << k and stores the result in b | |
void shift_big_integer_left(big_integer *b, unsigned k); | |
// performs b >> k and stores the result in b | |
void shift_big_integer_right(big_integer *b, unsigned k); | |
// performs b1+b2 and stores the result in sum | |
// returns 1 if overflow occurs, 0 otherwise | |
int add_big_integers(big_integer b1, big_integer b2, big_integer *sum); | |
// prints the message and value followed by the program usage message | |
void print_usage(const char *message, const char* value); | |
// main method | |
int main(int argc, char* argv[]){ | |
if(argc < 3 || argc > 4){ | |
print_usage("Too many or too few arguments", ""); | |
return 0; | |
} | |
if(argc == 3){ // NOT operation (3 arguments) | |
if(strcmp(argv[1], "not")){ // check if operation is 'not' | |
} | |
return 0; | |
} | |
if(argc == 4){ //and, or, xor, sl, sr, add operations (4 arguments) | |
} | |
return 0; | |
} | |
// reads 32 hex characters | |
// returns 0 if the hexadecimal number is invalid, 1 otherwise | |
int read_big_integer(union value *v, char *input){ | |
return 0; | |
} | |
// writes the 32 hex characters b to standard output | |
void write_big_integer(union value b){ | |
} | |
// performs b1 & b2 and stores the result in b1_and_b2 | |
void and_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_and_b2){ | |
} | |
// performs b1 | b2 and stores the result in b1_or_b2 | |
void or_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_or_b2){ | |
} | |
// performs b1 ^ b2 and stores the result in b1_xor_b2 | |
void xor_big_integers(big_integer b1, big_integer b2, | |
big_integer *b1_xor_b2){ | |
} | |
// performs ~b and stores the result in b | |
void not_big_integer(big_integer *b){ | |
} | |
// performs b << k and stores the result in b | |
void shift_big_integer_left(big_integer *b, unsigned k){ | |
} | |
// performs b >> k and stores the result in b | |
void shift_big_integer_right(big_integer *b, unsigned k){ | |
} | |
// performs b1+b2 and stores the result in sum | |
// returns 1 if overflow occurs, 0 otherwise | |
int add_big_integers(big_integer b1, big_integer b2, big_integer *sum){ | |
return 0; | |
} | |
// prints the message and value followed by the program usage message | |
void print_usage(const char *message, const char* value){ | |
printf("%s %s\n", message, value); | |
} |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
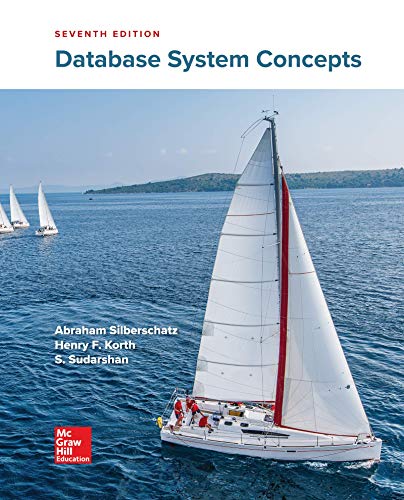
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
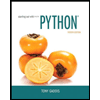
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
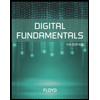
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
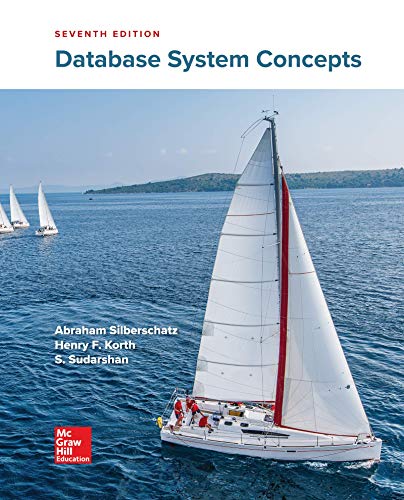
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
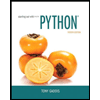
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
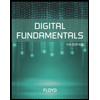
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
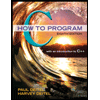
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
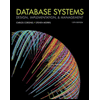
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
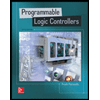
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education