This is the C code I have so far #include #include struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]); printf(" %d", e->yearBorn); printf("\n$%d.", e->salary); } // function to free the memory of the employee pointer void releaseEmployee(struct employees *e) { free(e); } // main method int main() { // creating the employee struct employees *emp = createEmployee(); // printing the information display(emp); // free the memory releaseEmployee(emp); return0; }
This is the C code I have so far
#include <stdio.h>
#include <stdlib.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
struct employees **emps = new employees()[10]; //Added new statement ---- bartleby
// function to read the employee data from the user
void readEmployee(struct employees *emp)
{
printf("Enter name: ");
gets(emp->name);
printf("Enter ssn: ");
for(int i =0; i <9; i++)
scanf("%d", &emp->ssn[i]);
printf("Enter birth year: ");
scanf("%d", &emp->yearBorn);
printf("Enter salary: ");
scanf("%d", &emp->salary);
}
// function to create a pointer of employee type
struct employees *createEmployee()
{
// creating the pointer
struct employees *emp = malloc(sizeof(struct employees));
// function to read the data
readEmployee(emp);
// returning the data
return emp;
}
// function to print the employee data to console
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
// function to free the memory of the employee pointer
void releaseEmployee(struct employees *e)
{
free(e);
}
// main method
int main()
{
// creating the employee
struct employees *emp = createEmployee();
// printing the information
display(emp);
// free the memory
releaseEmployee(emp);
return0;
}


Step by step
Solved in 4 steps with 4 images

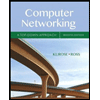
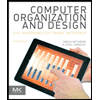
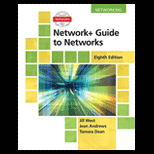
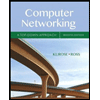
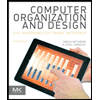
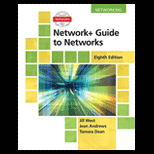
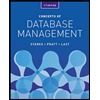
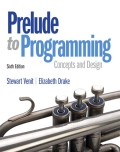
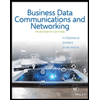