transfer this c++ code to python code C++ CODE #include using namespace std; class LinkNode { public: int data; LinkNode *next; }; void insert(LinkNode** header, int data) { LinkNode* node = new LinkNode(); node->data = data; node->next = *header; *header = node; } void add(LinkNode** header, int data) { LinkNode* node = new LinkNode(); LinkNode *last = *header; node->data = data; node->next = NULL; if(*header== NULL) { *header=node; return; } while(last->next != NULL) { last = last->next; } last->next=node; return; } LinkNode* removeNode(struct LinkNode* head) { if(head == NULL) return NULL; if (head->next == NULL) { delete head; return NULL; } LinkNode* second_last = head; while(second_last->next->next != NULL) second_last = second_last->next; delete (second_last->next); second_last->next = NULL; return head; } void printList(LinkNode *node) { while (node != NULL) { cout<<" "<data; node = node->next; } } int main() { LinkNode* header = NULL; add(&header, 11); insert(&header, 22); insert(&header, 33); insert(&header, 44); insert(&header, 55); cout<<"Created Linked list is: "; printList(header); header = removeNode(header); cout<<"\nLinked list is After deleletion: "; printList(header); return 0; }
transfer this c++ code to python code
C++ CODE
#include <bits/stdc++.h>
using namespace std;
class LinkNode
{
public:
int data;
LinkNode *next;
};
void insert(LinkNode** header, int data)
{
LinkNode* node = new LinkNode();
node->data = data;
node->next = *header;
*header = node;
}
void add(LinkNode** header, int data)
{
LinkNode* node = new LinkNode();
LinkNode *last = *header;
node->data = data;
node->next = NULL;
if(*header== NULL)
{
*header=node;
return;
}
while(last->next != NULL)
{
last = last->next;
}
last->next=node;
return;
}
LinkNode* removeNode(struct LinkNode* head)
{
if(head == NULL)
return NULL;
if (head->next == NULL)
{
delete head;
return NULL;
}
LinkNode* second_last = head;
while(second_last->next->next != NULL)
second_last = second_last->next;
delete (second_last->next);
second_last->next = NULL;
return head;
}
void printList(LinkNode *node)
{
while (node != NULL)
{
cout<<" "<<node->data;
node = node->next;
}
}
int main()
{
LinkNode* header = NULL;
add(&header, 11);
insert(&header, 22);
insert(&header, 33);
insert(&header, 44);
insert(&header, 55);
cout<<"Created Linked list is: ";
printList(header);
header = removeNode(header);
cout<<"\nLinked list is After deleletion: ";
printList(header);
return 0;
}

Step by step
Solved in 4 steps with 4 images

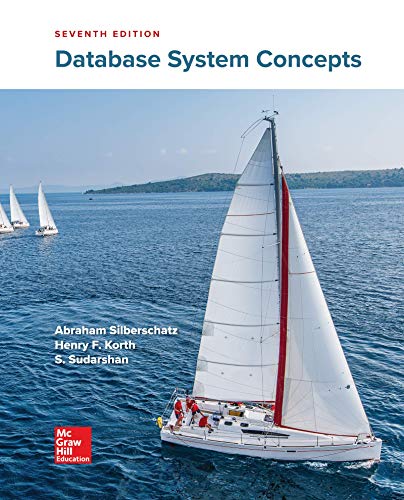
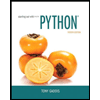
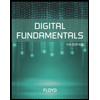
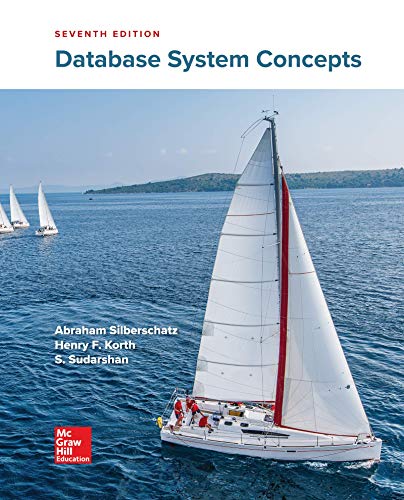
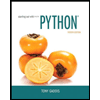
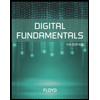
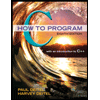
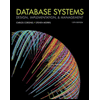
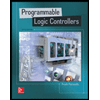