Consider the pop() function in lab4c.c. It is correctly written to take the stack parameter as a pointer. Also note that this pop( ) function only removes the top value from the stack by decrementing the inUse value. It does NOT remove the top value AND also return the top value as is often normally done in a pop( ) function. (Writing this function to remove and return the top value would add way too much complexity to this question. So this code was given using a simpler algorithm/variation of pop.)
given code lab4
#include <stdio.h>
#include <stdlib.h>
/* typical C boolean set-up */
#define TRUE 1
#define FALSE 0
typedef struct StackStruct
{
int* darr; /* pointer to dynamic array */
int size; /* amount of space allocated */
int inUse; /* top of stack indicator
- counts how many values are on the stack */
} Stack;
void init (Stack* s)
{
s->size = 2;
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
void push (Stack* s, int val)
{
/* QUESTION 7 */
/* check if enough space currently on stack and grow if needed */
/* add val onto stack */
s->darr[s->inUse] = val;
s->inUse = s->inUse + 1;
}
int isEmpty (Stack* s)
{
if ( s->inUse == 0)
return TRUE;
else
return FALSE;
}
int top (Stack* s)
{
return ( s->darr[s->inUse-1] );
}
/* QUESTION 9.1 */
void pop (Stack* s)
{
if (isEmpty(s) == FALSE)
s->inUse = s->inUse - 1;
}
void reset (Stack* s)
{
/* Question 10: how to make the stack empty? */
}
int main (int argc, char** argv)
{
Stack st1;
init (&st1);
push (&st1, 20);
push (&st1, 30);
push (&st1, 40);
push (&st1, 50);
while ( isEmpty(&st1) == FALSE)
{
int value = top (&st1);
printf ("The top value on the stack is %d\n", value);
/* QUESTION 9.2 */
pop (&st1);
}
printf ("The stack is now empty\n");
}


#include <stdio.h>
#include <stdlib.h>
/* typical C boolean set-up */
#define TRUE 1
#define FALSE 0
typedef struct StackStruct
{
int* darr; /* pointer to dynamic array */
int size; /* amount of space allocated */
int inUse; /* top of stack indicator
- counts how many values are on the stack */
} Stack;
void init (Stack* s)
{
s->size = 2;
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
void push (Stack* s, int val)
{
/* QUESTION 7 */
/* check if enough space currently on stack and grow if needed */
if(s->inUse+1 >= s->size){ //If whole array is inn use i.e inUse +1 = size
s->size=s->size*2; //Increase the size to 2 times the current
//Using realloc to resize the size of array, it automatically retains previous elemnts
s->darr = (int *)realloc(s->darr,(s->size)*sizeof(int));
}
/* add val onto stack */
s->darr[s->inUse] = val;
s->inUse = s->inUse + 1;
}
int isEmpty (Stack* s)
{
if ( s->inUse == 0)
return TRUE;
else
return FALSE;
}
int top (Stack* s)
{
return ( s->darr[s->inUse-1] );
}
/* QUESTION 9 */
void pop (Stack* s)
{
s->inUse = s->inUse - 1;
}
void reset (Stack* s)
{
s->size=2;
free(s->darr);
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
int main (int argc, char** argv)
{
Stack st1;
init (&st1);
push (&st1, 20);
push (&st1, 30);
push (&st1, 40);
push (&st1, 50);
while ( isEmpty(&st1) == FALSE)
{
int value = top (&st1);
printf ("The top value on the stack is %d\n", value);
/* QUESTION 10 */
pop (&st1);
}
printf ("The stack is now empty\n");
}
Step by step
Solved in 2 steps

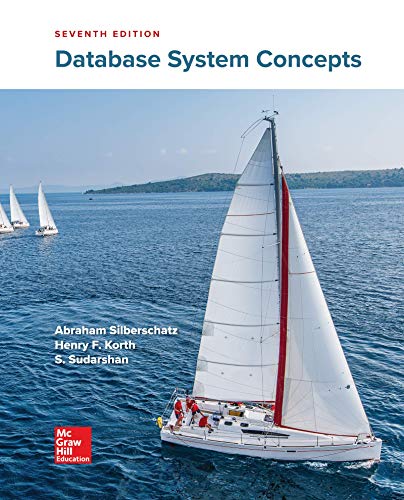
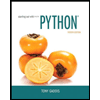
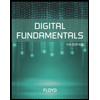
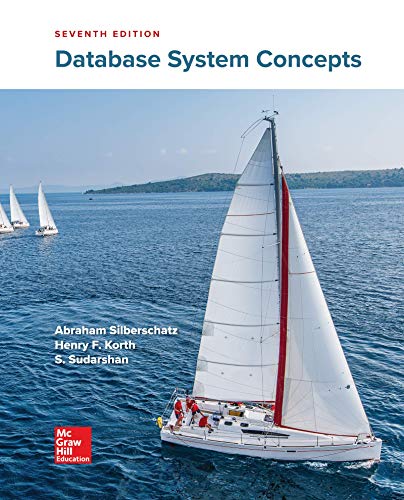
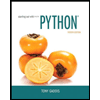
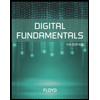
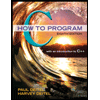
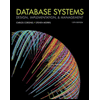
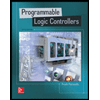