stack.h is in picture this is stack.c #include #include #include #include #include "stack.h" void push(Stack **q, char *word) { // IMPLEMENT } char *pop(Stack *q) { // IMPLEMENT } void print(Stack *q) { // IMPLEMENT } int isEmpty(Stack *q) { // IMPLEMENT } void delete(Stack *q) { // IMPLEMENT } /***** Expected output: ***** No items a b c a b c d e f No items s = World t = Hello *****************************/ int main(int argc, char **argv) { Stack *q = NULL; // print the stack print(q); // push items push(&q, "a"); push(&q, "b"); push(&q, "c"); print(q); // pop items while (!isEmpty(q)) { char *item = pop(q); printf("%s\n", item); free(item); } char *item = pop(q); assert(item == NULL); // push again push(&q, "d"); push(&q, "e"); push(&q, "f"); print(q); // destroy the stack delete(q); // print again print(q); // check copy char *s = (char *)malloc(10); strcpy(s, "Hello"); push(&q, s); strcpy(s, "World"); char *t = pop(q); printf("s = %s\n", s); printf("t = %s\n", t); free(t); free(s); // free the stack free(q); }
stack.h is in picture this is stack.c #include #include #include #include #include "stack.h" void push(Stack **q, char *word) { // IMPLEMENT } char *pop(Stack *q) { // IMPLEMENT } void print(Stack *q) { // IMPLEMENT } int isEmpty(Stack *q) { // IMPLEMENT } void delete(Stack *q) { // IMPLEMENT } /***** Expected output: ***** No items a b c a b c d e f No items s = World t = Hello *****************************/ int main(int argc, char **argv) { Stack *q = NULL; // print the stack print(q); // push items push(&q, "a"); push(&q, "b"); push(&q, "c"); print(q); // pop items while (!isEmpty(q)) { char *item = pop(q); printf("%s\n", item); free(item); } char *item = pop(q); assert(item == NULL); // push again push(&q, "d"); push(&q, "e"); push(&q, "f"); print(q); // destroy the stack delete(q); // print again print(q); // check copy char *s = (char *)malloc(10); strcpy(s, "Hello"); push(&q, s); strcpy(s, "World"); char *t = pop(q); printf("s = %s\n", s); printf("t = %s\n", t); free(t); free(s); // free the stack free(q); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
stack.h is in picture
this is stack.c
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <assert.h>
#include "stack.h"
void push(Stack **q, char *word) {
// IMPLEMENT
}
char *pop(Stack *q) {
// IMPLEMENT
}
void print(Stack *q) {
// IMPLEMENT
}
int isEmpty(Stack *q) {
// IMPLEMENT
}
void delete(Stack *q) {
// IMPLEMENT
}
/***** Expected output: *****
No items
a
b
c
a
b
c
d
e
f
No items
s = World
t = Hello
*****************************/
int main(int argc, char **argv)
{
Stack *q = NULL;
// print the stack
print(q);
// push items
push(&q, "a");
push(&q, "b");
push(&q, "c");
print(q);
// pop items
while (!isEmpty(q)) {
char *item = pop(q);
printf("%s\n", item);
free(item);
}
char *item = pop(q);
assert(item == NULL);
// push again
push(&q, "d");
push(&q, "e");
push(&q, "f");
print(q);
// destroy the stack
delete(q);
// print again
print(q);
// check copy
char *s = (char *)malloc(10);
strcpy(s, "Hello");
push(&q, s);
strcpy(s, "World");
char *t = pop(q);
printf("s = %s\n", s);
printf("t = %s\n", t);
free(t);
free(s);
// free the stack
free(q);
}
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <assert.h>
#include "stack.h"
void push(Stack **q, char *word) {
// IMPLEMENT
}
char *pop(Stack *q) {
// IMPLEMENT
}
void print(Stack *q) {
// IMPLEMENT
}
int isEmpty(Stack *q) {
// IMPLEMENT
}
void delete(Stack *q) {
// IMPLEMENT
}
/***** Expected output: *****
No items
a
b
c
a
b
c
d
e
f
No items
s = World
t = Hello
*****************************/
int main(int argc, char **argv)
{
Stack *q = NULL;
// print the stack
print(q);
// push items
push(&q, "a");
push(&q, "b");
push(&q, "c");
print(q);
// pop items
while (!isEmpty(q)) {
char *item = pop(q);
printf("%s\n", item);
free(item);
}
char *item = pop(q);
assert(item == NULL);
// push again
push(&q, "d");
push(&q, "e");
push(&q, "f");
print(q);
// destroy the stack
delete(q);
// print again
print(q);
// check copy
char *s = (char *)malloc(10);
strcpy(s, "Hello");
push(&q, s);
strcpy(s, "World");
char *t = pop(q);
printf("s = %s\n", s);
printf("t = %s\n", t);
free(t);
free(s);
// free the stack
free(q);
}

Transcribed Image Text:/* Do not modify */
#ifndef CQUEUE_H
#define CQUEUE_H
struct node_struct {
char *data;
struct node_struct *next;
};
typedef struct node_struct Node;
struct stack_struct {
Node *head, *tail;
};
typedef struct stack_struct Stack;
/* Push a word to the top of this stack
* You must keep a *COPY* of the word.
* If q is NULL, allocate space for it here
*/
void push (Stack **q, char *word);
/* Returns the data at the top of the stack
* and remove it from the stack as well.
* If q is empty, return NULL
char *pop (Stack *q);
/* Checks if the stack is empty */
int isEmpty (Stack *q);
/* Prints out the current stack, front to back.
* If the stack is empty, prints out "No items"
*/
void print (Stack *q);
/* Deallocates all items in the stack */
void delete (Stack *q);
#endif

Transcribed Image Text:Stack (or a LIFO) is another basic data structure that is very common in low-level system implementa-
tions i.e. system libraries and OS kernels. You will work on a simple implementation of a dynamic stack
where each element is a string. The underlying implementation of your stack will be similar to a linked
list.
Your Task: Complete the implement of the following functions in stack. c,
• void push (Stack **q, char *word) - Pushes a string word to the top of the stack q. Instead
of keeping the pointer to the array, you must instead keep a COPY of the word inside the stack.
Also, if q hasn't been allocated i.e. q is NULL, you must allocate space for it here as well. Also,
please note that the input is a double pointer as the address to your stack can change in the case
q has not been allocated.
• char *pop (Stack *q) - Returns the pointer to the string at the top of the Stack and removes it
from the stack. When q is empty, this function returns NULL.
• int isEmpty (Stack *q) - Return true if the stack is empty and false otherwise.
• void print (Stack *q) - Prints out the elements of a given stack q, top to bottom. If q is empty,
prints out No items.
• void delete (Stack **q) - Deallocates the stack as well as all items in it.
Expected Behavior: After you have finish implementing stack.c, you can check your own work by
running:
> ./stack
and compare the output with the provided expected output in the starter code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
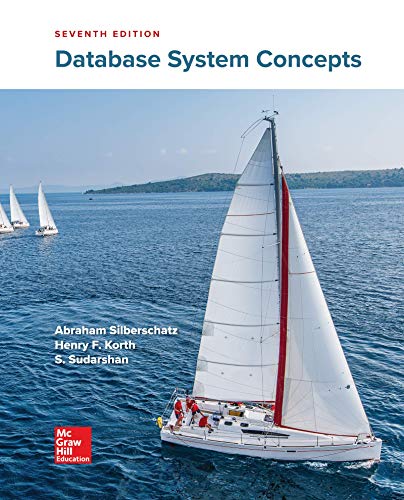
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
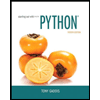
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
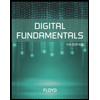
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
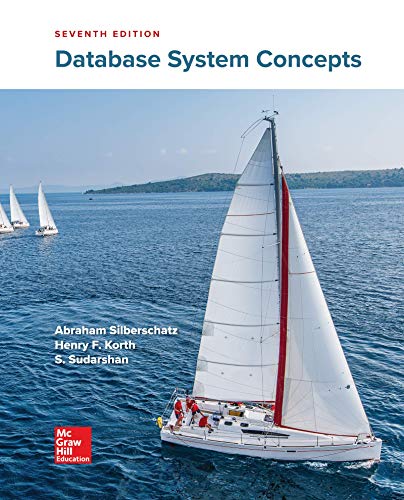
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
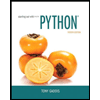
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
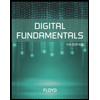
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
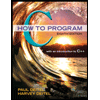
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
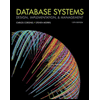
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
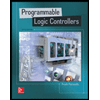
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education