I'm getting a different output. I need to get the expected output Python Code: from Stack import Stack def display_(S_): node = S_.list.head while node != None: print(node.data) node = node.next def Sorting_Stack(num_stack): temp_head1 = num_stack.list.head # sorting the strings in the stack in descending order using bubble sort while(temp_head1!=None): temp_head2 = temp_head1.next # looping through to end and placing the greatest element at the current position while(temp_head2!=None): # if next element of the current is greater then swapping if(temp_head2.data>temp_head1.data): temp_d = temp_head1.data temp_head1.data = temp_head2.data temp_head2.data = temp_d temp_head2 = temp_head2.next temp_head1 = temp_head1.next if __name__ == '__main__': # creatin stack str_stack = Stack() # taking user input till sentinel value is entered inp_str = input() while (inp_str != "End"): str_stack.push(inp_str) inp_str = input() if (str_stack.list.head == None): print("Empty Stack, enter a valid string!") inp_str = input() str_stack.push(inp_str) # sorting and displaying the sorted stack Sorting_Stack(str_stack) display_(str_stack)
I'm getting a different output. I need to get the expected output
Python Code:
from Stack import Stack
def display_(S_):
node = S_.list.head
while node != None:
print(node.data)
node = node.next
def Sorting_Stack(num_stack):
temp_head1 = num_stack.list.head
# sorting the strings in the stack in descending order using bubble sort
while(temp_head1!=None):
temp_head2 = temp_head1.next
# looping through to end and placing the greatest element at the current position
while(temp_head2!=None):
# if next element of the current is greater then swapping
if(temp_head2.data>temp_head1.data):
temp_d = temp_head1.data
temp_head1.data = temp_head2.data
temp_head2.data = temp_d
temp_head2 = temp_head2.next
temp_head1 = temp_head1.next
if __name__ == '__main__':
# creatin stack
str_stack = Stack()
# taking user input till sentinel value is entered
inp_str = input()
while (inp_str != "End"):
str_stack.push(inp_str)
inp_str = input()
if (str_stack.list.head == None):
print("Empty Stack, enter a valid string!")
inp_str = input()
str_stack.push(inp_str)
# sorting and displaying the sorted stack
Sorting_Stack(str_stack)
display_(str_stack)


Step by step
Solved in 4 steps with 2 images

Need to fix code to pass the final output in image
Python code:
from Stack import Stack
def display_(S_):
node = S_.list.head
while node != None:
print(node.data)
node = node.next
def Sorting_Stack(num_stack):
temp_head1 = num_stack.list.head
# sorting the strings in the stack in descending order using bubble sort
while(temp_head1!=None):
temp_head2 = temp_head1.next
# looping through to end and placing the greatest element at the current position
while(temp_head2!=None):
# if next element of the current is greater then swapping
if(temp_head2.data>temp_head1.data):
temp_d = temp_head1.data
temp_head1.data = temp_head2.data
temp_head2.data = temp_d
temp_head2 = temp_head2.next
temp_head1 = temp_head1.next
if __name__ == '__main__':
# creatin stack
str_stack = Stack()
# taking user input till sentinel value is entered
inp_str = input()
while (inp_str != "End"):
str_stack.push(inp_str)
inp_str = input()
if (str_stack.list.head == None):
print("Empty Stack, enter a valid string!")
inp_str = input()
str_stack.push(inp_str)
# sorting and displaying the sorted stack
Sorting_Stack(str_stack)
display_(str_stack)
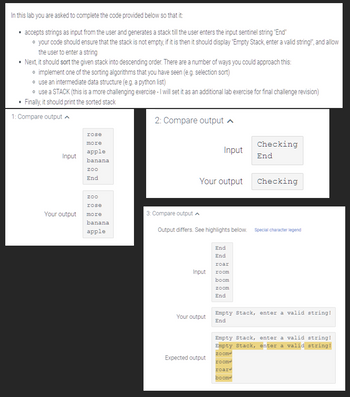
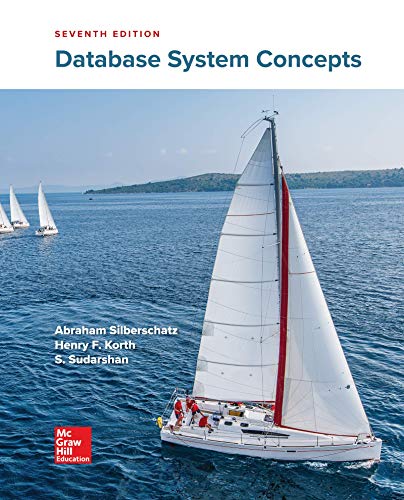
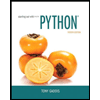
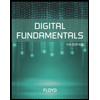
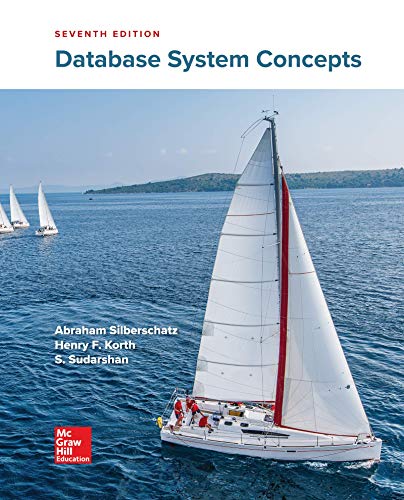
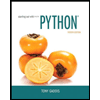
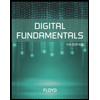
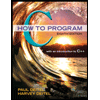
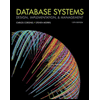
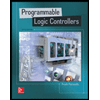