title self.director = director self.producer = producer self.release_date = release_date self.duration = duration self.dvd_id = dvd_id def get_title(self): return self.title def get_director(self): return self.director def get_producer(self): return self.producer
class dvd:
def __init__(self, title, director, producer, release_date, duration, dvd_id):
self.title = title
self.director = director
self.producer = producer
self.release_date = release_date
self.duration = duration
self.dvd_id = dvd_id
def get_title(self):
return self.title
def get_director(self):
return self.director
def get_producer(self):
return self.producer
def get_release_date(self):
return self.release_date
def get_duration(self):
return self.duration
def get_dvd_id(self):
return self.dvd_id
def __repr__(self):
return "Movie Name: "+self.title+", \nDirector : "+self.director+", \nProducer : "+self.producer+", \nRelease Date : "+str(self.release_date)+", \nDuration : "+str(self.duration)+", \nDVD ID : "+str(self.dvd_id)
class customer:
def __init__(self, name, customer_id):
self.name = name
self.dvds=[]
self.customer_id = customer_id
def get_name(self):
return self.name
def get_customer_id(self):
return self.customer_id
def getDvds(self):
s=""
for d in self.dvds:
s=s+d.__repr__()+"\n"
return s
def __repr__(self):
return "Customer Name : "+self.name+"\nCustomer ID : "+str(self.customer_id)+"\n Rented DVD Details : "+self.getDvds()
class store:
def __init__(self):
self.dvds = []
self.customers = []
def add_dvd(self, dvd):
self.dvds.append(dvd)
def add_customer(self, customer):
self.customers.append(customer)
def rent_dvd(self, dvd_id, customer_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
for customer in self.customers:
if customer.get_customer_id() == customer_id:
customer.dvds.append(dvd)
self.dvds.remove(dvd)
def return_dvd(self, dvd_id, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
for dvd in customer.dvds:
if dvd.get_dvd_id() == dvd_id:
self.dvds.append(dvd)
customer.dvds.remove(dvd)
def get_dvd(self, dvd_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
return dvd
def get_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer
def get_dvds(self):
return self.dvds
def get_customers(self):
return self.customers
def get_dvds_for_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer.dvds
# main
store = store()
dvd1 = dvd("Iron Man", "Jon Favreau", "Avi Arad/Kevin Feige", 2008, 156, 1)
dvd2 = dvd("The Incredible Hulk", "Louis Leterrier", "Avi Arad/Gale Anne Hurd/Kevin Feige", 2008, 91, 2)
dvd3 = dvd("Iron Man 2", "Jon Favreau", "Kevin Feige", 2010, 150, 3)
dvd4 = dvd("Thor", "Kenneth Branagh", "Kevin Feige", 2011, 144, 4)
dvd5 = dvd("Captain America: The First Avenger", "Joe Johnston", "Kevin Feige", 2011, 144, 5)
dvd6 = dvd("The Avengers", "Joss Whedon", "Kevin Feige", 2012, 133, 6)
dvd7 = dvd("Iron Man 3", "Shane Black", "Kevin Feige", 2013, 126, 7)
dvd8 = dvd("Thor: The Dark World ", "Kenneth Branagh", "Kevin Feige", 2013, 114, 8)
dvd9 = dvd("Guardians of the Galaxy", "James Gunn", "Kevin Feige", 2014, 120, 9)
dvd10 = dvd("Avengers: Age of Ultron", "Joss Whedon", "Kevin Feige", 2015, 132, 10)
store.add_dvd(dvd1)
store.add_dvd(dvd2)
store.add_dvd(dvd3)
store.add_dvd(dvd4)
store.add_dvd(dvd5)
store.add_dvd(dvd6)
store.add_dvd(dvd7)
store.add_dvd(dvd8)
store.add_dvd(dvd9)
store.add_dvd(dvd10)
customer1 = customer("Willim Harvay", 1)
customer2 = customer("Robort Root", 2)
customer3 = customer("Romina Daniel", 3)
customer4 = customer("Ravi Chaudri", 4)
customer5 = customer("Elsa Gorgeo", 5)
customer6 = customer("John Trimander", 6)
customer7 = customer("Srabell Carnico", 7)
customer8 = customer("Mike samson", 8)
customer9 = customer("Shorlok Williamson", 9)
customer10 = customer("Rocky simon", 10)
store.add_customer(customer1)
store.add_customer(customer2)
store.add_customer(customer3)
store.add_customer(customer4)
store.add_customer(customer5)
store.add_customer(customer6)
store.add_customer(customer7)
store.add_customer(customer8)
store.add_customer(customer9)
store.add_customer(customer10)
store.rent_dvd(1, 1)
store.rent_dvd(2, 1)
store.rent_dvd(3, 2)
store.rent_dvd(3, 3)
store.return_dvd(2, 1)
store.rent_dvd(1, 1)
store.rent_dvd(2, 1)
store.rent_dvd(3, 2)
store.rent_dvd(3, 3)
store.return_dvd(2, 1)
Create the management method for the following classes,
a)Admin mode:
- See the Available List of DVD
- See the rent-out DVD list
- Add DVD
- Add Customer
- Remove DVD
- Remove Customer
b)Customer mode:
- See the Available List of DVD
- Rent a DVD
- Return a DVD

Step by step
Solved in 2 steps

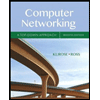
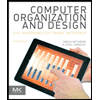
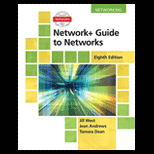
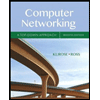
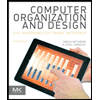
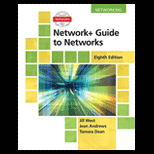
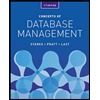
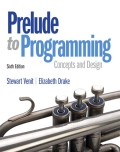
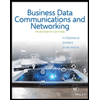